19th Sep 2022
Increase The Performance Of A Static Website With Gulp
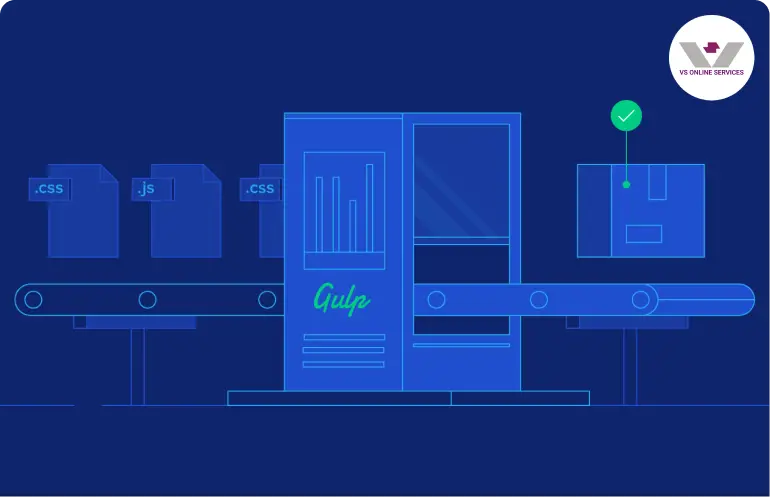
When dealing with a static website which has several css and js files, it is necessary to think about minifying those js and css files, and combining those into separate single files respectively, to speed up loading of the website.
Also there is another issue with caching. When You release new version, browser won't load new files, until You go into developer tools, and disable caching. This can be overcome by changing the query string manually for our css, js files as follows in your index.html

This can also be automated using gulp
Demo project
I created a demo project, where Gulp is used to minify and do Cache Busting for release version of site in public folder
This project contains CSS files in ‘css directory’ and JavaScript files in ‘js’ Directory. Other than that we have fonts and images
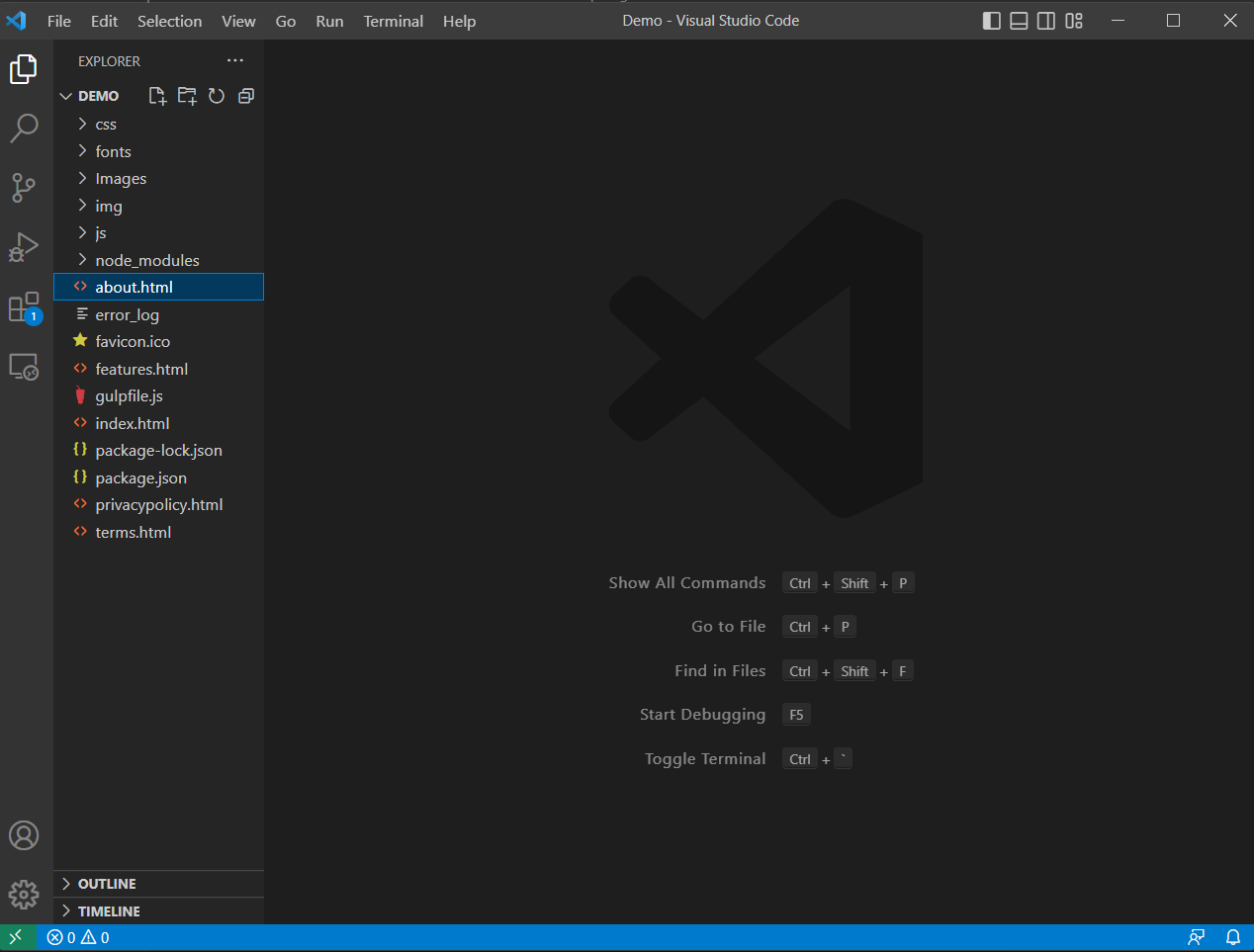
Install dependencies
For minifying and Cache Busting we are going to use Gulp.js
We also need node installed to run the package
Install Gulp
Installing Gulp CLI. Gulp-cli is required to run glupfile.js file
npm install --global gulp-cli
Now let’s CD into our project root, and initialize Node dependency file with
npm init
Now you have created package.json file, which contains list of dependencies
Install Gulp modules
npm install --save-dev gulp gulp-if gulp-useref gulp-uglify gulp-clean-css del gulp-cache-bust gulp-string-replace
Then I Created a gulpfile.js file in the project root directory which is where the automation tasks are defined.
import gulp from 'gulp';
import gulpif from 'gulp-if';
import useref from 'gulp-useref';
import uglify from 'gulp-uglify';
import minifyCss from 'gulp-clean-css';
import {deleteAsync} from 'del';
import cachebust from 'gulp-cache-bust';
import replace from 'gulp-string-replace';
import ngAnnotate from 'gulp-ng-annotate';
gulp.task('delete_all', function() {
return deleteAsync([
'public/*.*',
'public/css/*.*',
