20th May 2024
An Introductory Guide to Programming Logic for Beginners
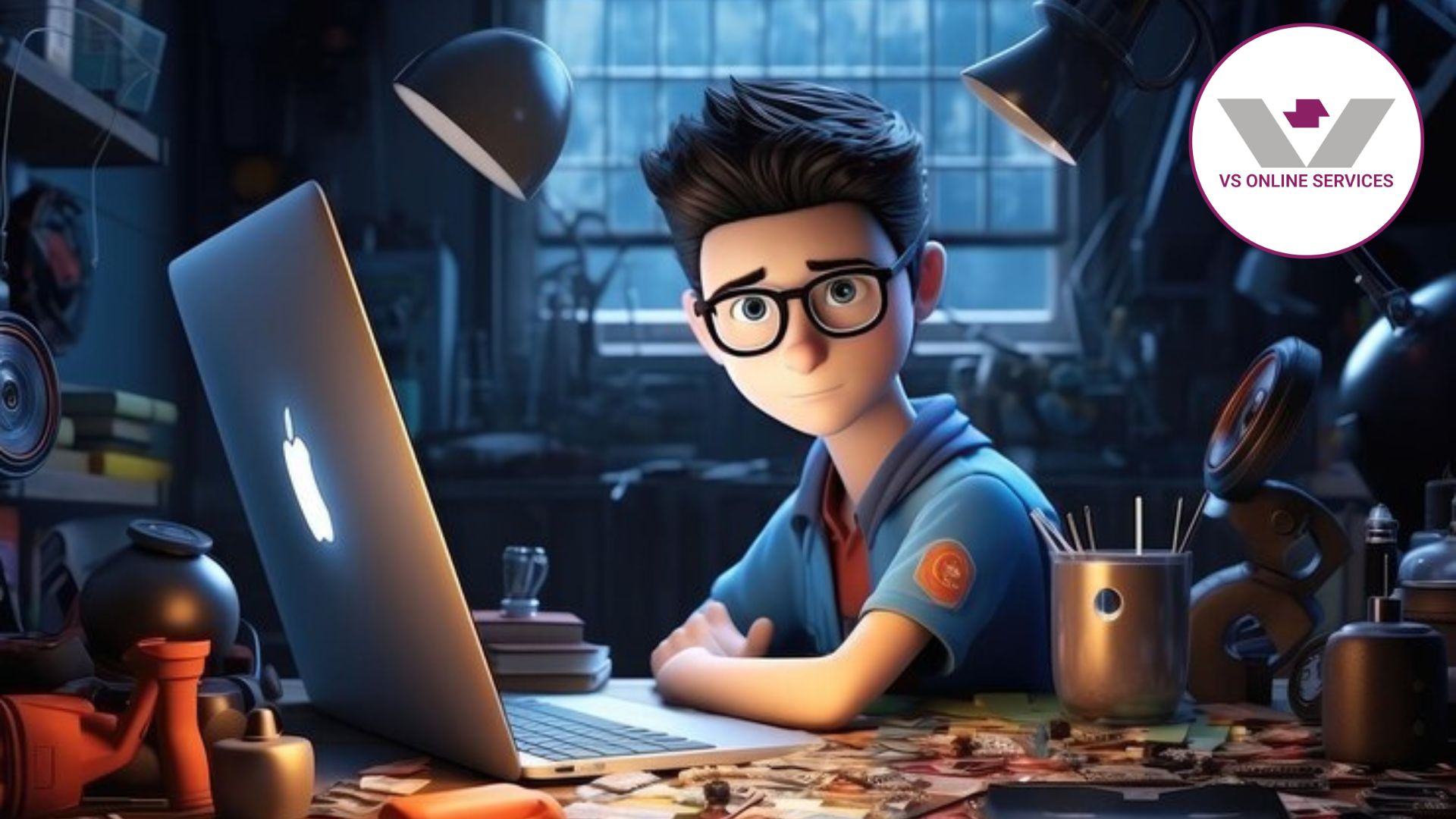
Introduction to Programming Logic
Welcome to the exciting world of programming! In this guide, we'll embark on a journey to understand the fundamental concepts behind creating computer programs. Even if you've never written a single line of code before, don't worry! This guide is designed for beginners and will break down programming logic into easy-to-grasp steps.
Programming logic is like a set of instructions you give to a computer. It's a roadmap that tells the computer what steps to take to solve a problem or complete a task. Just like you wouldn't start a journey without a plan, programming logic ensures your computer follows a clear path to achieve the desired outcome.
By understanding programming logic, you'll gain valuable skills like:
- Problem-solving: Learn to break down complex challenges into smaller, more manageable steps.
- Critical thinking: Develop the ability to analyze situations, identify patterns, and design solutions.
- Communication: Translate your ideas into clear instructions that a computer can understand.
