01st June 2024
Understanding the Differences Between Functional and Class Components in React
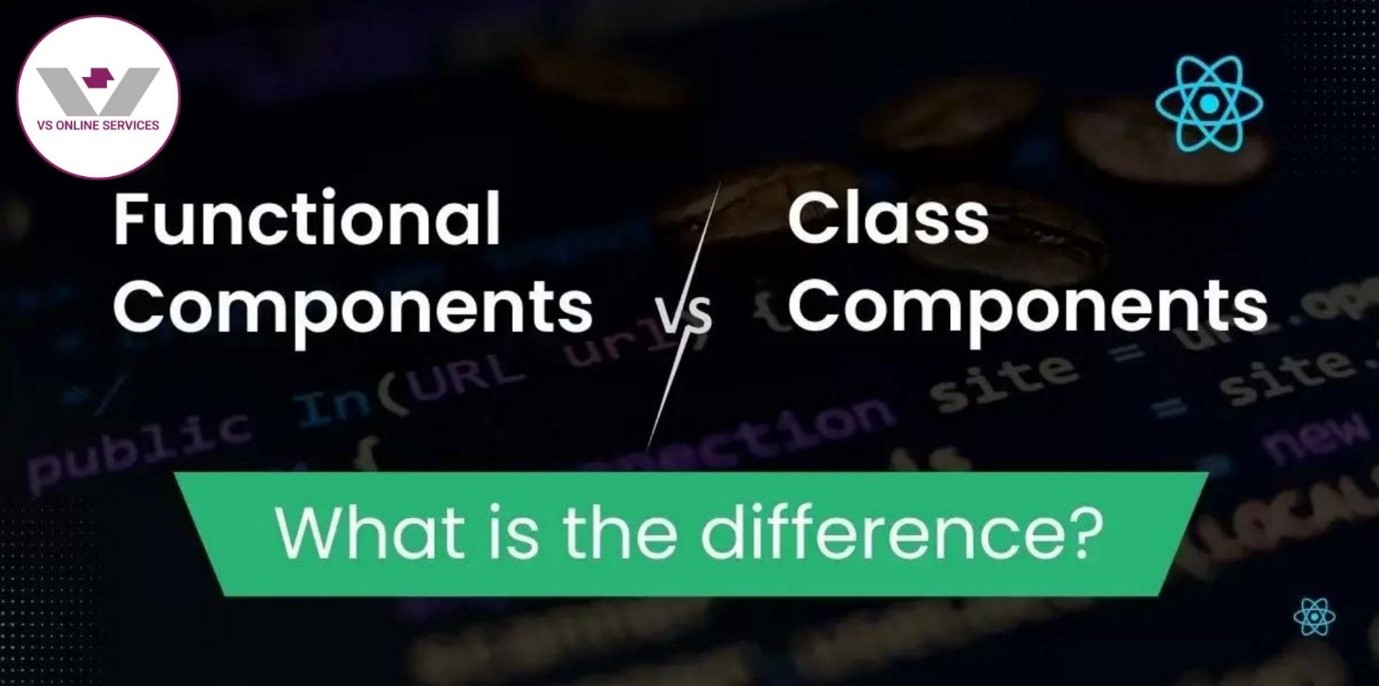
React, a popular JavaScript library for building user interfaces, offers two primary types of components: functional and class components. Both have their unique characteristics and use cases. This article provides an in-depth exploration of the differences between functional and class components, complete with detailed explanations and code examples.
Introduction to React Components
In React, components are the building blocks of the user interface. They encapsulate the structure, behavior, and style of UI elements and can be reused throughout the application. There are two main types of components in React:
- Functional Components: Simple functions that take props as arguments and return JSX.
- Class Components: ES6 classes that extend React.Component and have a render method that returns JSX.
Functional ComponentsDefinition
Functional components are JavaScript functions that accept a single "props" object as an argument and return React elements (JSX). They are often used for simpler components that do not need to manage state or lifecycle methods.
