01th Jun 2023
Implementing Logging in .Net with Serilog, NLog, Log4Net
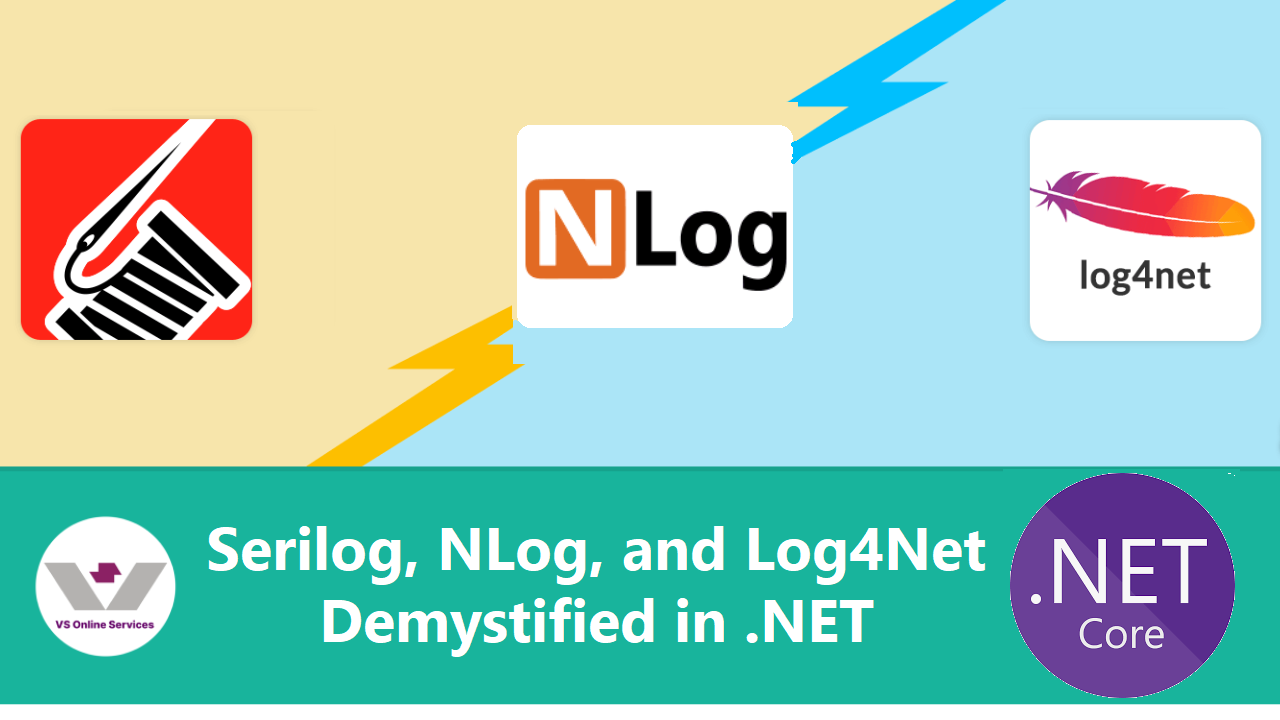
Overview of Serilog
In ultramodern software development, logging plays a pivotal part in maintaining and troubleshooting operations. It allows inventors and system directors to gain precious perceptivity into the geste of an operation, track down bugs, and cover performance. When it comes to logging in ASP.NET Core there are several tools available each with its own pros and cons let’s first see an overview of the three prominent logging tools used in .Net followed by how to implement each of the them in your .Net application
Serilog
Serilog is a newer logging framework that aims to provide a more flexible and structured approach to logging.Serilog emphasizes the conception of structured logging, where log events correspond of crucial- value dyads, making it easier to query and assay logs. It supports colorful cesspools for affair, similar as press, train, database, and third- party services.
NLog
NLog provides a largely flexible and important configuration system that allows you to configure logging geste through XML, JSON, or law- grounded configurations. It offers fine- granulated control over log situations, targets, pollutants, and formatting options, making it suitable for complex logging scripts. This inflexibility can be salutary when dealing with large or different operations.
