12th Jun 2023
Implementing WebSocket in Node.js
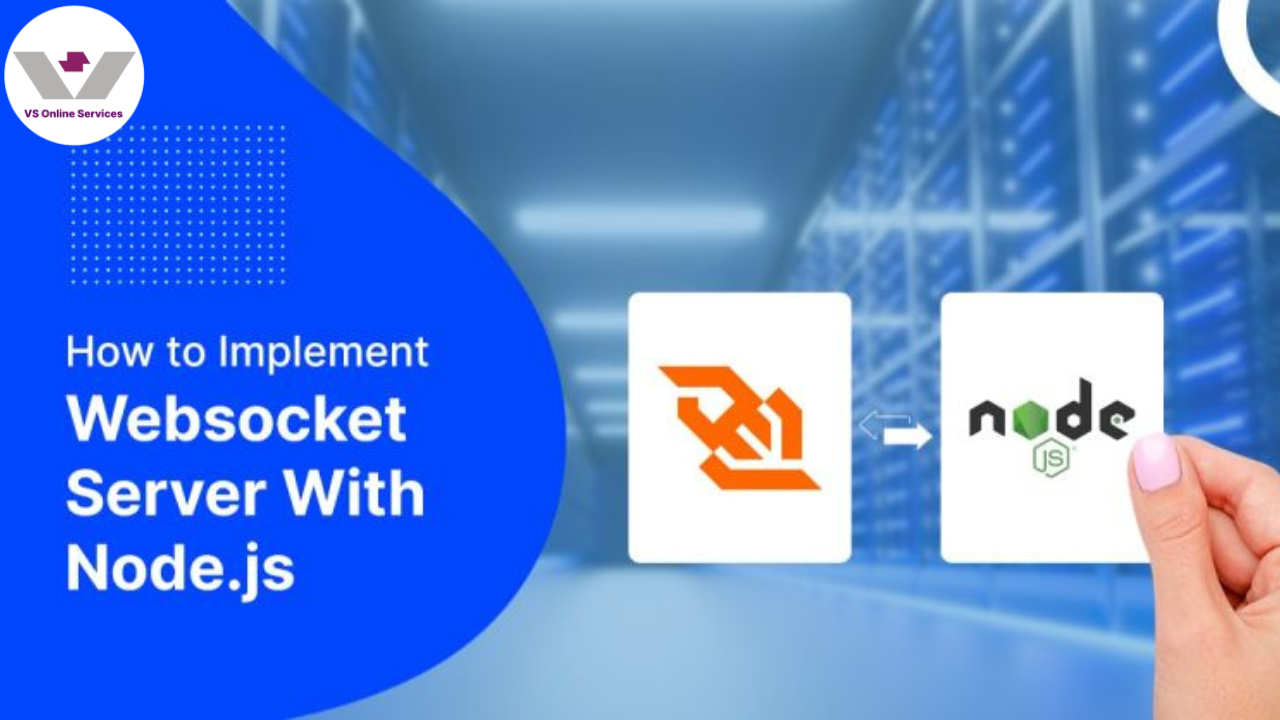
Node.js is a JavaScript runtime environment that allows you to execute JavaScript code outside of a web browser. It provides a rich set of libraries and frameworks for building various types of applications, including web applications.
One of the popular use cases of Node.js is creating WebSocket servers. WebSocket is a communication protocol that enables real-time, bi-directional communication between a client and a server over a single TCP connection. It provides a persistent connection that allows data to be sent and received simultaneously, without the need for repeated requests and responses. In the context of Node.js, you can use various libraries and modules to implement WebSocket functionality. One commonly used library is ws.
Why WebSocket
Real-time communication: WebSocket provide a persistent, bidirectional communication channel between the client and the server. Unlike traditional HTTP requests, which are stateless and require the client to initiate communication, WebSocket allow for real-time, instant communication between the server and the client. This is particularly useful for applications that require live updates, real-time collaboration, instant messaging, or any scenario where real-time interaction is essential.
WebSocket client and server implementation
The WebSocket server listens on a specified port and waits for WebSocket connection requests from clients. Once a client initiates a WebSocket connection, the server performs a handshake process to establish the connection. The handshake involves exchanging specific headers and information between the client and server to confirm compatibility and upgrade the connection from HTTP to the WebSocket protocol.
Let’s start by creating the Server side implementation, to do so in your project directory create a file name ‘server.js’. Then lets installed the WebSocket dependency ‘ws’ as discussed above.
Install the ws library by running npm install ws in your project directory.
npm install ws
Add the below code to ‘server.js’ file
const WebSocket = require('ws');
const server = new WebSocket.Server({ port: 8080 });
Event listener for WebSocket server connection
The event listener for WebSocket server connection refers to a function or callback that is executed when a new client establishes a WebSocket connection with the server. It is associated with the 'connection' event of the WebSocket server.
