14th Aug 2023
How to Implement RxJS in Angular
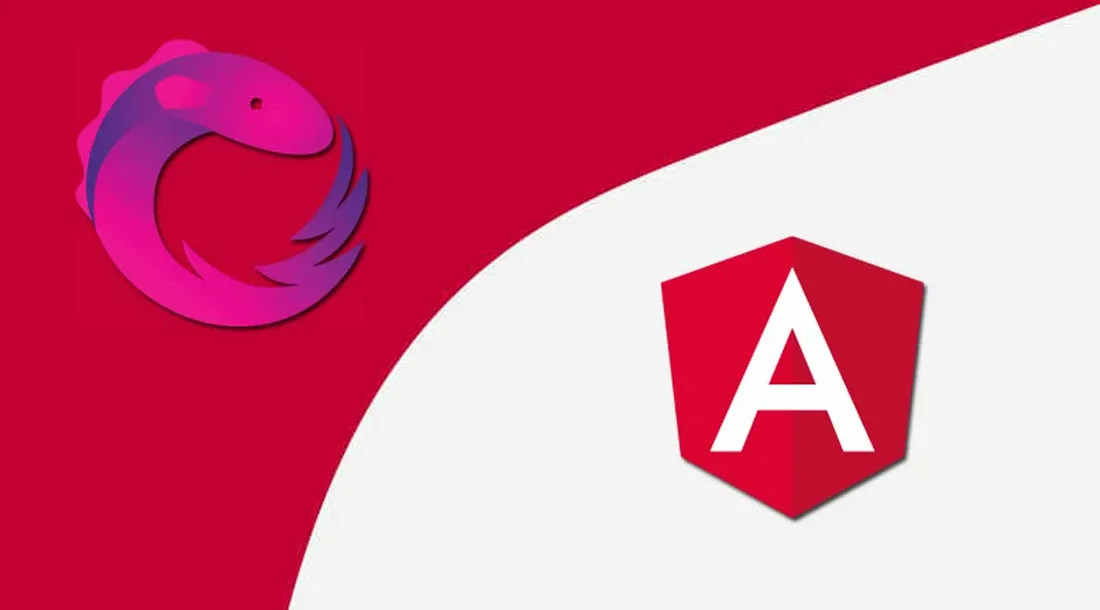
Angular
Angular is a popular web application framework for building dynamic and robust single-page applications (SPAs). Angular is developed and maintained by Google. It allows developers to create efficient and maintainable web applications by providing a structured way to manage and organize code. Angular applications are built using components, which are reusable, self-contained building blocks that encapsulate the HTML, CSS, and logic for a specific part of the user interface.
Angular RxJS
RxJS (Reactive Extensions for JavaScript) is a library that provides a powerful way to work with asynchronous data streams. In an Angular operation, RxJS is frequently used to handle colorful tasks related to asynchronous programming, similar as managing HTTP requests, handling stoner input, and dealing with events.
Angular and RxJS key concepts
- Observables Observables are the core structure blocks of RxJS. They represent a stream of data that can be observed over time. Observables can emit values, errors, and completion signals, and they are used to handle asynchronous data sources like HTTP requests, timers, and user events.
- Subscribing to Observables When you create an observable, it's like setting up a stream of data. Subscribing to an observable means you're listening to that stream and reacting to the data it emits. In other words, it's how you start receiving and handling values, errors, and completion signals emitted by the observable.
- Unsubscribing to Observables Unsubscribing from an observable is an important practice in RxJS to prevent memory leaks and unnecessary resource usage. When you subscribe to an observable, a subscription object is returned. Calling the unsubscribe () method on this subscription object will terminate the subscription, stopping further emissions from the observable and releasing any resources associated with it.
- BehaviorSubject BehaviorSubject is a type of subject in RxJS, which is both an observable and an observer. It allows you to multicast a value or event to multiple subscribers, and it keeps track of the most recent value emitted. This makes it particularly useful for scenarios where you want subscribers to receive the most recent value immediately upon subscription.