Log4Net
Log4net follows the logging stylish practices and supports colorful log situations, including debug, word, advise, error, and fatal. It offers multiple logging appenders, such as console, file, database, and remote logging, enabling developers to choose the appropriate destination for log messages.Log4net supports configuration through XML or law, furnishing inflexibility in customizing logging geste . also, it offers features like log filtering, log formatting, and log scale, allowing for fine- granulated control over log affair.
let’s see how to implement Serilog in your .net application.
Implement Serilog
Step 1: Install the Serilog NuGet package
In Visual Studio, right-click on your project in the Solution Explorer.
Select "Manage NuGet Packages".
Search for "Serilog" and click on "Install" to add the package to your project.
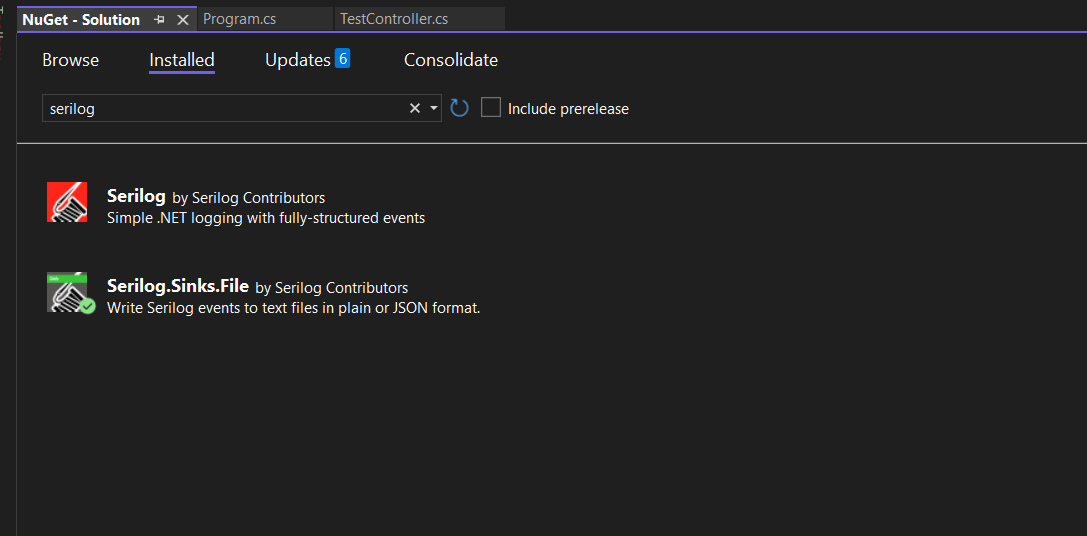
Step 2: Configure Serilog
In your code, add the following using statement
using Serilog;
Configure Serilog in your application, typically in the Main method or in the application Program.cs:
Log.Logger = new LoggerConfiguration().MinimumLevel.Debug().WriteTo.File("logs/log-.logs",
rollingInterval: RollingInterval.Day).CreateLogger();
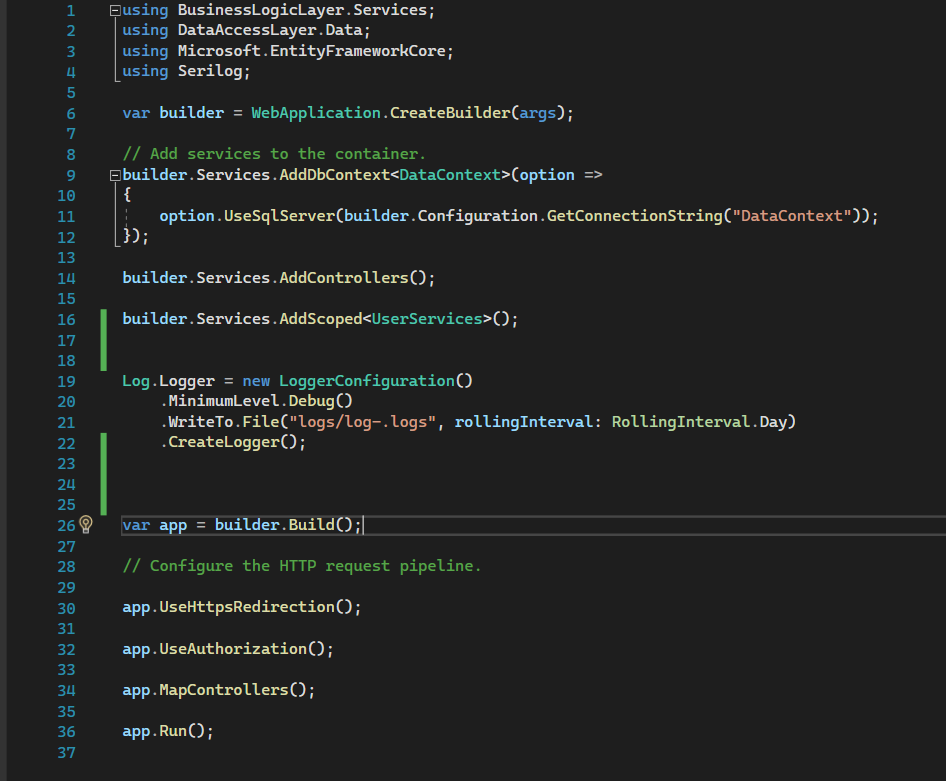
Step 3: Use Serilog to log events
Throughout your code, you can use Serilog to log events at different levels (e.g., Information, Warning, Error):
Log.Debug("Hello");
Log.Error("Error");
Log.Information("Information");
