24th May 2024
Localization and Globalization in .NET Applications: A Comprehensive Guide
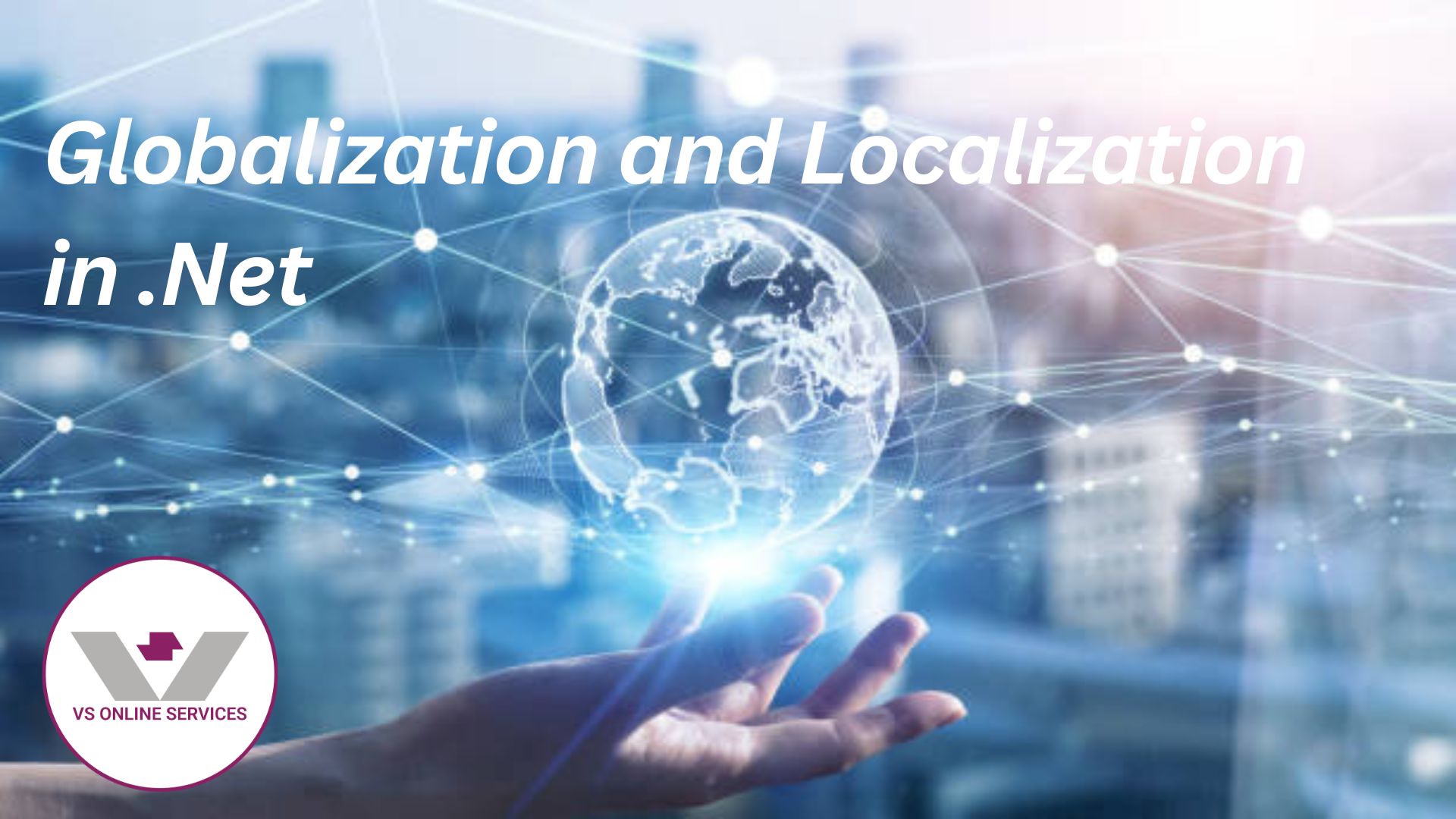
Localization and globalization are essential aspects of modern web applications that aim to provide a personalized experience for users across different cultures and languages. In this blog, we'll explore the concepts of localization and globalization in .NET and provide a detailed example using ASP.NET Web Forms (.NET Framework) with English (default), Spanish, and French languages.
Importance of Localization and Globalization in Modern Applications
In today's globalized world, applications need to be accessible to users from all over the world. Localization and globalization are essential for:
- Reaching a wider audience: By making your application available in multiple languages and regions, you can significantly increase your potential user base.
- Improving user experience: Users are more likely to engage with an application that is presented in their native language and understands their cultural conventions.
- Boosting sales and conversions: Localized applications can help you build trust with users and increase sales in international markets.
Introduction to Localization and Globalization
Globalization is the process of designing and developing applications that function for multiple cultures. It involves:
- Using culture-neutral data formats (e.g., dates, numbers).