'public/js/*.*',
'public/Images/*.*',
'public/img/*.*',
'public/fonts/*.*'
]);
});
gulp.task('minify', function(){
return gulp.src('index.html')
.pipe(useref())
.pipe(gulpif('*.js', ngAnnotate()))
.pipe(gulpif('*.js', uglify()))
.pipe(gulpif('*.css', minifyCss()))
.pipe(gulpif('*.css', replace(/url(skins/g, 'url(../Images/skins')))
.pipe(cachebust({
type: 'timestamp'
}))
.pipe(gulp.dest('public'));
});
gulp.task('copy1',async function () {
return gulp.src('Images/**/*')
.pipe(gulp.dest('public/Images'))
});
gulp.task('copy2', function () {
return gulp.src('img/*.*')
.pipe(gulp.dest('public/img'));
});
//add more tasks based on necessity
gulp.task('default', gulp.series('delete_all', 'minify', 'copy1', 'copy2'));
First I need to import the necessary packages
Let’s start defining the automation tasks one by one
The ‘delete_all’ task above is used to delete our previously generated release version of our app if already created
The ‘minify’ task is where we minify and uglify the css and js files respectively and also do ‘cacheBust’ which we mentioned previously and places the resulting files in the ‘public’ directory
In some cases you might have a disorganized file structure, which leads to broken image and font paths which can be solved using ‘gulp-string-replace’ where you can add a regex for the string(url) that you want to be replaced , and your relief string as alternate parameter, which replaces all the circumstances of the string which matches the given regex pattern with your relief string.
Here ‘copy’ tasks is used to copy the remaining files/folders (other than css and js which we have minified above) to the destination folder(public) which will serve as our final build
Final line of the file is where we execute all our task which we defined in the above steps
Changes to project source
Before running Gulp script, we must do some changes in source.
In our index.html file where we have pointed out our css and js file references needs to be modified in the following way.
