12th June 2024
Understanding IEnumerable vs IQueryable: A Comprehensive Guide
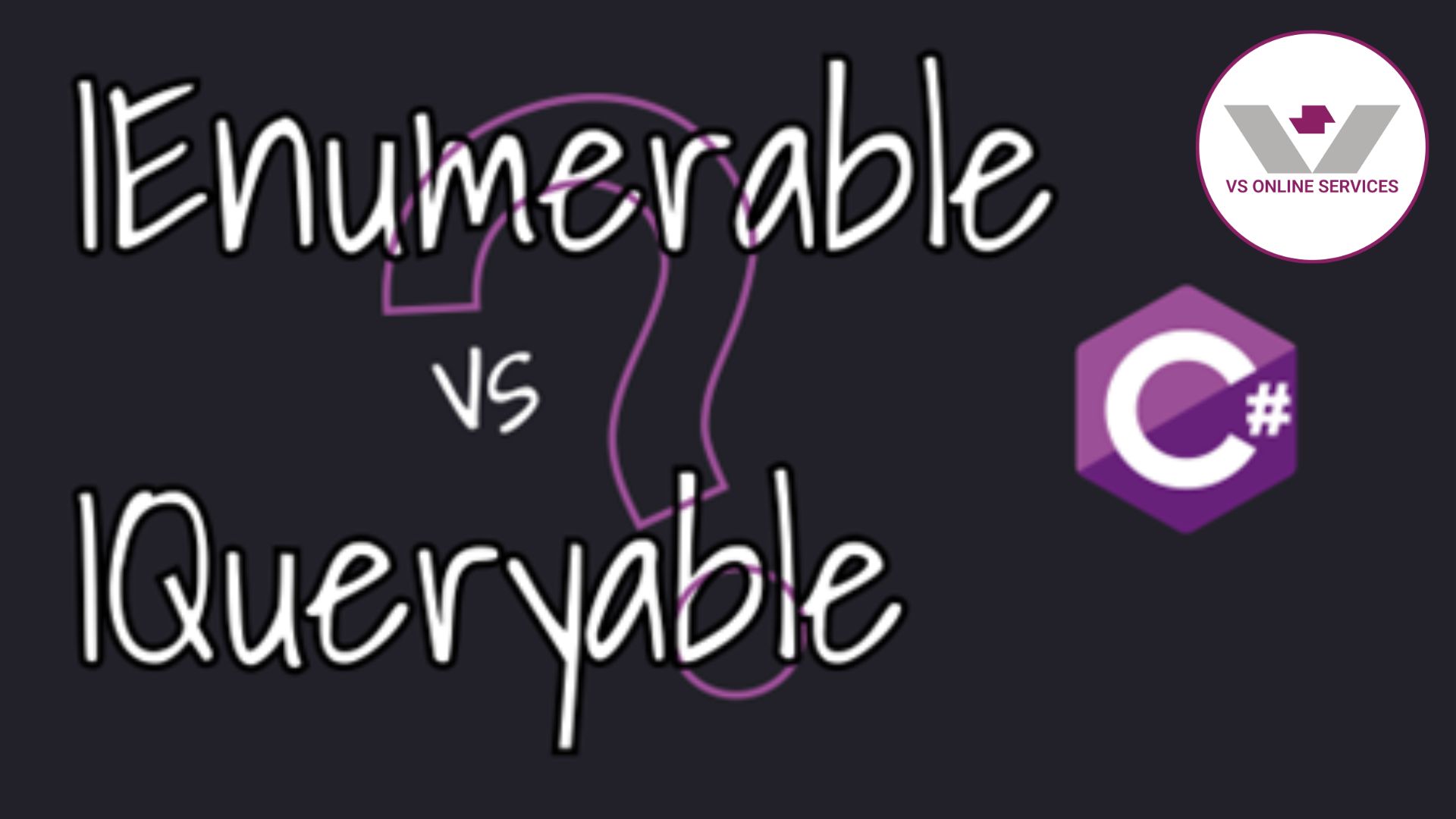
In the .NET ecosystem, collections and data querying are foundational aspects of many applications. Two important interfaces that you often encounter are IEnumerable and IQueryable. Although they may appear similar at first glance, they are designed for different purposes and scenarios. This blog aims to provide a detailed understanding of what these interfaces are, why and how to use them, when to choose one over the other, and common pitfalls to avoid.
What are IEnumerable and IQueryable ?
IEnumerable
- Definition: IEnumerable is an interface defined in the System.Collections.Generic namespace. It represents a forward-only iteration over a collection of a specified type.
What is Forward only iteration?
Forward-only iteration refers to the process of traversing a collection or data set in a single direction, from the first element to the last element, without the ability to go back to previous elements.
- Execution: Deferred execution, meaning that the query is executed when you start iterating over the collection.
- What is Deferred execution: means that the query does not run until you actually try to access the data by iterating over it.
1. Define the query:
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
IEnumerable<int> evenNumbers = numbers.Where(n => n % 2 == 0);
At this point, evenNumbers is just a definition of a query. No data has been processed yet.
2. Execute the query:
The foreach loop starts iterating over evenNumbers, and only now is the Where clause actually applied to filter the numbers list. The query is executed during this iteration.
foreach (var num in evenNumbers)
{
Console.WriteLine(num);
}
- Primary Use Suitable for in-memory collections (e.g., arrays, lists).