- Ensuring the application can display content in different languages and formats.
Localization is the process of customizing an application for a specific culture or locale. It involves:
- Translating the user interface and content.
- Adapting to regional formats (e.g., date and time, currency).
Setting Up a New ASP.NET Web Forms Project
Prerequisites
- Ensure you have the latest version of Visual Studio installed.
- Make sure you have installed the ASP.NET and web development workload in the Visual Studio installer.
- Verify that you have the .NET Framework 4.72 or later installed in Visual Studio.
- Check that the .NET Framework Item Template for ASP.NET and web development is selected in the options.
1. Create a New Project
- Open Visual Studio.
- Create a new ASP.NET Web Application project.
- Choose the Web Forms template and name it LocalizationSample.
2. Add Global Resource Files
Right-click on the project and select Add > New Folder. Name it App_GlobalResources.
Add resource files for English (default), Spanish, and French:
- Resource.resx (default/English)
- Resource.es.resx (Spanish)
- Resource.fr.resx (French)
3.Configuring Localization Resources
Add the following key-value pairs to each resource file:
Resource.resx (default/English):
<data name="LabelFirstName" xml:space="preserve">
<value>First Name</value>
</data>
<data name="PlaceholderFirstName" xml:space="preserve">
<value>Enter First Name</value>
</data>
<data name="LabelLastName" xml:space="preserve">
<value>Last Name</value>
</data>
<data name="PlaceholderLastName" xml:space="preserve">
<value>Enter Last Name</value>
</data>
<data name="LabelEmail" xml:space="preserve">
<value>Email</value>
</data>
<data name="PlaceholderEmail" xml:space="preserve">
<value>Enter Email</value>
</data>
<data name="LabelDOB" xml:space="preserve">
<value>Date of Birth</value>
</data>
<data name="PlaceholderDOB" xml:space="preserve">
<value>Enter Date of Birth</value>
</data>
<data name="LabelSalary" xml:space="preserve">
<value>Salary</value>
</data>
<data name="ButtonSubmit" xml:space="preserve">
<value>Submit</value>
</data>
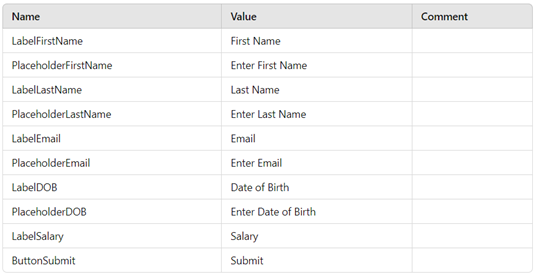
<data name="LabelFirstName" xml:space="preserve">
<value>Nombre</value>
</data>
<data name="PlaceholderFirstName" xml:space="preserve">
<value>Ingrese Nombre</value>
</data>
<data name="LabelLastName" xml:space="preserve">
<value>Apellido</value>
</data>
<data name="PlaceholderLastName" xml:space="preserve">
<value>Ingrese Apellido</value>
</data>
<data name="LabelEmail" xml:space="preserve">
<value>Correo Electrónico</value>
</data>
<data name="PlaceholderEmail" xml:space="preserve">
<value>Ingrese Correo Electrónico</value>
</data>
<data name="LabelDOB" xml:space="preserve">
<value>Fecha de Nacimiento</value>
</data>
<data name="PlaceholderDOB" xml:space="preserve">
<value>Ingrese Fecha de Nacimiento</value>
</data>
<data name="LabelSalary" xml:space="preserve">
<value>Salario</value>
</data>
<data name="ButtonSubmit" xml:space="preserve">
<value>Enviar</value>
</data>
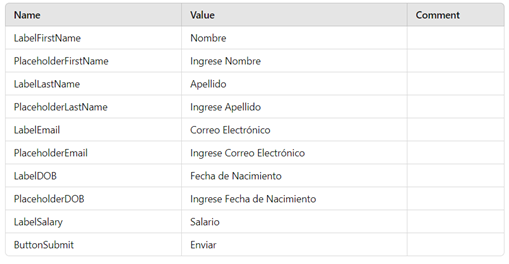
<data name="LabelFirstName" xml:space="preserve">
<value>Prénom</value>
</data>
<data name="PlaceholderFirstName" xml:space="preserve">
<value>Entrez Prénom</value>
</data>
<data name="LabelLastName" xml:space="preserve">
<value>Nom</value>
</data>
<data name="PlaceholderLastName" xml:space="preserve">
<value>Entrez Nom</value>
</data>
<data name="LabelEmail" xml:space="preserve">
<value>Email</value>
</data>
<data name="PlaceholderEmail" xml:space="preserve">
<value>Entrez Email</value>
</data>
<data name="LabelDOB" xml:space="preserve">
<value>Date de Naissance</value>
</data>
<data name="PlaceholderDOB" xml:space="preserve">
<value>Entrez Date de Naissance</value>
</data>
<data name="LabelSalary" xml:space="preserve">
<value>Salaire</value>
</data>
<data name="ButtonSubmit" xml:space="preserve">
<value>Soumettre</value>
</data>
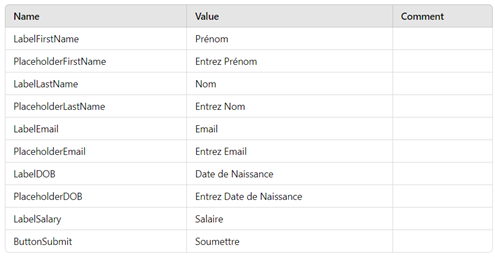
4. Creating a Localized Form