These skills are not only valuable for programmers but can be applied to various aspects of life! So, whether you're a student, a curious individual, or someone who wants to build the next big app, understanding programming logic is a great foundation.
Basic Concepts of Programming Logic
Now that you're familiar with the concept of programming logic, let's explore some fundamental building blocks:
Sequencing:
Imagine giving instructions to a friend on how to change a tire. You would tell them:
- Loosen the lug nuts: This is the first step, followed by...
- Jack up the car: Only after loosening the nuts can they safely lift the car.
- Remove the flat tire: Once the car is raised, they can take off the flat tire.
- Put on the spare tire: Now that the flat tire is removed, they can install the spare.
- Lower the car and tighten the lug nuts: Finally, they can lower the car and secure the spare tire with the lug nuts.
In programming logic, we can translate these steps into a sequence of instructions for the computer to follow. This ensures the actions are performed in the correct order, mimicking how you would guide your friend through the process.
Selection (Conditional Statements):
Let's say you're writing a program to control a home heating system. You want the system to turn on the heater if the temperature falls below a certain point (e.g., 18°C). Here's a simplified example of a conditional statement:
if (temperature < 18) {
turnOnHeater();
} else {
// Keep the heater off
}
In this example, the computer checks the temperature variable. If it's less than 18°C (the condition is true), the turnOnHeater() function is executed. Otherwise, the heater remains off.
Loops (Iteration):
Imagine you're writing a program to calculate the total cost of groceries in your shopping cart. You have a list of items with individual prices. A loop can help you iterate through each item, adding its price to the running total.
Here's a basic example (pseudocode):
totalCost = 0
for each item in shoppingCart:
totalCost = totalCost + item.price
print("Total cost:", totalCost)
This loop iterates through each item in the shoppingCart list, adding its price to the total cost variable. Once all items are processed, the final total cost is displayed.
Input and Output:
Suppose you're building a simple quiz application. You want to display questions on the screen and collect user answers. Input allows the program to receive user input through methods like keyboard presses or mouse clicks. This input can then be stored in variables and used for further processing.
Output allows the program to display information back to the user. In our quiz app, it can display the question, record the chosen answer, and show the results.
Variables:
Think of variables like labeled boxes you use to store things. In programming logic, these boxes hold data that the program can access and manipulate. For example, a variable named name could store a person's name (text data), while age could hold their age (numerical data). You can then use these variables throughout your program to personalize the experience or perform calculations.
By understanding and applying these basic concepts with examples, you'll be well on your way to mastering programming logic and building your own computer programs!
Data Types and Structures
In the previous section, we explored the fundamental building blocks of programming logic. Now, let's delve deeper into how we organize information within our programs:
Data Types:
Data types define the kind of information a variable can hold. They act like labels on our storage boxes, specifying what type of data we're storing (text, numbers, true/false values, etc.). Common data types include:
- Integers: Whole numbers (e.g., 10, -5)
- Floats: Numbers with decimal points (e.g., 3.14, -12.5)
- Strings: Text data (e.g., "Hello, world!", "This is my name")
- Booleans: True or false values (e.g., true, false)
Using the appropriate data type ensures the computer handles the information correctly. Imagine trying to store a name (text) in a box meant for numbers (integers) - it wouldn't work well!
Data Structures:
Data structures are more complex ways of organizing data collections. These "containers" allow us to group related data items in a structured manner. Here are some common data structures:
- Arrays: Ordered lists of items, accessed by an index (position). Imagine a shopping list where each item is at a specific spot.
- Lists: Similar to arrays, but the order might not be strictly enforced. Think of a grocery list where items can be added or removed without affecting the overall structure.
- Dictionaries (or Objects): Collections of key-value pairs. Like a phonebook, you can look up information (value) using a unique key (name).
- Sets: Unordered collections of unique items. Imagine a set of unique playing cards, where duplicates are not allowed.
Choosing the right data structure depends on how you need to access and manipulate the data within your program.
Benefits of Using Data Types and Structures:
- Improved Organization: Data is well-defined and easier to understand and manage.
- Reduced Errors: Using the correct data type helps prevent errors caused by incompatible data.
- Efficient Processing: Data structures allow for faster access and manipulation of data based on their organization.
By understanding and utilizing data types and structures, you'll be able to create clean, efficient, and well-organized programs that handle information effectively.
Functions and Procedures
As you start building more complex programs, you'll encounter repetitive tasks. Imagine writing the same set of instructions to calculate the area of a rectangle multiple times. Functions and procedures come to the rescue, promoting code reusability and modularity.
Functions:
- Functions are reusable blocks of code that perform a specific task. They take inputs (parameters) and often return an output (a value or result).