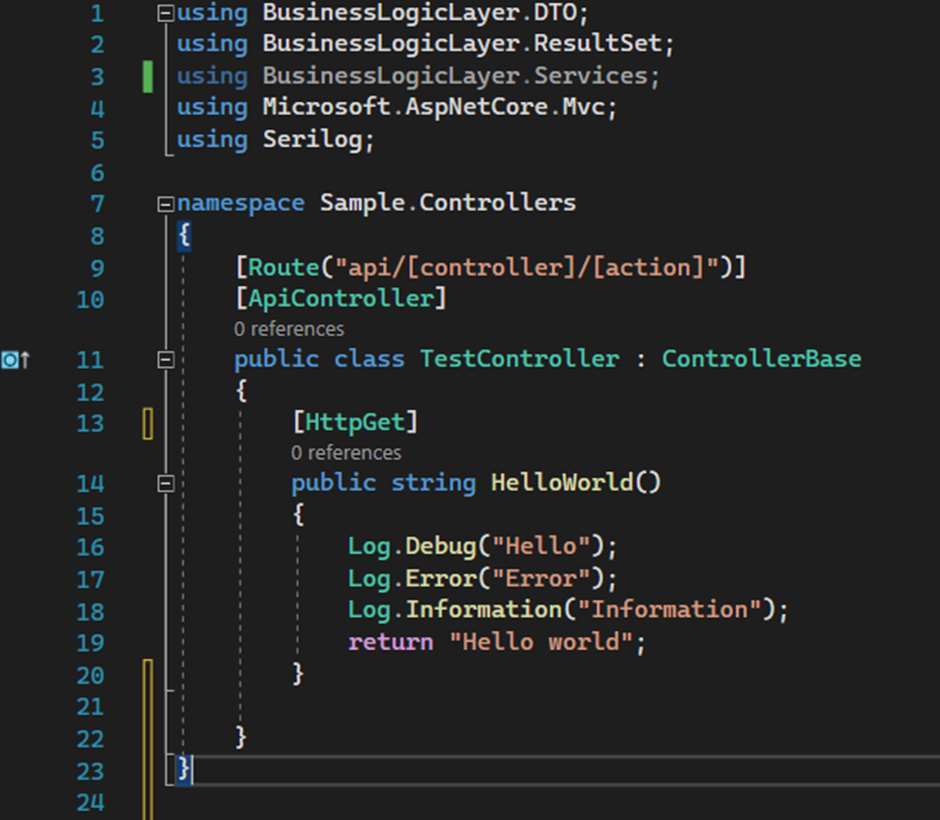
You can also include additional properties with your log events:
Log.Information("User {UserId} logged in", userId);
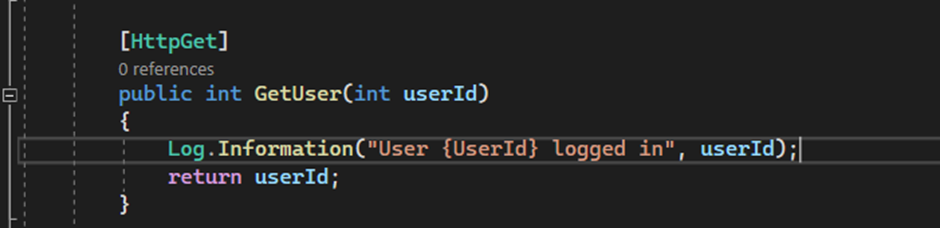
Step 4: Dispose Serilog logger
It's a good practice to dispose of the Serilog logger when your application is shutting down:
Log.CloseAndFlush();
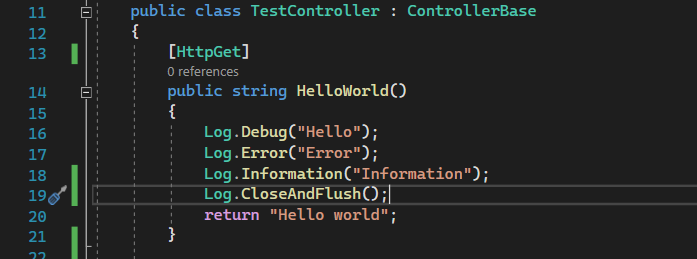
Step 5: View the log output
your operation runs, you'll see log dispatches in the configured labors(e.g., press, train).
your operation runs, you'll see log dispatches in the configured labors(e.g., press, train).
Implementing NLog
Step 1: Installing reliance using nuget package director
To install the needed dependencies for NLog in yourASP.NET Core operation, you can use NuGet by executing the following commands.
Install-Package NLog
Install-Package NLog.Web.AspNetCore
Step 2: Creating NLog Configuration file
The NLog configuration file is an XML file that contains the settings related to NLog. This train must be named in lower- case, and you can place it in the root of your design.
There are two main rudiments needed by every configuration targets and rules. It may also have other rudiments similar as extensions, include, and variables. But those are voluntary which can be useful in certain scripts.
The targets element defines a log target that's defined by a target element. There are two main attributes name( name of the target) and type( target type similar as- train, database,etc.).
