24th May 2024
Angular Inheritance Demystified: Components and Services
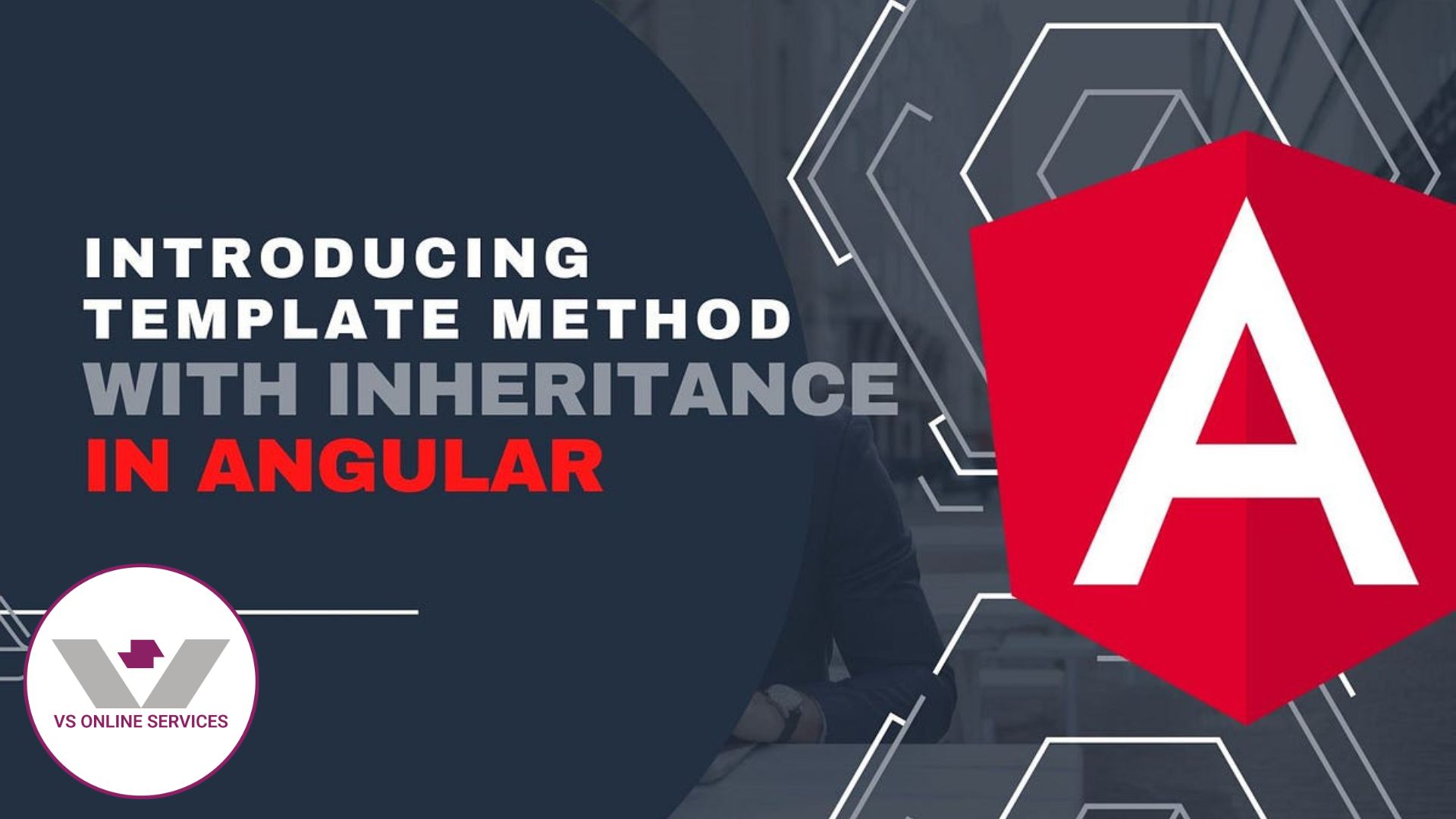
Introduction to Inheritance
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows a class to inherit properties and methods from another class. The class that inherits is called the derived class or child class, while the class being inherited from is known as the base class or parent class. This mechanism promotes code reuse, simplifies maintenance, and enables polymorphism, where objects of different classes can be treated as objects of a common superclass.
