28th Nov 2022
Here are the steps to implement JWT authentication in ASP.NET Core 6
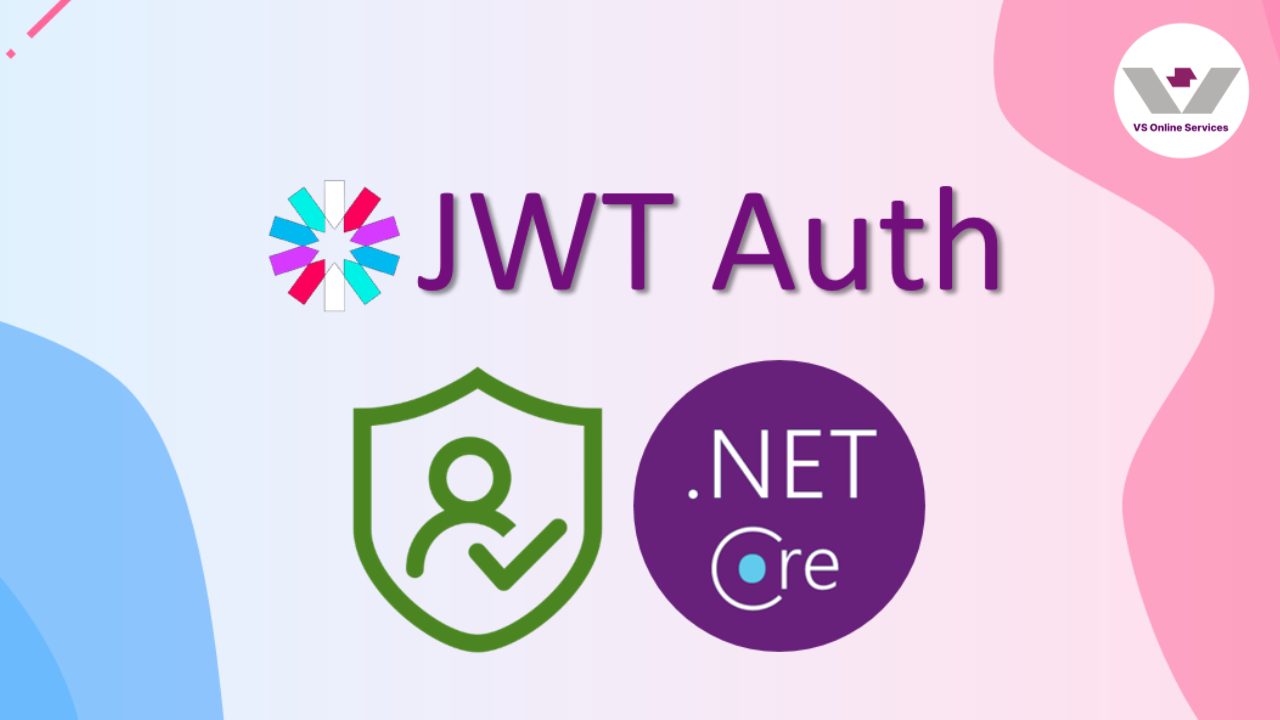
Step 1: Install the required NuGet packages To get started with JWT authentication in ASP.NET Core 6, you need to install the following NuGet packages:
- Microsoft.AspNetCore.Authentication.JwtBearer
- Microsoft.IdentityModel.Tokens
You can install these packages using the NuGet Package Manager in Visual Studio, or by running the following commands in the Package Manager Console
Install-Package Microsoft.AspNetCore.Authentication.JwtBearer
Install-Package Microsoft.IdentityModel.Tokens
Step 2: Configure JWT authentication in your app
In your ASP.NET Core 6 app, open the Program.cs file and add the following code inside the ConfigureServices method:
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme).AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = false,
ValidateIssuerSigningKey = true,
ValidIssuer = "Jwt:Issuer",
ValidAudience = "Jwt:Audience",
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("Jwt:Key"))
};
});
This code configures the JWT authentication middleware for your app and sets up the options for token validation. You'll need to replace the values for Jwt:Issuer, Jwt:Audience, and Jwt:Key with your own values.
And also add
app.UseAuthentication();
Step 3:Add authorization to your API endpoints
To protect your API endpoints with JWT authentication, you need to add the [Authorize] attribute to your controller or action methods. For example:
[Authorize]
[HttpGet]
public string HelloWorld()
{
return "HelloWorld";
}
This code adds the [Authorize] attribute to the Get method, which means that only authenticated users with a valid JWT token can access this endpoint.
Generate JWT tokens To generate JWT tokens for your users, you can use a library like
System.IdentityModel.Tokens.Jwt.
Here's an example of how to generate a JWT token:
public string GenerateToken()
{
var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("Jwt:Key"));
var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256);
var token = new JwtSecurityToken(
issuer: "Jwt:Issuer",
audience: "Jwt:Audience",
claims: new[] {
new Claim("sub", "user1")
},
expires: DateTime.Now.AddHours(1),
signingCredentials: creds);
var jwtToken = new JwtSecurityTokenHandler().WriteToken(token);
return jwtToken;
}
This code generates a JWT token that is valid for 1 hour, using the values for Jwt:Issuer, Jwt:Audience, and Jwt:Key from your configuration.
With these steps, you can implement JWT authentication in your ASP.NET Core 6 app.
About Us
- VS Online Services : Custom Software Development
VS Online Services has been providing custom software development for clients across the globe for many years - especially custom ERP, custom CRM, Innovative Real Estate Solution, Trading Solution, Integration Projects, Business Analytics and our own hyperlocal e-commerce platform vBuy.in and vsEcom.
We have worked with multiple customers to offer customized solutions for both technical and no technical companies. We work closely with the stake holders and provide the best possible result with 100% successful completion To learn more about VS Online Services Custom Software Development Solutions or our product vsEcom please visit our SaaS page, Web App Page, Mobile App Page to know about the solution provided. Have an idea or requirement for digital transformation? please write to us at siva@vsonlineservices.com