Css file references should be moved under

And Js file references should be moved under

Everything between those lines will be combined in just one file, release.css and release.js and also will be minified. Those new files will be automatically added as reference by gulp.js
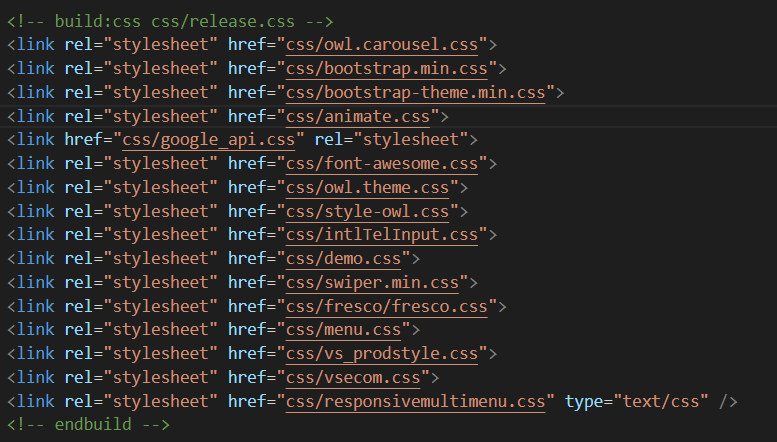
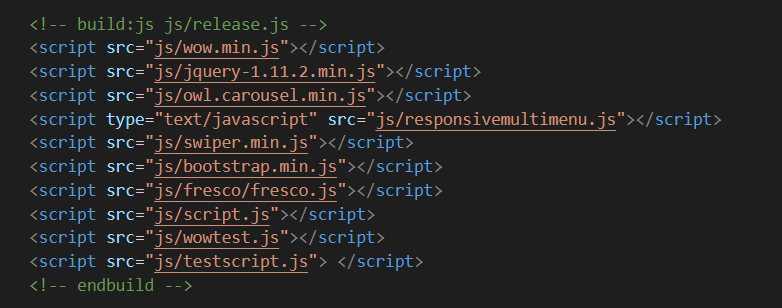
Connect mysql database with nodejs
To create the build version, in the root directory of our project run the command
gulp
This will create the build files in our public directory
[17:23:03] Using gulpfile D:DemoDemogulpfile.js
[17:23:03] Starting 'default'...
[17:23:03] Starting 'delete_all'...
[17:23:03] Finished 'delete_all' after 124 ms
[17:23:03] Starting 'minify'...
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Replaced: "url(skins" to "url(../Images/skins" in a file: D:DemoDemocss
elease.css
[17:23:12] Finished 'minify' after 9.05 s
[17:23:12] Starting 'copy'...
[17:23:13] Finished 'copy' after 70 ms
[17:23:13] Starting 'copy1'...
[17:23:13] Finished 'copy1' after 3.57 ms
[17:23:13] Starting 'copy2'...
[17:23:13] Finished 'copy2' after 97 ms
[17:23:13] Starting 'copy3'...
[17:23:13] Finished 'copy3' after 640 ms
[17:23:13] Starting 'copy4'...
[17:23:13] Finished 'copy4' after 11 ms
[17:23:13] Starting 'copy5'...
[17:23:13] Finished 'copy5' after 22 ms
[17:23:13] Starting 'copy6'...
[17:23:13] Finished 'copy6' after 40 ms
[17:23:13] Starting 'copy7'...
[17:23:13] Finished 'copy7' after 16 ms
[17:23:13] Starting 'copy8'...
[17:23:13] Finished 'copy8' after 21 ms
[17:23:13] Finished 'default' after 10 s
Take a look into generated source in public folder. You will see in index.html, that we have only one js and css file, with time stamp added as query parameter and in release.js you can see that query string is automatically streamlined with the time stamp at the time of running quaff.


Conclusion
Using quaff We created release interpretation of our web point, where all js and css lines are minified and combined into single lines, and where Cache bust is included, to force web browser to load new version.
About Us
- VS Online Services : Custom Software Development
VS Online Services has been providing custom software development for clients across the globe for many years – especially custom ERP, custom CRM, Innovative Real Estate Solution, Trading Solution, Integration Projects, Business Analytics and our own hyperlocal e-commerce platform vBuy.in and vsEcom.
We have worked with multiple customers to offer customized solutions for both technical and no technical companies. We work closely with the stake holders and provide the best possible result with 100% successful completion To learn more about VS Online Services Custom Software Development Solutions or our product vsEcom please visit our SaaS page, Web App Page, Mobile App Page to know about the solution provided. Have an idea or requirement for digital transformation? please write to us at siva@vsonlineservices.com