14th June 2024
Understanding .NET Core Garbage Collection and IDisposable
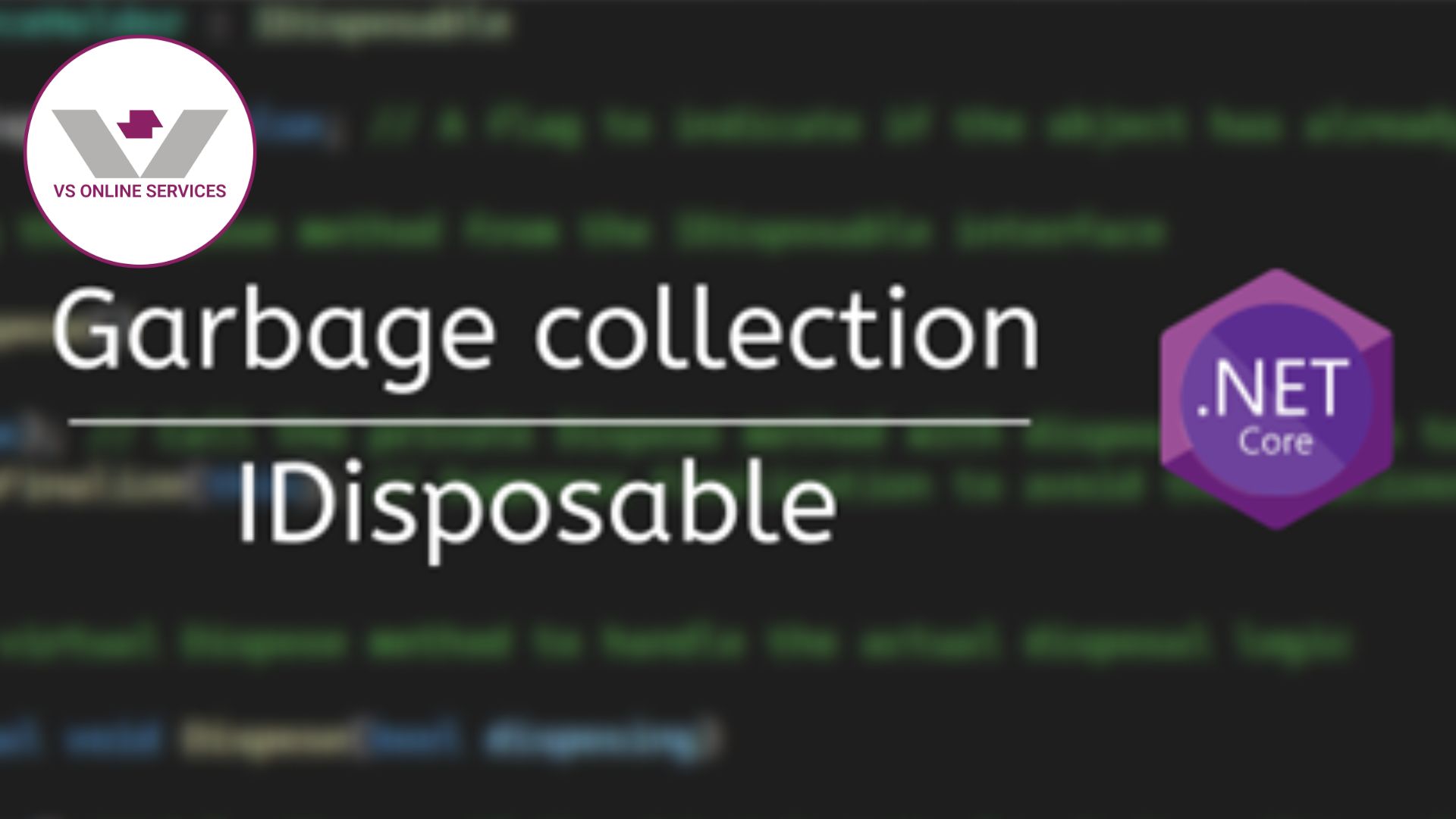
Garbage collection (GC) and the IDisposable interface are fundamental concepts in .NET Core that manage memory and resource usage efficiently. Proper understanding and implementation of these concepts ensure that your applications run smoothly, with minimal memory leaks and resource mismanagement. This blog will delve into the .NET Core garbage collection and the IDisposable pattern, highlighting their importance, workings, and best practices.