When a client initiates a WebSocket connection and successfully completes the handshake process with the server, the server emits a 'connection' event. This event indicates that a new client has connected to the server, and the associated event listener is triggered or called.
server.on('connection', (socket) => {
console.log('Client connected');
});
Event listener for receiving messages from the client
The event listener for receiving messages from the client refers to a function or callback that is executed when the WebSocket server receives a message from a connected client. It is associated with the 'message' event of a WebSocket connection object. When a client sends a message to the server through a WebSocket connection, the server emits a 'message' event. This event indicates that a new message has been received from the client, and the associated event listener is triggered or called. The event listener function for the 'message' event typically takes a parameter that represents the received message.
socket.on('message', (message) => {
console.log('Received message:', message);
// Send a response back to the client
socket.send('Server received your message: ' + message);
});
Event listener for WebSocket connection close
The event listener for WebSocket connection close refers to a function or callback that is executed when a WebSocket connection between the server and a client is closed. It is associated with the 'close' event of a WebSocket connection object.
socket.on('close', () => {
console.log('Client disconnected');
});
The entire ‘server.js’ file
const WebSocket = require('ws');
// Create a WebSocket server
const server = new WebSocket.Server({ port: 8080 });
// Event listener for WebSocket server connection
server.on('connection', (socket) => {
console.log('Client connected');
// Event listener for receiving messages from the client
socket.on('message', (message) => {
console.log('Received message:', message);
// Send a response back to the client
socket.send('Server received your message: ' + message);
});
// Event listener for WebSocket connection close
socket.on('close', () => {
console.log('Client disconnected');
});
});
WebSocket Client (client.js)
In another directory create a index.html and paste the below codeWe will use a simple HTML file with the client.js file to understand how client side implementation works.
<!DOCTYPE html>
<thtml>
<thead>
<title>Button Example</title>
<script src="./client.js"></script>>
</head>
<body>
<input type="text" id="message" placeholder="enter message"/>>
<button id="myButton" onclick="sendMessage()">Send</button>
<br>
<h2 id="returnMessage"></h2>
</body>
</html>
Create a ‘client.js’ file in the same directory as the HTML file, this is where we will follow the steps to implement the WebSocket in the client side.
To create the socket which points to the server paste the below code in ‘client.js’.
const socket = new WebSocket('ws://localhost:8080');
Event listener for WebSocket connection open
The event listener for WebSocket connection open refers to a function or callback that is executed when a WebSocket connection between the server and a client is successfully established and opened. It is associated with the 'open' event of a WebSocket connection object. The event listener function for the 'open' event typically does not take any parameters. However, you may have access to the WebSocket connection object itself within the event listener.
Configure Serilog in your application, typically in the Main method or in the application Program.cs:
ws.onopen = function () {
ws.send("Message to send");
};
Event listener for receiving messages from the server
The WebSocket client library provides an event listener or callback function that you can define to handle incoming messages from the server. This event listener is triggered when the client receives a message from the server and the server receives a message from the client.
ws.onmessage = function (message) {
console.log("Received message:", message.data);
};
vent listener for WebSocket connection close
The event listener for WebSocket connection close refers to a function or callback that is executed when a WebSocket connection between the server and a client is closed. It is associated with the 'close' event of a WebSocket connection object.
ws.onclose = function () {
console.log("Disconnected from server");
};
The entire ‘client.js’ file
// Create a WebSocket connection to the server
const ws = new WebSocket("ws://localhost:8080");
ws.onopen = function () {
ws.send("Message to send");
};
// // Event listener for receiving messages from the server
ws.onmessage = function (message) {
document.getElementById("returnMessage").innerHTML = message.data;
console.log("Received message:", message.data);
};
// // Event listener for WebSocket connection close
ws.onclose = function () {
console.log("Disconnected from server");
};
function sendMessage() {
debugger;
let messages = document.getElementById("message");
if (ws.readyState === WebSocket.OPEN) {
ws.send(messages.value);
} else {
console.log("WebSocket connection is not open");
}
}
Now our sample project set-up is done, lets start by running the server side code. To do so, go to the server side implementation directory and run the following command in terminal
node Server.js
Now server side is ready, lets initiate the connection from the client side. Go to the client side directory and open the html file in any browser.
*image after opening HTML file in browser*
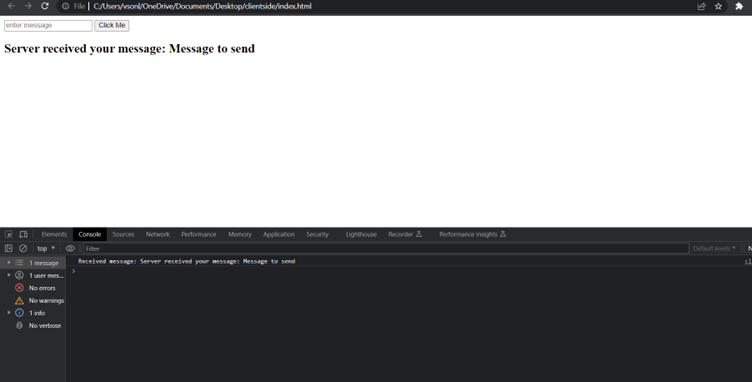
Now in the input we can enter any input, this will be sent to the server side and will be returned with additional text.
E.g. Enter ‘Hello’ in the input and click ‘Send’. This message will be sent to the server via WebSocket and will be returned as ‘Server received your message: Hello’
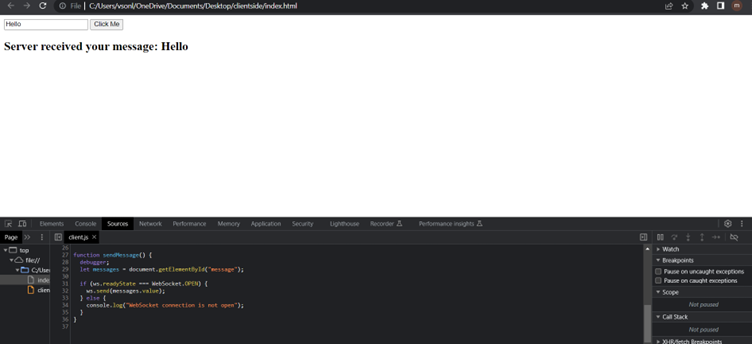
Conclusion
Overall, by leveraging Node.js to implement WebSockets, developers can create highly responsive, scalable, and interactive web applications that provide real-time communication and enhance the overall user experience.