16th Feb 2023
Dockerizing a Node.js web app
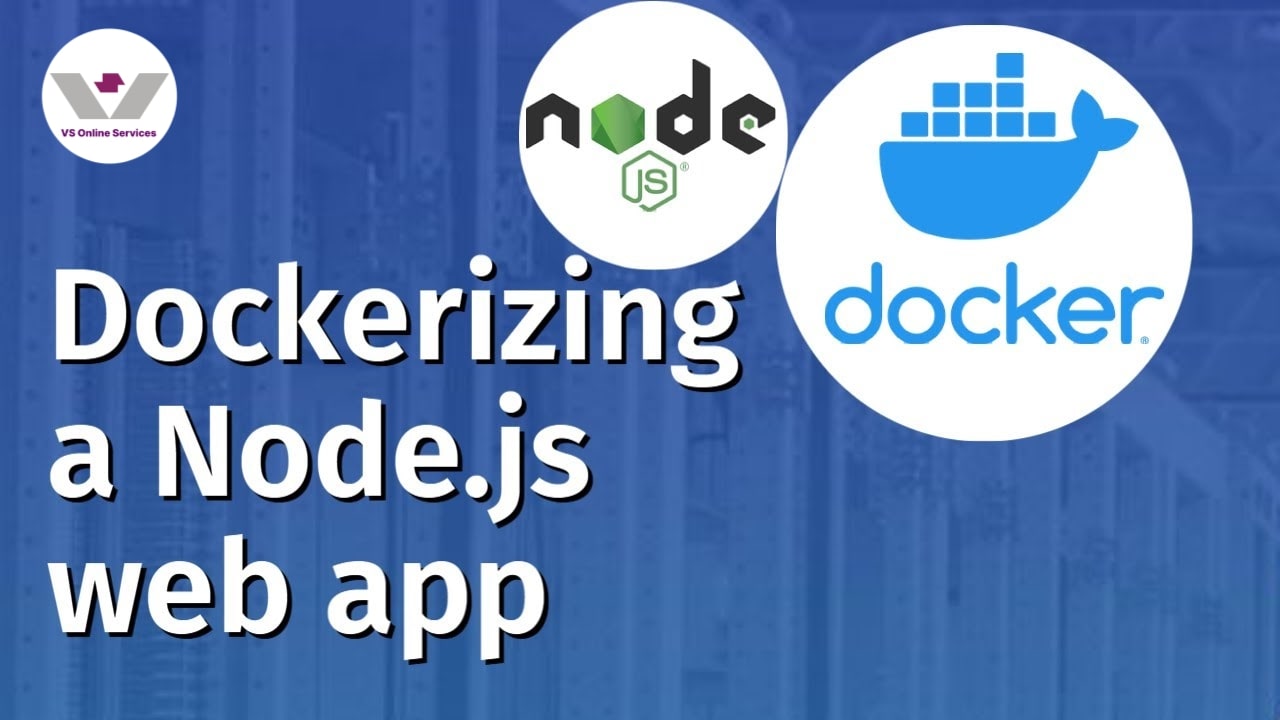
The goal of this example is to show you how to get a Node.js web app into a Docker container. The guide also assumes you have a working Docker installation and a basic understanding of how a Node.js application is structured.
Initially, we will develop a basic web application using Node.js, followed by constructing a Docker image for the application, and finally, we will launch a container based on the created image.
By utilizing Docker, it is possible to encapsulate an application along with its environment and all necessary dependencies into a container, often comprising a trimmed-down variant of a Linux operating system. An image serves as a template for a container, while the container functions as an active instance of the image.
Develop the Node.js application
Initially, generate a new directory to store all the relevant files. Within the directory, create a package.json file that outlines the application and its required dependencies.
{
“name”:”docker_web_app”,
“version”:”1.0.0”,
“description”:Node.js on Docker”,
“author”:”First Last”,
“main”:”server.js”,
“scripts”:{
“start”:”node server.js”
},
“dependencies”:{
“express”:”^14.6.1”
}
}
With your new package.json file, run npm install. If you are using npm version 5 or later, this will generate a package-lock.json file which will be copied to your Docker image. Then, create a server.js file that defines a web app using the Express.js framework:
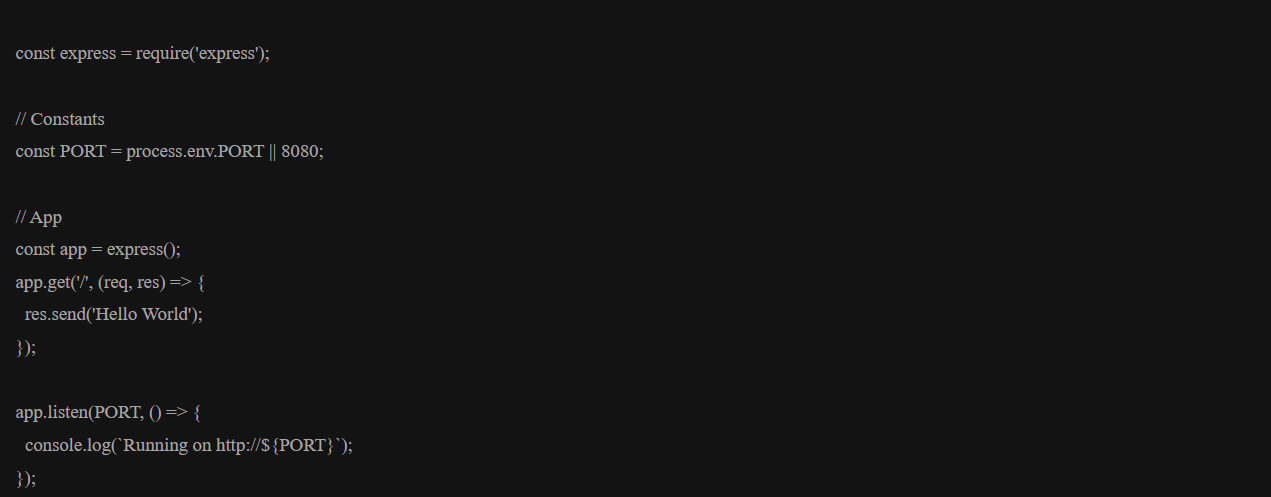
In the next steps, we'll look at how you can run this app inside a Docker container using the official Docker image. First, you'll need to build a Docker image of your app.
