13th June 2024
A Deep Dive into Map, Filter, Reduce, and NgModel
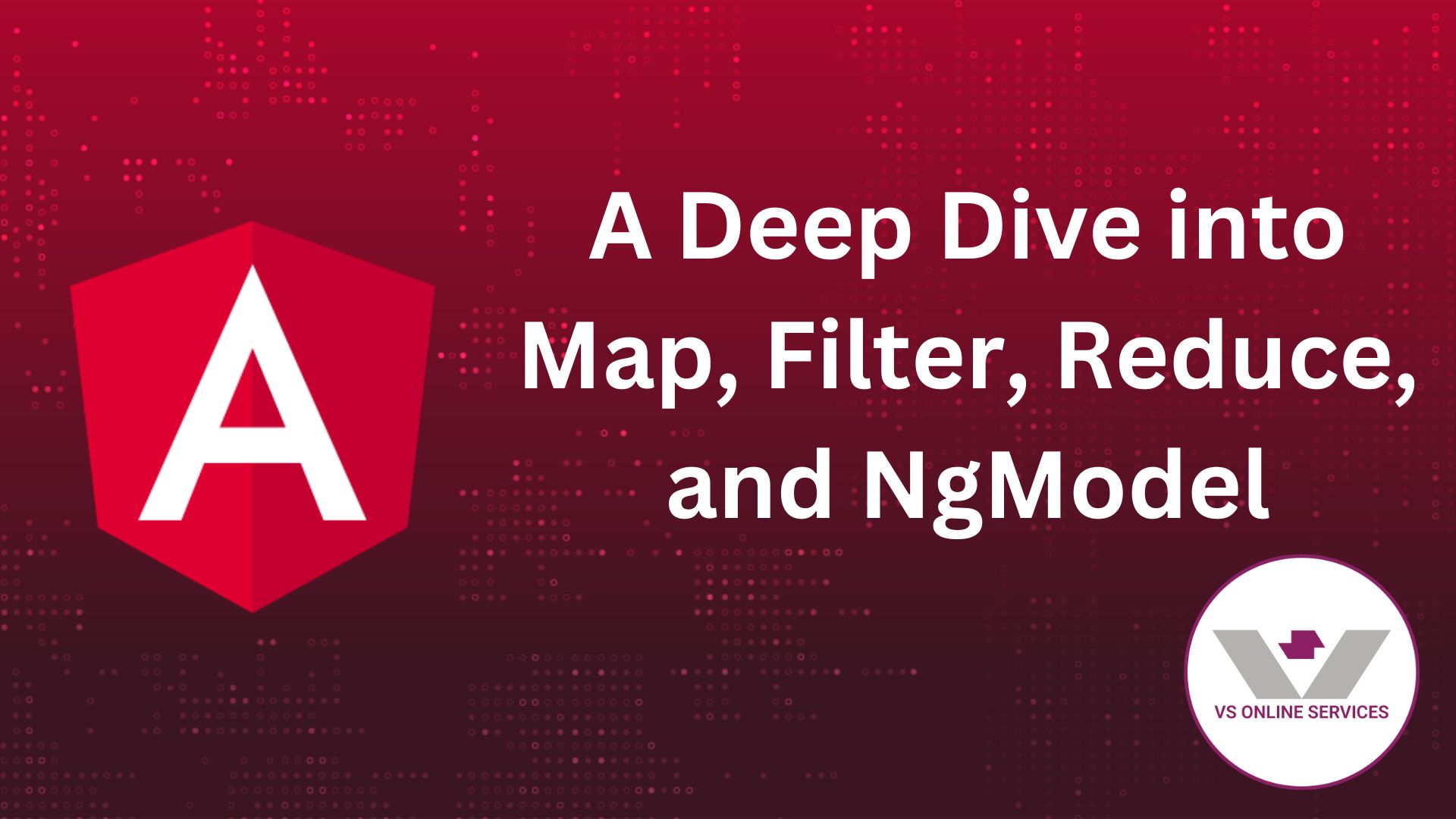
Introduction
In this blog, we'll explore the power and versatility of JavaScript's functional programming tools—map, filter, and reduce—and how they can transform your data manipulation tasks. Additionally, we'll delve into NgModel, a cornerstone of Angular's two-way data binding, to streamline the synchronization of data between your application and the UI.
The map Function
The map function is a built-in method for arrays in JavaScript. It's a powerful tool for iterating over an array, applying a transformation to each element, and creating a new array with the transformed elements.
Key Points
- map does not modify the original array. It creates a new array with the transformed elements.
- map skips empty slots in sparse arrays (arrays with holes). It doesn't call the callback function for those positions.
- You can use arrow functions for concise callback functions
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(function(number) {
return number * 2;
});
console.log(numbers); // [1, 2, 3, 4, 5] (original array remains unchanged)
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
What is map?
The map operator applies a given function to each value emitted by the source Observable, transforming them into new values. This is particularly useful in scenarios where you need to manipulate or format the data received from an HTTP request or any other asynchronous operation before further processing.