- Think of functions like mini-programs within your main program. You can give them a descriptive name and call them whenever you need to perform the defined task.
function calculateArea(length, width) {
const area = length * width;
return area;
}
// Calling the function and using the returned value
const rectangleArea = calculateArea(5, 10);
console.log("Area of the rectangle:", rectangleArea);
In this example, the “calculateArea” function takes two parameters (length and width) and returns the calculated area. You can call this function multiple times with different values to calculate the area for various rectangles.
Procedures:
- Procedures are similar to functions but don't necessarily return a value. They primarily focus on performing actions or modifying data within the program.
- Imagine a procedure like a recipe that outlines a series of steps without necessarily producing a specific output dish (though it might change ingredients during the process).
function greetUser(name) {
console.log("Hello, " + name + "!");
}
greetUser("Alice"); // This procedure simply prints a greeting message
The “greetUser” procedure takes a name as input and prints a personalized greeting message. It doesn't return a value, but it modifies the program's output by displaying the greeting.
Benefits of Using Functions and Procedures:
- Code Reusability: You can write the logic once and use it multiple times, reducing code duplication and making your program more concise.
- Improved Readability: Breaking down complex tasks into functions and procedures makes your code easier to understand and maintain.
- Modularity: Functions and procedures promote modular programming, where the program is organized into smaller, independent units.
By mastering functions and procedures, you'll be able to write more efficient, maintainable, and well-structured programs. In the next section, we'll explore different programming paradigms, which are overarching approaches to problem-solving in the programming world.
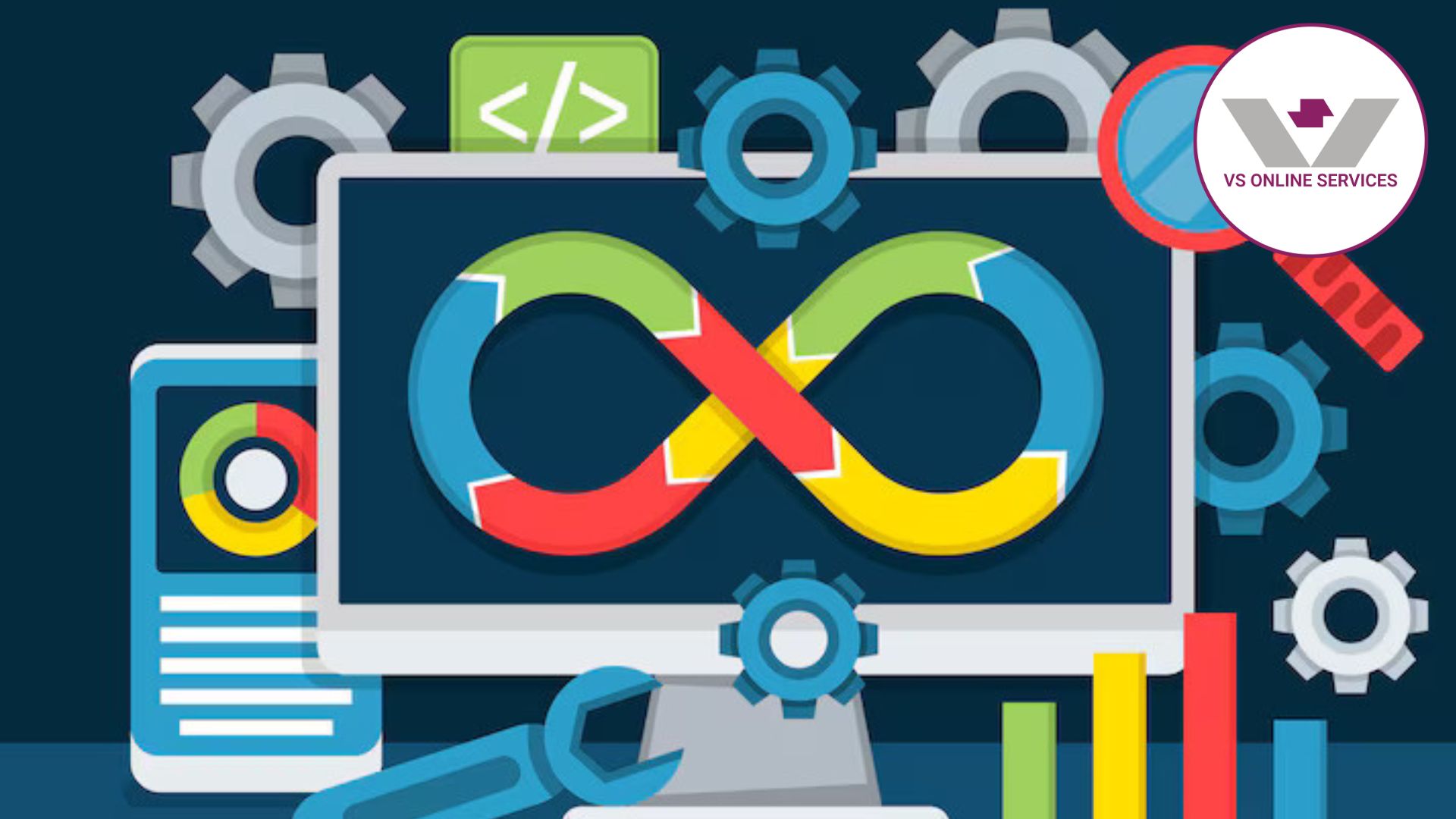
So far, we've explored the building blocks and fundamental concepts of programming logic. Now, let's delve into a broader perspective: programming paradigms.