Key features of inheritance include:
- Code Reusability: Allows sharing of common logic among multiple classes without code duplication.
- Extensibility: Enables extending existing functionalities without modifying the original code.
Introduction to Inheritance in Angular
Angular, a popular framework for building web applications, leverages TypeScript, which is an object-oriented language supporting inheritance. In Angular, inheritance is commonly used in both components and services to promote code reuse and maintainability.
Component Inheritance
Component inheritance allows developers to create a base component with shared logic and extend it in multiple derived components. This approach helps in reducing code duplication and ensures that common functionality is centralized.
For example, you can have a BaseComponent that includes common lifecycle hooks, methods, and properties. Derived components can extend BaseComponent, inheriting its functionality and adding specific behaviors or overrides as needed.
Service Inheritance
Similar to component inheritance, service inheritance involves creating a base service with shared logic that can be extended by other services. This is particularly useful for defining common data handling, HTTP requests, or utility functions that multiple services can utilize.
Using inheritance in services helps to maintain consistency and reduce redundancy across your application’s service layer. By extending a base service, derived services inherit common methods and properties, ensuring that shared logic is centralized and easily maintainable.
Benefits of Using Inheritance in Angular
- Code Reusability: Inherits common logic, reducing duplication across components and services.
- Maintainability: Centralizes shared functionality, making it easier to update and manage.
- Scalability: Simplifies extending and enhancing functionalities without modifying existing code.
- Consistency: Ensures that common behaviors are consistent across different parts of the application.
Component Inheritance in Angular
Component inheritance in Angular is a powerful feature that allows developers to create base components with common functionality and then extend these components to create more specific, derived components. This technique promotes code reuse, consistency, and maintainability, making it easier to manage complex applications. In this blog post, we will explore the concept of component inheritance in Angular in detail, including practical examples and code snippets.
Component inheritance refers to the practice of defining a base component with shared logic, methods, and properties, and then extending this base component in other components to reuse the shared functionality. This approach helps in reducing code duplication and centralizing common behaviors, making the application easier to maintain and extend.
Creating a Base Component
Let's start by creating a base component that includes some shared properties and methods.
// base.component.ts
import { OnInit } from '@angular/core';
export class BaseComponent implements OnInit {
sharedProperty: string;
ngOnInit() {
this.sharedProperty = 'Shared Value';
this.sharedMethod();
}
sharedMethod() {
console.log('This is a shared method.');
}
}
In this example, BaseComponent implements the OnInit interface and includes a shared property (sharedProperty) and a shared method (sharedMethod). The ngOnInit lifecycle hook is also defined to initialize the shared property and call the shared method.
Extending the Base Component
Now, let's create a derived component that extends the BaseComponent.
// derived.component.ts
import { Component, OnInit } from '@angular/core';
import { BaseComponent } from './base.component';
@Component({
selector: 'app-derived',
template: '<div>{{ sharedProperty }}</div>',
})
export class DerivedComponent extends BaseComponent implements OnInit {
ngOnInit() {
super.ngOnInit();
console.log('This is the derived component.');
}
}
In this DerivedComponent, we extend the BaseComponent and override the ngOnInit lifecycle hook. We call super.ngOnInit() to ensure that the initialization logic from the base component is executed. The derived component can also add its specific logic.
Using the Derived Component in a Template
You can now use the DerivedComponent in your Angular application as you would with any other component.
<!-- app.component.html -->
<app-derived></app-derived>
When this component is rendered, it will display the shared property value from the base component and execute the shared method.
Advanced Example: Multiple Levels of Inheritance
Component inheritance can be extended to multiple levels. Let's create a more complex example with multiple levels of inheritance.