Syntax:
import { map } from 'rxjs/operators';
sourceObservable.pipe(
map(value => transformedValue)
);
Example Use Case
Suppose you have an Angular service that fetches user data from an API, and you want to transform the response to include only specific fields.
Service:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { map } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class UserService {
private apiUrl = 'https://api.example.com/users';
constructor(private http: HttpClient) {}
getUsers(): Observable<any> {
return this.http.get<any[]>(this.apiUrl).pipe(
map(users => users.map(user => ({
id: user.id,
name: user.name,
email: user.email
})))
);
}
}
In this example, the getUsers method fetches a list of users from an API and transforms each user object to include only the id, name, and email fields using the map operator.
How to Use in a Component
Component:
import { Component, OnInit } from '@angular/core';
import { UserService } from './user.service';
@Component({
selector: 'app-user-list',
template: '
<ul>
<li *ngFor="let user of users">
{{ user.name }} ({{ user.email }})
</li>
</ul>
'
})
export class UserListComponent implements OnInit {
users: any[] = [];
constructor(private userService: UserService) {}
ngOnInit(): void {
this.userService.getUsers().subscribe(data => {
this.users = data;
});
}
}
Explanation
1. Service Method (getUsers):
- Makes an HTTP GET request to fetch user data.
- Uses the map operator to transform the response by mapping each user object to a new object with only the desired properties.
2. Component (UserListComponent):
- Calls the getUsers method of the UserService in the ngOnInit lifecycle hook.
- Subscribes to the Observable returned by getUsers and assigns the transformed user data to the users array.
Benefits of Using map
- Data Transformation: Easily transform data before it reaches the component.
- Code Clarity: Keeps the transformation logic within the service, maintaining a clear separation of concerns.
- Reusability: The transformation logic can be reused across different components.
What is filter?
The filter operator filters items emitted by the source Observable by only emitting those that satisfy a specified predicate function. This is especially useful when you need to exclude unwanted data or handle only specific cases.
The filter function in JavaScript is another essential tool for array manipulation, working alongside map for transforming and selecting elements. Here's a breakdown of filter:
Purpose
- Creates a new array containing only elements from the original array that pass a test implemented by a provided function.
- Elements that don't meet the test criteria are excluded from the new array.
Key Points
- filter does not modify the original array. It creates a new array with the filtered elements.
- filter skips empty slots in sparse arrays. It doesn't call the callback function for those positions.
- You can use arrow functions for concise callback functions.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(function(number) {
return number % 2 === 0;
});
console.log(numbers); // [1, 2, 3, 4, 5] (original array remains unchanged)
console.log(evenNumbers); // [2, 4]
Syntax
import { filter } from 'rxjs/operators';
sourceObservable.pipe(
filter(value => predicate(value))
);
Example Use Case
Suppose you have an Angular service that fetches a list of products from an API, and you only want to work with products that are in stock.
Service:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { filter, map } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class ProductService {
private apiUrl = 'https://api.example.com/products';
constructor(private http: HttpClient) {}
getInStockProducts(): Observable<any> {
return this.http.get<any[]>(this.apiUrl).pipe(
map(products => products.filter(product => product.inStock))
);
}
}
In this example, the getInStockProducts method fetches a list of products from an API and uses the filter operator to include only the products that are in stock.
How to Use in a Component
Component:
import { Component, OnInit } from '@angular/core';
import { ProductService } from './product.service';
@Component({
selector: 'app-product-list',
template: '
<ul>
<li *ngFor="let product of products">
{{ product.name }} - $"{{ product.price }}"
</li>
</ul>
'
})
export class ProductListComponent implements OnInit {
products: any[] = [];
constructor(private productService: ProductService) {}
ngOnInit(): void {
this.productService.getInStockProducts().subscribe(data => {
this.products = data;
});
}
}
Explanation
1. Service Method (getInStockProducts):
- Makes an HTTP GET request to fetch product data.
- Uses the filter operator to emit only the products that are in stock.
- The map operator is used here to apply the filter method on the array of products.
2. Component (ProductListComponent):
- Calls the getInStockProducts method of the ProductService in the ngOnInit lifecycle hook.
- Subscribes to the Observable returned by getInStockProducts and assigns the filtered product data to the products array.
Benefits of Using filter
- Data Selectivity: Efficiently handle only the data that meets specific conditions.
- Code Clarity: Maintain cleaner and more readable code by separating filtering logic.
- Performance: Reduce the processing overhead by working only with relevant data.
What is reduce?
The reduce function in JavaScript is a versatile tool for iterating over an array and accumulating a single value based on a provided function. Here's a comprehensive explanation:
- Applies a function (called a reducer) against an accumulator and each element in an array to reduce it to a single value.
- Useful for combining elements, finding totals, creating objects, or performing custom aggregations.
Key Points
- reduce can be used with or without an initial value. If not provided, the first element in the array becomes the initial accumulator, and the reducer function starts from the second element.
- reduce does not modify the original array. It creates a single value as the result.
- reduce skips empty slots in sparse arrays. It doesn't call the reducer function for those positions.