Garbage Collection in .NET Core
What is Garbage Collection?
Garbage collection is a form of automatic memory management. The garbage collector attempts to reclaim memory occupied by objects that are no longer in use by the application. The main goal is to free up memory so that it can be reused for other purposes.
- Memory Management: The process of controlling and coordinating computer memory, assigning portions to various running programs to optimize overall system performance.
- Memory Leak: A situation where a program consumes memory but fails to release it back to the system, leading to decreased available memory and potentially causing the application to crash.
The .NET Core Garbage Collector
The .NET Core garbage collector is a sophisticated, optimized system designed to manage memory automatically. It works by:
- Allocating memory for new objects on the managed heap.
- Tracking object references to determine when an object is no longer reachable.
- Reclaiming memory occupied by unreachable objects.
GC Fundamentals
The garbage collector in .NET Core operates on the following principles:
- Generational Hypothesis: This hypothesis states that most objects die young (i.e., they become unreachable soon after allocation), and objects that survive multiple collections are likely to live longer.
- Managed Heap: A region of memory reserved for use by the .NET runtime to allocate managed objects (i.e., objects whose lifetimes are managed by the garbage collector).
Question: What is the size of the managed heap region, and how is it determined?
Answer: The size of the managed heap is dynamic and can change during the execution of a program based on the memory needs of the application and the behavior of the garbage collector. It is initially allocated by the .NET runtime and can grow as needed. Factors influencing its size include system architecture, .NET runtime version, and configuration settings. On 32-bit systems, the addressable memory is typically around 2GB, while on 64-bit systems, it is much larger, limited by physical memory and the operating system's constraints.
- Roots: The starting point for GC is to identify all active roots in the application. Roots can include static fields, local variables, CPU registers, etc.
- References: Pointers or links to objects in memory. If an object is no longer referenced, it's considered unreachable and can be collected by the GC.
Generations and Segments
Generations: The managed heap is divided into generations (Gen 0, Gen 1, and Gen 2), which help optimize the performance of garbage collection. Each generation can have its own segments.
- Generation 0 (Gen 0): The youngest generation, where new objects are allocated.
- Generation 1 (Gen 1): A middle generation that holds objects that have survived one garbage collection cycle in Gen 0.
- Generation 2 (Gen 2): The oldest generation for long-lived objects that have survived multiple garbage collection cycles.
Segments: The managed heap is also divided into segments, which are contiguous regions of memory. There are separate segments for different generations:
- Small Object Heap (SOH): Contains Gen 0, Gen 1, and Gen 2 objects.
- Large Object Heap (LOH): Contains large objects (usually 85,000 bytes or larger) and is collected less frequently than the SOH.
Promotion: The process of moving objects from a lower generation to a higher generation if they survive a garbage collection cycle.
GC Modes
.NET Core supports different GC modes to cater to various application needs:
- Workstation GC: Optimized for desktop and client applications, emphasizing responsiveness.
- Server GC: Designed for server applications, providing high throughput and scalability.
- Concurrent GC: Minimizes application pauses by performing garbage collection concurrently with application threads.
GC Process
The garbage collection process involves several steps:
- Marking: The GC identifies all live objects.
- Sweeping: It reclaims the memory occupied by dead objects.
- Compacting: The GC compacts the heap to reduce fragmentation and improve memory allocation efficiency.