Intermediate Base Component
// intermediate-base.component.ts
import { OnInit } from '@angular/core';
import { BaseComponent } from './base.component';
export class IntermediateBaseComponent extends BaseComponent implements OnInit {
intermediateProperty: string;
ngOnInit() {
super.ngOnInit();
this.intermediateProperty = 'Intermediate Value';
this.intermediateMethod();
}
intermediateMethod() {
console.log('This is an intermediate method.');
}
}
Final Derived Component
// final-derived.component.ts
import { Component, OnInit } from '@angular/core';
import { IntermediateBaseComponent } from './intermediate-base.component';
@Component({
selector: 'app-final-derived',
template: '<div>{{ sharedProperty }}</div>
<div>{{ intermediateProperty }}</div>',
})
export class FinalDerivedComponent extends IntermediateBaseComponent implements OnInit {
ngOnInit() {
super.ngOnInit();
console.log('This is the final derived component.');
}
}
Handling Input and Output Properties
Inheritance can also be used to handle @Input and @Output properties. Let's extend our example to include these.
Base Component with Input and Output
// base-input-output.component.ts
import { Input, Output, EventEmitter } from '@angular/core';
export class BaseInputOutputComponent {
@Input() baseInput: string;
@Output() baseOutput = new EventEmitter<string>();
emitBaseOutput() {
this.baseOutput.emit('Base Output Emitted');
}
}
Derived Component Handling Input and Output
// derived-input-output.component.ts
import { Component, Input, Output, EventEmitter } from '@angular/core';
import { BaseInputOutputComponent } from './base-input-output.component';
@Component({
selector: 'app-derived-input-output',
template: '<div>{{ baseInput }}</div>
<button (click)="emitBaseOutput()">Emit Output</button>',
})
export class DerivedInputOutputComponent extends BaseInputOutputComponent {
@Input() derivedInput: string;
@Output() derivedOutput = new EventEmitter<string>();
emitDerivedOutput() {
this.derivedOutput.emit('Derived Output Emitted');
}
}
Service Inheritance in Angular
Service inheritance in Angular is a pattern that allows you to create a base service with common functionality and extend it in derived services. This approach promotes code reuse, consistency, and maintainability across your application’s service layer. In this blog post, we will explore the concept of service inheritance in Angular, providing detailed examples and code snippets to illustrate how it can be implemented effectively.
Service inheritance involves defining a base service class with shared logic and extending this base class to create derived services. These derived services inherit the properties and methods of the base service, allowing them to reuse common functionality without duplication. This technique is particularly useful for handling common data operations, HTTP requests, and utility functions that multiple services might need.
Creating a Base Service
Let’s start by creating a base service that includes some shared properties and methods.
// base.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class BaseService {
protected baseUrl: string = 'https://api.example.com';
log(message: string) {
console.log('BaseService: message');
}
fetchData(endpoint: string): string {
// Simulate a fetch operation
return "Data from "this.baseUrl"/endpoint";
}
}
In this example, BaseService includes a protected property (baseUrl) and two methods (log and fetchData). The log method logs a message to the console, and the fetchData method simulates fetching data from an API endpoint.
Extending the Base Service
Now, let’s create a derived service that extends the BaseService.
// derived.service.ts
import { Injectable } from '@angular/core';
import { BaseService } from './base.service';
@Injectable({
providedIn: 'root',
})
export class DerivedService extends BaseService {
getSpecificData() {
const data = this.fetchData('specific-endpoint');
this.log('Fetched specific data');
return data;
}
}
In this DerivedService, we extend the BaseService and add a new method (getSpecificData). This method calls the fetchData method from the base service to get data from a specific endpoint and logs a message using the base service’s log method.
Using the Derived Service in a Component
You can now inject the DerivedService into a component and use it.
// app.component.ts
import { Component, OnInit } from '@angular/core';
import { DerivedService } from './derived.service';
@Component({
selector: 'app-root',
template: '<div>{{ data }}</div>',
})
export class AppComponent implements OnInit {
data: string;
constructor(private derivedService: DerivedService) {}
ngOnInit() {
this.data = this.derivedService.getSpecificData();
}
}
In this AppComponent, we inject the DerivedService and use its getSpecificData method to fetch and display data.
Conclusion
Inheritance in Angular, encompassing both component and service inheritance, offers a robust mechanism for promoting code reuse, consistency, and maintainability in your applications. By creating base classes with shared logic and extending them in derived classes, you can centralize common functionality, reduce code duplication, and streamline your development process.