Syntax
The syntax for a functional component is straightforward:
import React from 'react';
const FunctionalComponent = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
};
export default FunctionalComponent;
Example
Here is a simple example of a functional component that displays a greeting message:
import React from 'react';
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
Class Components
Definition
Class components are more feature-rich and can hold and manage their state. They can also use lifecycle methods to perform actions at different stages of the component's life.
Syntax
The syntax for a class component involves extending React.Component and defining a render method:
import React, { Component } from 'react';
class ClassComponent extends Component {
render() {
return (
<div>
<h1>Hello, {this.props.name}!</h1>
</div>
);
}
}
export default ClassComponent;
Example
Here is a simple example of a class component that displays a greeting message:
import React, { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default Greeting;
Differences Between Functional and Class Components
State Management
One of the main differences between functional and class components is how they manage state.
- Functional Components: Initially, functional components were stateless, but with the introduction of Hooks in React 16.8, they can now manage state using the useState hook.
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
- Class Components: Class components manage state by initializing this.state in the constructor and updating it with this.setState.
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Counter;
Lifecycle Methods
- Class Components: Class components have built-in lifecycle methods that allow you to run code at specific points in a component's life (e.g., when it mounts, updates, or unmounts).
import React, { Component } from 'react';
class LifecycleDemo extends Component {
componentDidMount() {
console.log('Component mounted');
}
componentDidUpdate(prevProps, prevState) {
console.log('Component updated');
}
componentWillUnmount() {
console.log('Component will unmount');
}
render() {
return <div>Check the console for lifecycle method logs</div>;
}
}
export default LifecycleDemo;
- Functional Components: Functional components can use the useEffect hook to achieve similar functionality.
import React, { useEffect } from 'react';
const LifecycleDemo = () => {
useEffect(() => {
console.log('Component mounted');
return () => {
console.log('Component will unmount');
};
}, []);
useEffect(() => {
console.log('Component updated');
});
return <div>Check the console for lifecycle method logs</div>;
};
export default LifecycleDemo;
Hooks in Functional Components
Hooks have transformed functional components, allowing them to handle state and lifecycle events.
- useState: Manages state in functional components.
const [state, setState] = useState(initialState);
- useEffect: Performs side effects and replaces lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount.
useEffect(() => {
// Code to run on mount
return () => {
// Cleanup code to run on unmount
};
}, [dependencies]);
- Custom Hooks: Allows you to extract and reuse stateful logic between components.
import { useState, useEffect } from 'react';
const useCustomHook = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return data;
};
Performance
Functional components are generally easier for the React engine to optimize and may result in better performance, especially when using Hooks.
- Functional Components: Lighter and often faster, especially for simple components.
- Class Components: May introduce more complexity and overhead, especially for components with extensive state and lifecycle management.
Code Readability and Maintenance
Functional components often lead to cleaner and more readable code. With Hooks, they can now handle state and lifecycle methods, making them more versatile and easier to maintain.
- Functional Components: Tend to be more concise and easier to read.
- Class Components: Can become bulky with complex state and lifecycle logic.
When to Use Functional or Class Components
With the introduction of Hooks, functional components can now handle most use cases previously reserved for class components. However, there are scenarios where one might be preferred over the other:
- Functional Components: Use when you need simpler, more readable, and potentially more performant code. Ideal for components that do not require extensive state management or lifecycle methods.
- Class Components: Use if you are working on legacy codebases or if you prefer the class-based syntax and structure. They can still be useful in complex applications where the class-based approach is already established.
Conclusion
Both functional and class components have their place in React development. While functional components are now capable of handling complex state and lifecycle scenarios thanks to Hooks, class components remain a viable option, especially in existing projects. Understanding the differences and strengths of each type can help you make informed decisions when building your React applications.
By leveraging the right component type for your specific use case, you can create more efficient, maintainable, and readable codebases.