<?xml version="1.0" encoding="utf-8" ?>
<nlog xmlns="http://www.nlog-project.org/schemas/NLog.xsd" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" autoReload="true" internalLogLevel="info" internalLogFile="internalLog.txt">
<extensions>
<add assembly="NLog.Web.AspNetCore" />
</extensions>
<!-- the targets to write to -->
<targets>
<!-- write to file -->
<target xsi:type="File" name="alldata" fileName="demo-$shortdate}.log" layout="$longdate}|$event-properties:item=EventId_Id}|$uppercase:$level}}|$logger}|$message} $exception:format=tostring}" />
<!-- another file log. Uses some ASP.NET core renderers -->
<target xsi:type="File" name="otherFile-web" fileName="demo-Other-$shortdate}.log" layout="$longdate}|$event-properties:item=EventId_Id}|$uppercase:$level}}|$logger}|$message} $exception:format=tostring}|url: $aspnet-request-url}|action: $aspnet-mvc-action}" />
</targets>
<!-- rules to map from logger name to target -->
<rules>
<logger name="*" minlevel="Trace" writeTo="alldata" />
<!--Skip non-critical Microsoft logs and so log only own logs-->
<logger name="Microsoft.*" maxLevel="Info" final="true" />
<logger name="*" minlevel="Trace" writeTo="otherFile-web" />
</rules>
</nlog>
To ensure that the "nlog.config" file is available in the same location as the project's DLL, you need to perform the following steps:
- Right-click on the "nlog.config" file within your project
- From the context menu, choose "Properties".
