16th Oct 2023
Integrating Vipps payment in SaaS application
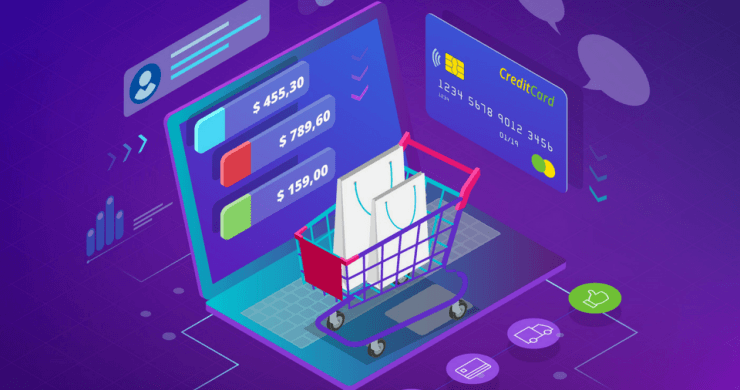
Our customer faced a significant chain in integrating the Vipps payment gateway into their SaaS product. Our dedicated VS Online Services Technical team collaborated closely with the client to successfully enable seamless Vipps payment integration. As the popularity of Vipps continues to rise in Norway, it becomes imperative to grasp the effective methods for integrating this payment solution into your SaaS application. Due to limited available resources, our team has crafted this article to provide a valuable resource for others looking to utilize the same information.
What is Vipps?
Vipps is a mobile payment platform that has gained immense fashionability in Norway. It allows users to make payments, split bills, transfer money, and even pay for goods and services through their smartphones. With its user-friendly interface and widespread adoption, Vipps has become a preferred choice for both businesses and consumers.
How Vipps Differs from Other Payment Integrations
Vipps stands out in several ways compared to other payment integrations:
- Norwegian Focus: Vipps is a Norwegian-born payment solution, which means it's uniquely suited to the Norwegian market. Businesses and customers in Norway choose it with confidence.
- Mobile-Centric: Vipps is designed for mobile devices, making it a convenient choice for customers who prefer to use their smartphones for payments.
- Recurring Payment Flexibility: Vipps offers a dynamic recreating payment result, allowing businesses to produce flexible subscription models, an option not always available with other payment gateways.
- Low Transaction Costs: Vipps' competitive transaction costs make it an attractive option for businesses looking to optimize their payment processing expenses.
Vipps vs. Other Payment Integration Options
Before we dive into the specialized aspects of Vipps integration, let's first explore how Vipps differs from other payment options generally used in SaaS operations.
- Security and Trust: In Norway, Vipps is a estimable payment provider with a strong character for security. For SaaS organisations, this is essential because managing client payments puts security first. Vipps provides features like translated deals and two- factor authentication to give guests and organisations peace of mind.
- Local Market Focus: Vipps caters specifically to the Norwegian request, which can be a significant advantage for SaaS companies operating in Norway. Original guests frequently prefer original payment options, and by integrating Vipps, you can offer a more individualized and accessible payment experience.
- User-Friendly Experience: Vipps is a desirable choice for your SaaS operation because of its stoner-friendly mobile app and speedy payment process.
How to Integrate Vipps Payments
To start benefiting from Vipps payment integration, you'll need to follow a few simple steps
- Register with Vipps: Sign up for a Vipps business account and go through their verification process.
- Choose an Integration Method: Vipps offers colourful integration styles, including API integration, web plugins, and mobile SDKs. elect the bone that stylish suits your business requirements.
- Develop or Configure Your Payment System: Depending on your chosen integration method, you may need to develop or configure your payment system to accept Vipps payments.
- Test and Go Live: Before going live, completely test your Vipps payment integration to insure everything is performing rightly.
One-Time Payment Integration with Vipps (C# Code)
To integrate Vipps payments into your C# operation, you'll need to use the Vipps API. Below is a simplified example of how you can initiate a payment request using C# and the Vipps API. This example demonstrates how to create a Vipps payment request and obtain a payment URL:
public string GeneratePaymentRequest()
{
string clientId = "your_client_id";
string clientSecret = "your_client_secret";
string baseUrl = "https://api.vipps.no";
string paymentRequestEndpoint = "/ecomm/v2/payments";
var paymentRequest = new
{
merchantInfo = new
{
merchantSerialNumber = "your_merchant_serial_number",
},
customerInfo = new
{
mobileNumber = "customer_mobile_number",
},
transaction = new
{
orderId = "your_order_id",
amount = 100, // The payment amount in cents
},
};
string jsonPaymentRequest = Newtonsoft.Json.JsonConvert.SerializeObject(paymentRequest);
using (var client = new HttpClient())
{
client.BaseAddress = new Uri(baseUrl);
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", Convert.ToBase64String(System.Text.Encoding.ASCII.GetBytes($"{clientId}:{clientSecret}"));
HttpResponseMessage response = await client.PostAsync(paymentRequestEndpoint, new StringContent(jsonPaymentRequest, System.Text.Encoding.UTF8, "application/json"));
if (response.IsSuccessStatusCode)
{
string responseContent = await response.Content.ReadAsStringAsync();
Console.WriteLine("Payment request successful.");
return responseContent;
}
else
{
return "Payment request failed.";
}
}
}
Recurring Payment Integration with Vipps (C# Code)
For businesses looking to set up recurring payment options, Vipps provides a solution.