IQueryable
- Definition: IQueryable is an interface defined in the System.Linq namespace. It extends IEnumerable and represents a queryable data source.
- Execution: Deferred execution, with the query translated into the native query language of the data source (e.g., SQL for a database).
Deferred execution works similarly, but the query is translated into the native query language of the data source and executed when you iterate or explicitly convert it to a collection (like calling ToList()).
Example:1. Define the query:
IQueryable<Product> query = dbContext.Products.Where(p => p.Price > 100);
At this point, query is just a definition. No database call has been made yet.
2. Execute the query:
foreach (var product in query)
{
Console.WriteLine(product.Name);
}
The foreach loop causes the query to be executed. The LINQ provider translates the Where clause into SQL and executes it on the database, retrieving the filtered results.
- Primary Use Suitable for querying external data sources like databases.
Key Points
- Deferred execution means defining a query doesn't immediately run it.
- The query runs only when you start accessing the results, typically through iteration (foreach) or conversion methods (ToList(), ToArray()).
- This allows for more efficient data handling, as the query is optimized and executed only when necessary.
Why Use IEnumerable and IQueryable?
Why IEnumerable?
- Simplicity: Ideal for simple, straightforward operations on in-memory collections.
- In-Memory Data: When working with data already loaded into memory, IEnumerable is efficient and easy to use.
- Iteration: Designed for forward-only iteration, making it suitable for scenarios where you need to traverse data sequentially.
Why IQueryable?
- Efficient Query Translation: Converts LINQ queries into the data source's native query language, optimizing performance.
- Large Data Sets: Ideal for working with large datasets where querying and filtering need to be performed on the server side to minimize data transfer.
- External Data Sources: Designed to interact with databases and other external data sources.
When to Use IEnumerable vs IQueryable?
Use IQueryable When:
- Querying Databases: Interacting with large datasets stored in databases.
- Optimized Queries: Need for server-side query optimization and execution.
- Minimizing Data Transfer: Reducing the amount of data transferred from the database to the application.
Common Mistakes and Pitfalls
Common Mistakes
1. Mixing IEnumerable and IQueryable:
- Combining these interfaces without understanding their execution contexts can lead to inefficient queries.
2. Forcing Immediate Execution:
- Using methods like ToList() or ToArray() too early can negate the benefits of deferred execution in IQueryable.
Pitfalls
1. Performance Issues:
- Inefficient querying can result from not leveraging the strengths of IQueryable for database operations.
2. Unexpected Results:
- Misunderstanding deferred execution can lead to unexpected results or multiple executions of the same query.
Questions
1. What happens when you call ToList() on an IQueryable?
- The query is executed immediately, and the results are loaded into memory as a list.
2. Can you chain LINQ methods on IQueryable like IEnumerable?
- Yes, but the LINQ methods for IQueryable are in System.Linq.Queryable and are translated into the data source’s query language.
3. How does deferred execution affect performance?
- Deferred execution can improve performance by executing queries only when necessary and optimizing them for the data source.
let's compare IEnumerable and IQueryable in the context of data fetching from a database with an illustrative picture.
IEnumerable
- Fetches All Data First: When using IEnumerable, the entire dataset is fetched from the database and loaded into memory.
- In-Memory Filtering: Any filtering or operations are performed in-memory after fetching the data.
IQueryable
- Query Translated to SQL: When using IQueryable, the query is translated into SQL and executed on the database server.
- Server-Side Filtering: Only the filtered data is fetched from the database and loaded into memory.
Example Scenario: Fetching Products with Price > 100
IEnumerable:
- Fetching Data: The entire Products table is fetched from the database into memory.
- In-Memory Filtering: Filtering happens in-memory, where products with Price > 100 are selected.
List<Product> products = dbContext.Products.ToList(); // Fetches all products into memory
IEnumerable<Product> expensiveProducts = products.Where(p => p.Price > 100); // Filters in memory
IQueryable:
- Query Translation: The query is translated into an SQL query by the Entity Framework.
- Server-Side Filtering: The SQL query with the WHERE clause is executed on the database server, and only the filtered products are fetched.
IQueryable<Product> query = dbContext.Products.Where(p => p.Price > 100); // Query translated to SQL
List<Product> expensiveProducts = query.ToList(); // Fetches only filtered products
Illustration:
Explanation of the Picture:
IEnumerable:
- The database sends the entire Products table to the application.
- The application filters the data in-memory to get products with Price > 100.
IQueryable:
- The application builds a query and sends it to the database.
- The database executes the query (SELECT * FROM Products WHERE Price > 100) and sends only the filtered results back to the application.
Practical Tasks and Projects
Task: Efficiently Querying a Database
- Objective: Use IQueryable to query a large database and retrieve only necessary data.
- Set up an Entity Framework context.
- Create a query using IQueryable.
- Execute the query and analyze the generated SQL.
Project: In-Memory Data Processing
- Objective: Use IEnumerable to process and transform data loaded from a file.
- Query a database using IQueryable.
- Load additional in-memory data.
- Combine the results using LINQ operations.
Conclusion
Understanding the differences between IEnumerable and IQueryable is crucial for writing efficient and effective .NET applications. Each interface serves specific purposes and knowing when and how to use them can significantly impact the performance and scalability of your application. By avoiding common pitfalls and leveraging the strengths of each, you can build robust and optimized solutions.