- You can use arrow functions for concise reducer functions.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce(function(accumulator, number) {
return accumulator + number;
}, 0); // Initial value (optional)
console.log(sum); // 15
The reduce operator applies an accumulator function to each value emitted by the source Observable, and emits the accumulated result when the source completes. This operator is useful for aggregating data into a single result, such as calculating totals or summarizing information.
Syntax
import { reduce } from 'rxjs/operators';
sourceObservable.pipe(
reduce((accumulator, currentValue) => accumulatorFunction(accumulator, currentValue), initialValue)
);
Example Use Case
Suppose you have an Angular service that fetches sales data from an API, and you want to calculate the total sales amount.
Service:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { reduce } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class SalesService {
private apiUrl = 'https://api.example.com/sales';
constructor(private http: HttpClient) {}
getTotalSales(): Observable<number> {
return this.http.get<{ amount: number }[]>(this.apiUrl).pipe(
reduce((total, sale) => total + sale.amount, 0)
);
}
}
In this example, the getTotalSales method fetches an array of sales objects from an API and uses the reduce operator to calculate the total sales amount.
How to Use in a Component
Component:
import { Component, OnInit } from '@angular/core';
import { SalesService } from './sales.service';
@Component({
selector: 'app-sales-total',
template: '
<p>Total Sales: $"{{ totalSales }}"</p>
'
})
export class SalesTotalComponent implements OnInit {
totalSales: number = 0;
constructor(private salesService: SalesService) {}
ngOnInit(): void {
this.salesService.getTotalSales().subscribe(total => {
this.totalSales = total;
});
}
}
Explanation
1. Service Method (getTotalSales):
- Makes an HTTP GET request to fetch sales data.
- Uses the reduce operator to accumulate the total sales amount.
- The accumulator function takes the current total and adds the amount of each sale to it, starting from an initial value of 0.
2. Component (SalesTotalComponent):
- Calls the getTotalSales method of the SalesService in the ngOnInit lifecycle hook.
- Subscribes to the Observable returned by getTotalSales and assigns the total sales amount to the totalSales variable.
Benefits of Using reduce
- Aggregation: Easily aggregate data into a single result.
- Simplification: Simplify complex data processing tasks by using a clear and concise accumulator function.
- Code Clarity: Maintain clean and readable code by encapsulating aggregation logic.
What is NgModel?
NgModel is part of Angular's FormsModule and is used to bind form controls to properties on the component. It allows for dynamic and interactive forms, enabling seamless communication between the template and the component class.
Setting Up NgModel
To use NgModel, you must import FormsModule into your Angular module.
Example:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, FormsModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Basic Usage
HTML:
<input [(ngModel)]="name" placeholder="Enter your name">
<p>Hello, {{ name }}!</p>
TypeScript:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '
<input [(ngModel)]="name" placeholder="Enter your name">
<p>Hello, {{ name }}!</p>
'
})
export class AppComponent {
name: string = '';
}
In this example, the input field is bound to the name variable in the component. Any change in the input field updates the name variable, and any change in the name variable updates the input field.
Advanced Usage
Binding to Objects
NgModel can also bind form controls to object properties.
HTML:
<input [(ngModel)]="user.name" placeholder="Enter your name">
<input [(ngModel)]="user.email" placeholder="Enter your email">
<p>{{ user | json }}</p>
TypeScript:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '
<input [(ngModel)]="user.name" placeholder="Enter your name">
<input [(ngModel)]="user.email" placeholder="Enter your email">
<p>{{ user | json }}</p>
'
})
export class AppComponent {
user = {
name: '',
email: ''
};
}
Handling ngModelChange
To perform additional actions whenever the model changes, you can use the ngModelChange event.
HTML:
<input [(ngModel)]="name" (ngModelChange)="onNameChange($event)" placeholder="Enter your name">
TypeScript:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '
<input [(ngModel)]="name" (ngModelChange)="onNameChange($event)" placeholder="Enter your name">
'
})
export class AppComponent {
name: string = '';
onNameChange(newValue: string) {
console.log('Name changed to:', newValue);
}
}
Benefits of Using NgModel
- Two-Way Data Binding: Automatically synchronizes the data between the component and the view.
- Code Simplicity: Reduces boilerplate code by managing form control updates automatically.
- Dynamic Forms: Facilitates the creation of interactive and responsive forms.
Conclusion
Understanding and effectively utilizing functional programming techniques such as map, filter, and reduce can significantly enhance your ability to write clean, efficient, and readable code in JavaScript. These functions allow for elegant solutions to complex data manipulation tasks, reducing the need for verbose looping constructs and enhancing code maintainability. By mastering these tools, you not only improve your coding efficiency but also embrace a more declarative style of programming that is easier to understand and debug.
In parallel, Angular's ngModel directive offers a powerful way to create dynamic, two-way data binding in your applications. This capability is central to developing interactive and user-friendly interfaces. By linking the view and model seamlessly, ngModel simplifies the synchronization of data between the UI and the underlying data model, which is particularly beneficial in forms and input-heavy applications.