Creating a Dockerfile
Create a file called Dockerfile:
touch Dockerfile
Open the Dockerfile in your local IDE
The first thing we need to do is define from what image we want to build from. Here we will use the latest LTS (long term support) version 16 of node available from the
Docker Hub:
FROM node:16
We will now generate a folder within the image specifically designed to store your application's code, which will serve as the designated working directory for your application.
#Create app directory
WORKDIR /usr/src/app
This image comes with Node.js and NPM already installed so the next thing we need to do is to install your app dependencies using the npm. Please note that if you are using npm version 4 or earlier a package-lock.json file will not be generated.Also copy the package.json file
#A wildcard is used to to ensure both package.json AND package-lock.json ARE copied
COPY package.json./
RUN npm install
To bundle your app's source code inside the Docker image, use the COPY instruction:
#Bundle app source
COPY ..
Your app binds to port 8080 so you'll use the EXPOSE instruction to have it mapped by the docker daemon:
EXPOSE 8080
Last but not least, define the command to run your app using CMD which defines your runtime. Here we will use node server.js to start your server:
CMD ["node","server.js"]
Your Dockerfile should now look like this:
FROM node:16
WORKDIR/app
#A wildcard is used to ensure both package.json and package-lock.json are copied
COPY package*.json ./
RUN npm install
COPY ..
ENV PORT=8080
EXPOSE 8080
CMD[“node”, “server.js”]
.dockerignore file
Create a .dockerignore file in the same directory as your Dockerfile with following content:
node_modules
npm-debug.log
This will prevent your local modules and debug logs from being copied onto your Docker image and possibly overwriting modules installed within your image.
Building your image
Go to the directory that has your Dockerfile and run the following command to build the Docker image. The -t flag lets you tag your image so it's easier to find later using the docker images command:
docker build.-t node-web-app
Your image will now be listed by Docker:
docker images
#Example
REPOSITORY TAG ID CREATED
node 16 3b6623445643 5days ago
node-web-app latest d64ddsf5b0d2 1 minute ago
Run the image
Running your image with -d runs the container in detached mode, leaving the container running in the background. The -p flag redirects a public port to a private port inside the container. Run the image you previously built:
docker run -p 8080 -d node-web-app
Test
To test your app, get the port of your app that Docker mapped:
#Example
ID IMAGE COMMAND ... PORTS
ecce33b30ebf node-web-app:latest npm start ... 8080->8080
In the example above, Docker mapped the 8080 port inside of the container to the port 8080 on your machine.
Now you can call your app using curl
curl -i localhost:8080
HTTP/1.1 200 OK
X-powered-By:Express
Content-Type:text/html;charset=utf-8
Content-Length:12
ETag:W/"c-M6tWOb/Y57lesdjQuHeB1P/qTV0"
Date:Mon, 13 Nov 2017 20:53:59 GMT
Connection:keep-alive
Hello World
Shut down the image
In order to shut down the app we started, we run the kill command. This uses the container's ID, which in this example was ecce33b30ebf.
# Kill our running container
docker kill <container_id>
<container_id>
# Confirm that the app has stopped
curl -i localhost:8080
curl:(7) Failed to connect to localhost port 8080:Connection refused
We hope this tutorial helped you get up and running a simple Node.js application on Docker.
Conclusion
Thus, We hope this tutorial helped you to get up and running a simple Node.js application on Docker.
About Us
- VS Online Services : Custom Software Development
VS Online Services has been providing custom software development for clients across the globe for many years - especially custom ERP, custom CRM, Innovative Real Estate Solution, Trading Solution, Integration Projects, Business Analytics and our own hyperlocal e-commerce platform vBuy.in and vsEcom.
We have worked with multiple customers to offer customized solutions for both technical and no technical companies. We work closely with the stake holders and provide the best possible result with 100% successful completion To learn more about VS Online Services Custom Software Development Solutions or our product vsEcom please visit our SaaS page, Web App Page, Mobile App Page to know about the solution provided. Have an idea or requirement for digital transformation? please write to us at siva@vsonlineservices.com