Example Scenario
Imagine you are writing a program that manages a list of customers. The program creates and uses several customer objects during its execution. Some of these objects are no longer needed as the program runs, and the garbage collector needs to reclaim the memory they occupy.
1. Marking
Marking is the first phase where the garbage collector identifies all live objects (objects that are still referenced and needed by the application).
Example:- Suppose your program has created four customer objects: Customer1, Customer2, Customer3, and Customer4.
- Customer1 and Customer2 are still in use (referenced), but Customer3 and Customer4 are no longer referenced anywhere in the code.
- During the marking phase, the garbage collector will traverse all object references starting from root references (like global variables, active method variables, etc.) and mark Customer1 and Customer2 as live objects.
2. Sweeping
Sweeping is the next phase where the garbage collector reclaims memory occupied by dead objects (objects that are no longer needed and not marked as live).
Example:- After the marking phase, the garbage collector knows that Customer3 and Customer4 are dead (no longer referenced).
- During the sweeping phase, the garbage collector reclaims the memory occupied by Customer3 and Customer4, making it available for new objects.
3. Compacting
Compacting is the final phase where the garbage collector compacts the heap to reduce fragmentation and improve memory allocation efficiency.
Example:- Imagine the memory layout before compaction looks like this: [Customer1, Customer2, (free space), (free space)].
- During the compacting phase, the garbage collector moves Customer1 and Customer2 to a contiguous block of memory to eliminate fragmentation.
- The memory layout after compaction might look like this: [Customer1, Customer2, (free space)].
GC Configuration
You can configure the garbage collector using environment variables and runtime configuration settings to optimize performance and resource usage according to your application's needs:
Environment Variables: These variables can be set to influence the behavior of the garbage collector.
- COMPlus_gcConcurrent: Enables or disables concurrent garbage collection.
- COMPlus_gcServer: Configures the garbage collector to use server mode, which is optimized for high-throughput applications.
- GCHeapHardLimit: Sets a maximum size for the managed heap, preventing the heap from growing beyond a specified limit.
- COMPlus_gcHeapCount: Controls the number of heaps (segments) on multi-processor systems, allowing the GC to scale with the number of processors.
App Configuration: Settings can be adjusted in configuration files associated with your .NET Core application.
- runtimeconfig.json: A file where you can specify runtime configuration settings.
This file is placed in your projects bin folder.
{
"runtimeOptions": {
"tfm": "net6.0",
"frameworks": [
{
"name": "Microsoft.NETCore.App",
"version": "6.0.0"
},
{
"name": "Microsoft.AspNetCore.App",
"version": "6.0.0"
}
],
"configProperties": {
"System.GC.Server": true,
"System.Runtime.Serialization.EnableUnsafeBinaryFormatterSerialization": false,
"System.GC.HeapHardLimit": 2147483648, // 2GB limit for the heap
"System.GC.HeapCount": 4 // Number of heaps for a multi-processor system
}
}
}
- csproj: The project configuration file where you can set properties that affect the build and runtime behavior of your application.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<RuntimeIdentifier>win-x64</RuntimeIdentifier>
<COMPlus_gcConcurrent>true</COMPlus_gcConcurrent>
<COMPlus_gcServer>true</COMPlus_gcServer>
<COMPlus_gcHeapHardLimit>2147483648</COMPlus_gcHeapHardLimit> <!-- 2GB limit for the heap -->
<COMPlus_gcHeapCount>4</COMPlus_gcHeapCount> <!-- Number of heaps for a multi-processor system -->
</PropertyGroup>
</Project>
Monitoring GC
Monitoring garbage collection can help you understand your application's memory usage patterns. Tools like Visual Studio Diagnostic Tools, PerfView, and dotnet-counters can provide insights into GC activity.
- Visual Studio Diagnostic Tools: A set of tools in Visual Studio that helps developers diagnose and analyze the performance and behavior of their applications.