Add the following code to Default page, if not available create a web form by right clicking on your project > Add > New Item > web form name it as Default.aspx
Default.aspx:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html>
<html>
<head runat="server">
<title>Localization Sample</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="lblFirstName" runat="server" Text="Label"></asp:Label>
<asp:TextBox ID="txtFirstName" runat="server" placeholder=""></asp:TextBox>
<br />
<asp:Label ID="lblLastName" runat="server" Text="Label"></asp:Label>
<asp:TextBox ID="txtLastName" runat="server" placeholder=""></asp:TextBox>
<br />
<asp:Label ID="lblEmail" runat="server" Text="Label"></asp:Label>
<asp:TextBox ID="txtEmail" runat="server" placeholder=""></asp:TextBox>
<br />
<asp:Label ID="lblDOB" runat="server" Text="Label"></asp:Label>
<asp:TextBox ID="txtDOB" runat="server" placeholder=""></asp:TextBox>
<br />
<asp:Label ID="lblSalary" runat="server" Text="Label"></asp:Label>
<asp:TextBox ID="txtSalary" runat="server" placeholder=""></asp:TextBox>
<br />
<asp:Button ID="btnSubmit" runat="server" Text="Button" OnClick="SubmitForm" />
</div>
</form>
</body>
</html>
Default.aspx.cs:
using System;
using System.Globalization;
using System.Threading;
using System.Web.UI;
public partial class _Default : Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
SetCulture();
LocalizeForm();
}
}
private void SetCulture()
{
string culture = Request.QueryString["lang"];
if (string.IsNullOrEmpty(culture))
{
culture = "en"; // Default culture
}
Thread.CurrentThread.CurrentCulture = new CultureInfo(culture);
Thread.CurrentThread.CurrentUICulture = new CultureInfo(culture);
}
private void LocalizeForm()
{
lblFirstName.Text = GetLocalizedString("LabelFirstName");
txtFirstName.Attributes["placeholder"] = GetLocalizedString("PlaceholderFirstName");
lblLastName.Text = GetLocalizedString("LabelLastName");
txtLastName.Attributes["placeholder"] = GetLocalizedString("PlaceholderLastName");
lblEmail.Text = GetLocalizedString("LabelEmail");
txtEmail.Attributes["placeholder"] = GetLocalizedString("PlaceholderEmail");
lblDOB.Text = GetLocalizedString("LabelDOB");
txtDOB.Attributes["placeholder"] = GetLocalizedString("PlaceholderDOB");
lblSalary.Text = GetLocalizedString("LabelSalary");
btnSubmit.Text = GetLocalizedString("ButtonSubmit");
}
private string GetLocalizedString(string key)
{
return GetGlobalResourceObject("Resource", key)?.ToString() ?? key;
}
protected void SubmitForm(object sender, EventArgs e)
{
DateTime dob = DateTime.Parse(txtDOB.Text);
decimal salary = decimal.Parse(txtSalary.Text);
// Handle form submission
}
}
5.Handling Date, Time, Number, and Currency Formats
To ensure date, time, number, and currency formats are correctly handled according to the selected culture, use the Thread.CurrentThread.CurrentCulture settings. Here is an example of parsing and displaying date and currency values:
protected void SubmitForm(object sender, EventArgs e)
{
try
{
DateTime dob = DateTime.Parse(txtDOB.Text, CultureInfo.CurrentCulture);
decimal salary = decimal.Parse(txtSalary.Text, CultureInfo.CurrentCulture);
lblResult.Text = string.Format(
CultureInfo.CurrentCulture,
"Date of Birth: {0:D}<br/>Salary: {1:C}",
dob,
salary
);
}
catch (FormatException ex)
{
lblResult.Text = "Invalid input format.";
}
}
6. Testing Localization
1. Run the Application:- Start the web application in Visual Studio.
- Append ?lang=es to the URL to view the Spanish version.
- Append ?lang=fr to the URL to view the French version.
- Ensure that the labels, placeholders, button text, and date/currency formats are displayed correctly according to the selected language and culture.
Conclusion
By following this guide, you can effectively implement localization and globalization in your ASP.NET Web Forms application. This ensures a personalized user experience for different cultures and languages, enhancing the accessibility and usability of your web application.
Feel free to customize and expand this example to suit your specific localization and globalization needs.