What are Programming Paradigms?
A programming paradigm is a fundamental style of problem-solving and programming language design. It defines how a programmer conceptualizes the solution and how the language structures the code. Different paradigms offer unique strengths and weaknesses, making them suitable for different types of problems.
Common Programming Paradigms:
Imperative Programming:- Focuses on giving explicit instructions to the computer, step-by-step, to achieve the desired outcome.
- Often involves variables, data types, and control flow statements (loops, conditionals).
- Example languages: C, Java, Python (can also be used in an object-oriented way).
- Focuses on what the program needs to achieve rather than how it should achieve it.
- The programmer specifies the desired outcome or problem to be solved, and the language handles the execution details.
- Example languages: SQL (for database queries), Haskell (functional language).
- Organizes code around objects, which encapsulate data (properties) and behavior (methods).
- Objects interact with each other through messages and method calls.
- Example languages: Java, C++, Python (which can also be used in an imperative way).
- View programs as a series of pure functions that transform data.
- Functions avoid side effects (changing global state) and are focused on returning new data based on the input.
- Example languages: Haskell, Lisp, JavaScript (can also be used in an imperative way).
Choosing the Right Paradigm:
The choice of programming paradigm depends on the specific problem you're trying to solve and your preferences. Here's a simplified guideline:
- Imperative: Good for general-purpose programming, tasks with clear sequences of steps.
- Declarative: Ideal for data manipulation, configuration, expressing relationships between data elements.
- Object-Oriented: Effective for modeling real-world entities with properties and behaviors, building complex systems with reusable components.
- Functional: Well-suited for problems that can be broken down into smaller, pure functions, promoting code maintainability and immutability.
Problem-Solving Techniques for Programming
Now that you've grasped the core concepts and paradigms of programming, let's delve into the art of problem-solving. Programming is as much about logic and creativity as it is about syntax and code. Here are some valuable techniques to approach programming challenges effectively:
Define the Problem Clearly:
The first step is to understand the problem you're trying to solve. Read the problem statement carefully, identify the inputs and expected outputs, and break down any complex functionalities into simpler components. Ask clarifying questions if needed.
Develop a Plan (Algorithm):
Once you understand the problem, outline a plan (algorithm) that details the steps the program will take to achieve the desired outcome. This algorithm can be written in pseudocode (informal language resembling actual code) or a flowchart that visually represents the steps and decision points.
Break Down into Smaller Problems:
Large problems can often feel overwhelming. Try to decompose the problem into smaller, more manageable subproblems. Solve each subproblem individually, and then combine the solutions to form the overall solution.
Use Examples and Test Cases:
Think of test cases as sample inputs and their corresponding expected outputs. Create a set of test cases to verify if your solution works correctly under various conditions. This helps identify and address potential errors early on.
Choose Appropriate Data Structures and Algorithms:
The efficiency and clarity of your program depend on the data structures and algorithms you choose. Select data structures that fit the type of data you're working with and algorithms that efficiently solve the problem at hand.
Write Readable and Maintainable Code:
As your code grows, readability and maintainability become crucial. Use clear variable names, comments to explain your logic, and proper indentation to structure your code. This makes it easier for you and others to understand and modify the code in the future.
Debug and Test Thoroughly:
No program is perfect! Debugging involves identifying and fixing errors in your code. Use print statements or a debugger to trace the execution of your program and pinpoint where issues arise. Test your code rigorously with various inputs to ensure it behaves as expected.
By mastering these problem-solving techniques, you'll be well-equipped to tackle programming challenges with confidence and creativity.
Conclusion
Congratulations! You've reached the end of this introductory guide to programming logic. This journey has equipped you with the fundamental concepts and tools to start exploring the world of programming.
By understanding the basics of programming logic, data types, structures, functions, and problem-solving techniques, you've laid a solid foundation for building your programming skills. Remember, the key is to keep practicing, experimenting, and learning from your experiences.
Here are some additional resources to fuel your programming journey:
Online Coding Platforms:- HackerRank (https://www.hackerrank.com/)
- LeetCode (https://leetcode.com/)
- Codecademy (https://www.codecademy.com/catalog)
- W3Schools (https://www.w3schools.com/)
- Tutorialspoint(https://www.tutorialspoint.com/)