- PerfView: A performance analysis tool that provides detailed information on CPU usage, memory allocation, and garbage collection events.
- dotnet-counters: A tool that collects and displays performance counter data for .NET Core applications in real-time.
The IDisposable Interface
What is IDisposable?
The IDisposable interface provides a mechanism for releasing unmanaged resources. It defines a single method, Dispose(), which is called to explicitly release resources.
New Terms:- IDisposable Interface: An interface in .NET that provides a standardized way to release unmanaged resources.
- Unmanaged Resources: Resources that are not handled by the .NET garbage collector, such as file handles, database connections, and network sockets.
Implementing IDisposable
To implement IDisposable, a class needs to:
- Implement the IDisposable interface.
- Provide the Dispose() method to release unmanaged resources.
- Suppress finalization if Dispose() has been called to prevent the garbage collector from calling the finalizer .
public class ResourceHolder : IDisposable
{
private bool disposed = false; // A flag to indicate if the object has already been disposed
// Implementing the Dispose method from the IDisposable interface
public void Dispose()
{
Dispose(true); // Call the private Dispose method with disposing set to true
GC.SuppressFinalize(this); // Suppress finalization to avoid the finalizer being called
}
// A protected virtual Dispose method to handle the actual disposal logic
protected virtual void Dispose(bool disposing)
{
if (!disposed) // Only dispose if the object hasn't already been disposed
{
if (disposing)
{
// Free managed resources here
}
// Free unmanaged resources here
disposed = true; // Mark the object as disposed
}
}
// A finalizer, which is called by the garbage collector
~ResourceHolder()
{
Dispose(false); // Call Dispose with disposing set to false to indicate it's being called by the finalizer
}
}
Finalizer: A method that the garbage collector calls on an object just before it reclaims the object's memory. It's typically used to release unmanaged resources if they haven't been released already.
SuppressFinalization: A method to prevent the finalizer from running if the resources have already been released manually.
Using IDisposable with the using Statement
The using statement provides a convenient syntax for working with IDisposable objects. It ensures that Dispose() is called automatically when the object goes out of scope, even if an exception occurs.
using (var resourceHolder = new ResourceHolder())
{
// Use resourceHolder within this block
// The Dispose method will be called automatically at the end of this block
}
Common Pitfalls and Best Practices
- Not Implementing IDisposable Properly: Ensure that all unmanaged resources are released.
- Forgetting to Call Dispose(): Use the using statement to ensure Dispose() is called automatically.
- Overusing Finalizers: Avoid using finalizers unless necessary, as they add overhead to garbage collection.
Can you use IDisposable without the using statement? How would you ensure resources are properly released?
Answer:Yes, you can use IDisposable without the using statement.
The using statement is a syntactic convenience that ensures Dispose is called automatically, but you can achieve the same functionality without it by explicitly calling Dispose..To ensure resources are properly released, you need to manually call the Dispose method. Here’s how you can do it:
public static void Main(string[] args)
{
ResourceHolder resourceHolder = null;
try
{
resourceHolder = new ResourceHolder();
// Use resourceHolder for various operations
}
finally
{
if (resourceHolder != null)
{
resourceHolder.Dispose(); // Explicitly calling Dispose to release resources
}
}
}
IDisposable and Asynchronous Programming
Implementing IDisposable in asynchronous programming can be challenging. Ensure that resources are correctly managed and released even when operations are performed asynchronously.
Customizing the Garbage Collector
The .NET Core garbage collector can be customized to suit specific application needs by adjusting configuration settings and environment variables.
Performance Considerations
Effective memory management and proper implementation of IDisposable can significantly impact application performance. Monitor and profile your application to identify and resolve memory-related issues.
Conclusion
Understanding .NET Core garbage collection and the IDisposable interface is crucial for developing efficient and reliable applications. By following best practices and leveraging the tools and configurations available, you can ensure optimal memory management and resource utilization in your .NET Core applications.