public string CreateSubscription()
{
string clientId = "your_client_id";
string clientSecret = "your_client_secret";
string baseUrl = "https://api.vipps.no";
string subscriptionEndpoint = "/recurring/v1/subscriptions";
var subscriptionRequest = new
{
merchantInfo = new
{
merchantSerialNumber = "your_merchant_serial_number",
},
customerInfo = new
{
mobileNumber = "customer_mobile_number",
},
subscription = new
{
planId = "your_plan_id",
price = 100, // The subscription price in cents
},
};
string jsonSubscriptionRequest = Newtonsoft.Json.JsonConvert.SerializeObject(subscriptionRequest);
using (var client = new HttpClient())
{
client.BaseAddress = new Uri(baseUrl);
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", Convert.ToBase64String(System.Text.Encoding.ASCII.GetBytes($"{clientId}:{clientSecret}"));
HttpResponseMessage response = await client.PostAsync(subscriptionEndpoint, new StringContent(jsonSubscriptionRequest, System.Text.Encoding.UTF8, "application/json"));
if (response.IsSuccessStatusCode)
{
string responseContent = await response.Content.ReadAsStringAsync();
Console.WriteLine("Subscription creation successful.");
return responseContent;
}
else
{
return "Subscription creation failed.";
}
}
}
Dynamic Recurring Payment Integration with Vipps (C# Code)
Dynamic Recreating payments allow your SaaS operation to bill guests different quantities grounded on operation or variable subscription plans. To enable dynamic recurring payments with Vipps, you can follow these steps:
- User Approval: Before enabling dynamic recurring payments, you can use Vipps to send a small transaction (e.g., NOK 20 to 30) to the user's account. The user will receive a notification asking for approval. If the user agrees, you can proceed with dynamic recurring payments.
- API Integration: Integrate Vipps' API into your SaaS operation to manage dynamic recreating payments. The API will handle the billing and keep track of varying payment quantities.
- Customization: Customize your application to calculate the dynamic amounts based on usage or subscription changes. Insure that your operation communicates effectively with Vipps to initiate the payments.
Dynamic recurring payments allow customers to set up recurring payments for varying amounts and schedules.
public string CreateDynamicRecurring()
{
string clientId = "your_client_id";
string clientSecret = "your_client_secret";
string baseUrl = "https://api.vipps.no";
string paymentLinkEndpoint = "/ecomm/v2/payments";
var dynamicRecurringRequest = new
{
merchantInfo = new
{
merchantSerialNumber = "your_merchant_serial_number",
},
transaction = new
{
orderId = "your_order_id",
amount = 0, // The initial payment amount (can be zero for dynamic recurring),
operation = "INITIATE_PAYMENT",
recurring = new
{
frequency = "WEEKLY", // Set the desired payment frequency
interval = 1, // Set the interval
endDate = "yyyy-MM-ddTHH:mm:ssZ", // Set the end date for recurring payments
},
},
};
string jsonDynamicRecurringRequest = Newtonsoft.Json.JsonConvert.SerializeObject(dynamicRecurringRequest);
using (var client = new HttpClient())
{
client.BaseAddress = new Uri(baseUrl);
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", Convert.ToBase64String(System.Text.Encoding.ASCII.GetBytes($"{clientId}:{clientSecret}"));
HttpResponseMessage response = await client.PostAsync(paymentLinkEndpoint, new StringContent(jsonDynamicRecurringRequest, System.Text.Encoding.UTF8, "application/json"));
if (response.IsSuccessStatusCode)
{
string responseContent = await response.Content.ReadAsStringAsync();
Console.WriteLine("Dynamic recurring payment link created successfully.");
return responseContent;
}
else
{
return "Dynamic recurring payment link creation failed.";
}
}
}
Challenges in Dynamic Recurring Payments
While dynamic recurring payments offer flexibility, there are challenges to consider:
- User Approval: Not all users may approve the initial small transaction, preventing you from enabling dynamic recurring payments for them. You may need to implement alternative billing methods for these users.
- Billing Accuracy: Icing the delicacy of billing for dynamic recreating payments can be complex. You must maintain precise records of usage and subscription changes to avoid billing errors.
- Communication: Effective communication between your SaaS operation and Vipps is pivotal. Any breakdown in communication can lead to issues with billing and user experience.
After receiving the response, you can save the response and extract the paymentLink to send to the user. When the user clicks the link in a web browser, they will be prompted to enter their credentials and proceed with the payment. However, if the user clicks the link on a mobile device, the behavior will depend on whether the Vipps app is installed:
- Vipps App Installed: If the Vipps app is installed on the user's mobile device, clicking the link will trigger the app to open. The stoner will be notified within the Vipps app, and they can give their credentials within the app to do with the payment.
- Vipps App Not Installed: However, clicking the link will affect in redirection to a web cybersurfer, If the Vipps app isn't installed on the stoner's mobile device. In the web browser, the user will be prompted to enter their credentials and complete the payment.
This approach ensures a smooth and flexible payment experience for druggies, allowing them to choose between the Vipps app and a web cybersurfer grounded on their preferences and device capabilities.
Conclusion
In conclusion, integrating Vipps into your SaaS operation provides a secure and stoner-friendly payment result, particularly in the Norwegian request. By understanding how to set up dynamic recurring payments and addressing potential challenges, you can offer a seamless payment experience to your customers while ensuring accurate billing.