Implement RxJS in Angular
Create a new component using the Angular CLI
ng generate component <Component Name>
EmitterComponent.html file to include subscriber components
<div>
<h2>Emitter Component</h2>
<button (click)="emitMessage()">Emit Message</button>
<app-subscriber></app-subscriber>
</div>
‘EmitterComponent.ts’ file and implement the observable
import { Component, OnInit } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
@Component({
selector: 'app-emitter',
templateUrl: './emitter.component.html',
styleUrls: ['./emitter.component.css']
})
export class EmitterComponent {
private messageSource = new BehaviorSubject<string>(''); // Initial value is empty
message$ = this.messageSource.asObservable();
emitMessage() {
this.messageSource.next('Hello from Emitter Component!');
}
}
‘subscriber.component.ts’ file and implement the subscription
import { Component, OnInit, OnDestroy } from '@angular/core';
import { Observable, Subscription, timer } from 'rxjs';
import { take, map, catchError } from 'rxjs/operators';
import { EmitterComponent } from '../emitter/emitter.component';
@Component({
selector: 'app-subscriber',
templateUrl: './subscriber.component.html',
styleUrls: ['./subscriber.component.css']
})
export class SubscriberComponent implements OnInit, OnDestroy {
private messageSubscription: Subscription | undefined;
message: string = '';
constructor(private emitter: EmitterComponent) {}
ngOnInit() {
this.messageSubscription = this.emitter.message$.subscribe((message) => {
this.message = message;
});
}
ngOnDestroy() {
if( this.messageSubscription){
this.messageSubscription.unsubscribe();
}
}
}
subscriber.component.html
<div>
<h2>Subscriber Component</h2>
<p>{{ message }}</p>
</div>
app-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { EmitterComponent } from './emitter/emitter.component';
const routes: Routes = [
{
path:"",
component:EmitterComponent
}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
app-module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { EmitterComponent } from './emitter/emitter.component';
import { SubscriberComponent } from './subscriber/subscriber.component';
@NgModule({
declarations: [
AppComponent,
EmitterComponent,
SubscriberComponent
],
imports: [
BrowserModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Run the application using the Angular CLI
ng serve
The EmitterComponent emits messages using a BehaviorSubject called messageSource.
The SubscriberComponent subscribes to the message$ observable of the EmitterComponent and displays the emitted message.
The EmitterComponent and SubscriberComponent are communicating through the observable mechanism.
Before click Tigger button:
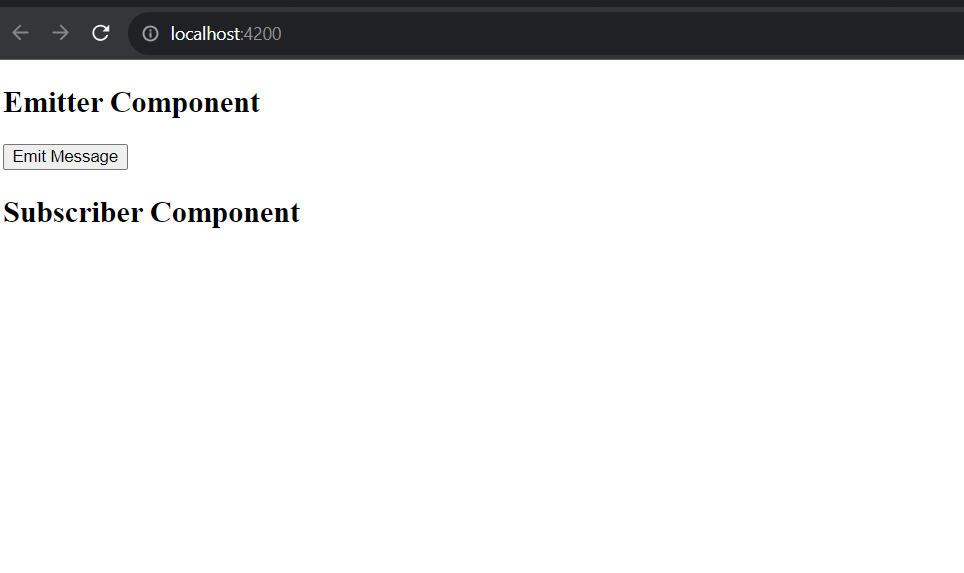
After Click Tigger button:
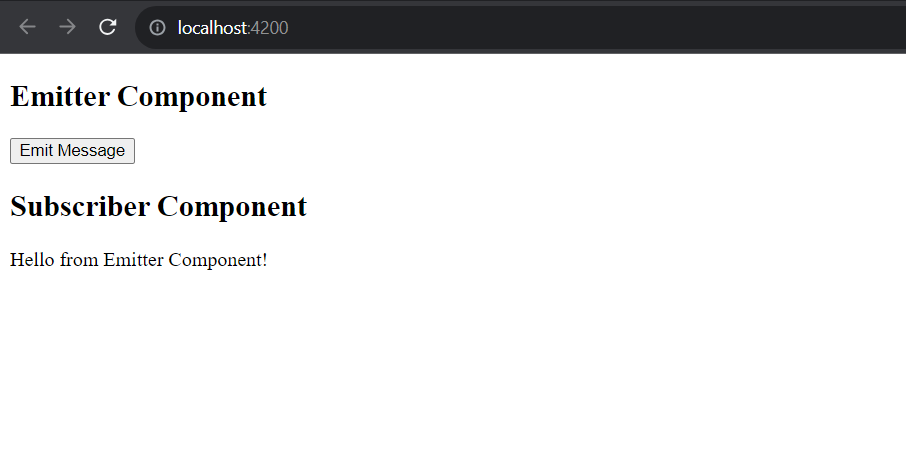
Conclusion
RxJS (Reactive Extensions for JavaScript) is a powerful library for managing asynchronous and event-driven programming in Angular applications. It provides a rich set of tools and operators to work with reactive programming concepts, allowing you to efficiently handle complex asynchronous scenarios, such as HTTP requests, user interactions, and real-time updates.