11th Feb 2022
Integrating Node.js with MongoDB
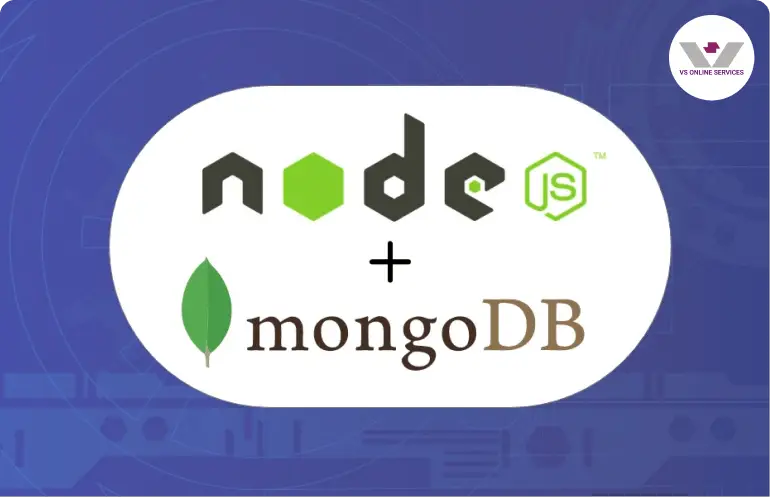
MongoDB is a document database which is referred to as a non-relational database. It indicates that relational data is kept in a unique way. A better way to refer to it is as a non-tabular database. MongoDB stores data in flexible documents. Instead of having multiple tables we can simply keep all of our related data together. This makes reading our data very fast. MongoDB is an open-source document and leading NoSQL database.
Install Node.JS
Node.js® is an open-source, cross-platform JavaScript runtime environment. Download and install the Node from the official website. NodeJS official download. Check whether the node and npm is installed in by running following the command
node -v
npm -v
Create an account in Atlas Mongo
MongoDB Atlas is a Database-as-a-Service platform for MongoDB which allows us to host database.
Click to sign up for a new account.
Create a Cluster
Click the 'Create' button under shared type on the 'Deploy a cloud database' screen after creating and validating the account. This will be the only FREE option.

In the 'Cloud Provider & Region' select the region of your choice. Here we have selected Mumbai(ap-south-1).

In Cluster Tier settings leave as is and for Cluster Name give your desired name, here we have given it as 'Tech'
Then click on Create Cluster at the bottom.
Create User for the Database
For new druggies who have registered, there will be a Quickstart option under security which will help us to configure the stoner credentials.
Under' How would you like to authenticate your connection? select Username and Password. Select 'Autogenerate Secure Password' for the password, and then type in the username you want.

By default, users created will be given 'Read and write to any database' permission.
Creating Cluster
After creating cluster, below it we will have the access option( This will be under Security-> Quickstart only for the first time when an account is created. latterly to modify, you can click on Network Access).
Choose My Local Environment and in the IP Address text box add the IP 0.0.0.0/0 and also click on 'Add Entry'.


Review the below entries and click on 'Finish and Close'. Your cluster is now created.
Accessing Cluster
Click on Database under 'Deployment' and click on Connect. In the modal popup click on Connect your application.

Next select Node.js as the driver, and for version, pick the one that matches the one that is installed on your PC. Click on Copy button to copy the URL. This URL is used to connect the database from our application.

Set up node js with database information created above
>mkdir NodeWithMongo
>cd NodeWithMongo
To initialize Node dependency file with npm init
The npm init command is used to create a Node. js project. The project files will be placed in a package that is created by the npm init command. All the modules you download will be stored in the package
>npm init -y
Create a newfolder and navigate to that folder
Install Dependencies for Your Node.js Project
To install express as dependency for your nodejs project. Express is a back-end web application framework for building RESTful APIs with Node.js, released as free and open-source software
>npm install express
To install mongodb as dependency for your nodejs project. The MongoClient class is a class that allows for making Connections to MongoDB.
>npm install mongodb
Let us produce the index.js file,which generally handles operation incipiency, routing, and other operation functions. Copy paste the below code in your index.js train
const Express = require("express");
const BodyParser = require("body-parser");
const MongoClient = require("mongodb").MongoClient;
const CONNECTION_URL = 'CONNECTION_URL';
const DATABASE_NAME = 'DATABASE_NAME';
var app = Express();
app.use(BodyParser.json());
app.use(BodyParser.text());
app.use(BodyParser.urlencoded({ extended: true }));
var database,collection;
MongoClient.connect(CONNECTION_URL, { useNewUrlParser: true }, (error, client) => {
if (error) {
throw error;
}
database = client.db(DATABASE_NAME);
collection = database.collection("Users");
console.log("Connected is '" + DATABASE_NAME + "'!");
});
app.get("/getAllUser", (request, response) => {
collection.find({}, {
projection: { _id: 0 }
}).toArray((error, result) => {
if (error) {
return response.status(500).send(error);
}
response.send(result);
});
});
app.get("/getUserDetails", (request, response) => {
collection.findOne({ "Email": (request.query.Email) },{projection: { _id: 0 }} ,(error, result) => {
if (result) {
response.send(result);
}
else {
response.send("user not found");
}
});
});
app.post("/addUser", (request, response) => {
collection.insert(request.body, (error, result) => {
if (error) {
return response.status(500).send(error);
}
if(result.insertedCount > 0){
response.send("user added successfully");
}
else{
response.send("user not added");
}
});
});
app.post("/updateUser", (request, response) => {
collection.findOne({ "Email": (request.body.Email) }, (error, result) => {
if (result) {
const query = { Email: result.Email };
const update = { $set: request.body };
const options = { upsert: true };
collection.updateOne(query, update, options);
response.send("values updated successfully");
}
else {
response.send("user not found");
}
});
});
app.delete("/deleteUser", (request, response) => {
var myquery = { Email: request.query.Email};
collection.deleteOne(myquery ,(error, result) => {
if (result.deletedCount > 0) {
response.send("user deleted successfully");
}
else {
response.send("user not found");
}
});
});
app.listen(6000, () => {
console.log('Server is running at port 6000');
});
In the above code replace CONNECTION_URL and DATABASE_NAME with the database name created in atlast mongo.
We have a method to insert('/addUser') and to update('/updateUser') user details via POST request. A GET request('/getAllUser') to retrieve the users added in the database and a DELETE request('/getAllUser') to remove a user.
Now lets run our application by executing the following command
>node index.js
Now we can Postman to hit the API and test our application. Below is our Add User method
>http://localhost/addUser

GET to retrive the added users
>http://localhost/getAllUser

You can also try UPDATE and DELETE methods.
Conclusion
In this tutorial, we have created an account in Atlas Mongo, created a cluster, established a connection with the Atlas and accessed the database from our node application
About Us
- VS Online Services : Custom Software Development
VS Online Services has been providing custom software development for clients across the globe for many years – especially custom ERP, custom CRM, Innovative Real Estate Solution, Trading Solution, Integration Projects, Business Analytics and our own hyperlocal e-commerce platform vBuy.in and vsEcom.
We have worked with multiple customers to offer customized solutions for both technical and no technical companies. We work closely with the stake holders and provide the best possible result with 100% successful completion To learn more about VS Online Services Custom Software Development Solutions or our product vsEcom please visit our SaaS page, Web App Page, Mobile App Page to know about the solution provided. Have an idea or requirement for digital transformation? please write to us at siva@vsonlineservices.com