- In the properties window,Find the "Copy to Output Directory" property in the properties pane.
- Set the value of this property to "Copy always".
By doing this, the"nlog.config" train will be automatically copied to the affair directory whenever the design is erected or executed. This ensures that the configuration train is available in the applicable position alongside the design's DLL.
Step 3: Configure NLog in the application
Now you can configure the NLog in your application as follows,
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
_logger.LogInformation("HomeController.Index method called!!!");
return View();
}
}
Implementing Log4Net
Step 1: Install the log4net package
Open the NuGet Package Manager in Visual Studio.
Look up "log4net" online and choose the log4net package.
To incorporate the log4net package into your project, click the "Install" button.
Step 2: Add log4net configuration file
Create a new XML configuration file in your project (e.g., "log4net.config").
Set the "Build Action" property of the file to "Content" and the "Copy to Output Directory" property to "Copy if newer".
Add the following content to the configuration file:
//code
<log4net>
<appender name="ConsoleAppender" type="log4net.Appender.ConsoleAppender">
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date [%thread] %-5level %logger - %message%newline" />
</layout>
</appender>
<root>
<level value="DEBUG" />
<appender-ref ref="ConsoleAppender" />
</root>
</log4net>
Step 3: Configure log4net in your code
At the entry point of your application (e.g., Main method), add the following code to configure log4net:
using log4net;
using log4net.Config;
namespace YourNamespace
{
class Program
{
private static readonly ILog log = LogManager.GetLogger(typeof(Program));
static void Main(string[] args)
{
XmlConfigurator.Configure(new System.IO.FileInfo("log4net.config"));
// ...
log.Debug("This is a debug message");
log.Info("This is an info message");
log.Warn("This is a warning message");
log.Error("This is an error message");
log.Fatal("This is a fatal error message");
// ...
}
}
}
Step 4: Use log4net in your application
In any class where you want to log messages, add the following line to create a logger instance:
private static readonly ILog log = LogManager.GetLogger(typeof(YourClassName));
Then, you can use the logger to log messages at different levels, such as:
//code
public string Helloworld()
{
log.Debug("This is a debug message");
log.Info("This is an info message");
log.Warn("This is a warning message");
log.Error("This is an error message");
log.Fatal("This is a fatal error message");
}
Step 5: Run your application
Build and run your application. You should see the log dispatches published to the press according to the configured log4net settings.
Note Make sure that the log4net.config train is present in the affair directory along with your executable train.
Serilog vs log4net
- Flexibility and Extensibility: Serilog provides a highly extensible and flexible configuration API, allowing customization of log sinks, formatters, and enrichers. on the other hand log4net offers extensibility but with a more limited API compared to Serilog.
- Performance: Serilog is known for its high performance and low memory footmark, thanks to its asynchronous loggingcapability.whereas log4net is generally slower than Serilog due to its coetaneous logging nature.
- Configuration: Serilog uses a law- grounded configuration approach, furnishing inflexibility and allowing complex logging setups and log4net generally uses XML- grounded configuration, which can be simpler for introductory logging setups.
- Documentation and Community Support: Serilog has comprehensive attestation, an active community, and a wide range of plugins and integrations available while log4net has a large stoner base, Plenitude of community coffers, but its sanctioned attestation might be lower up- to- date.
- Maintenance and Updates: Serilog is laboriously maintained and constantly streamlined, serving from ongoing development and advancements But log4net's development has braked down over the times, with lower frequent updates and bug fixes compared to Serilog.
Conclusion
Finally, logging in to a.NET application can be effectively implemented using Serilog, NLog, and log4net. Each framework offers powerful features, flexible possibilities for customisation and a smooth connection with different logging sinks. Whether you prioritize Whether it's ease of use, efficiency, or expandability, these logging libraries offer reliable answers for capturing and analyzing application logs in the .NET ecosystem.