06th June 2024
Understanding Mouse Events in JavaScript: A Practical Guide
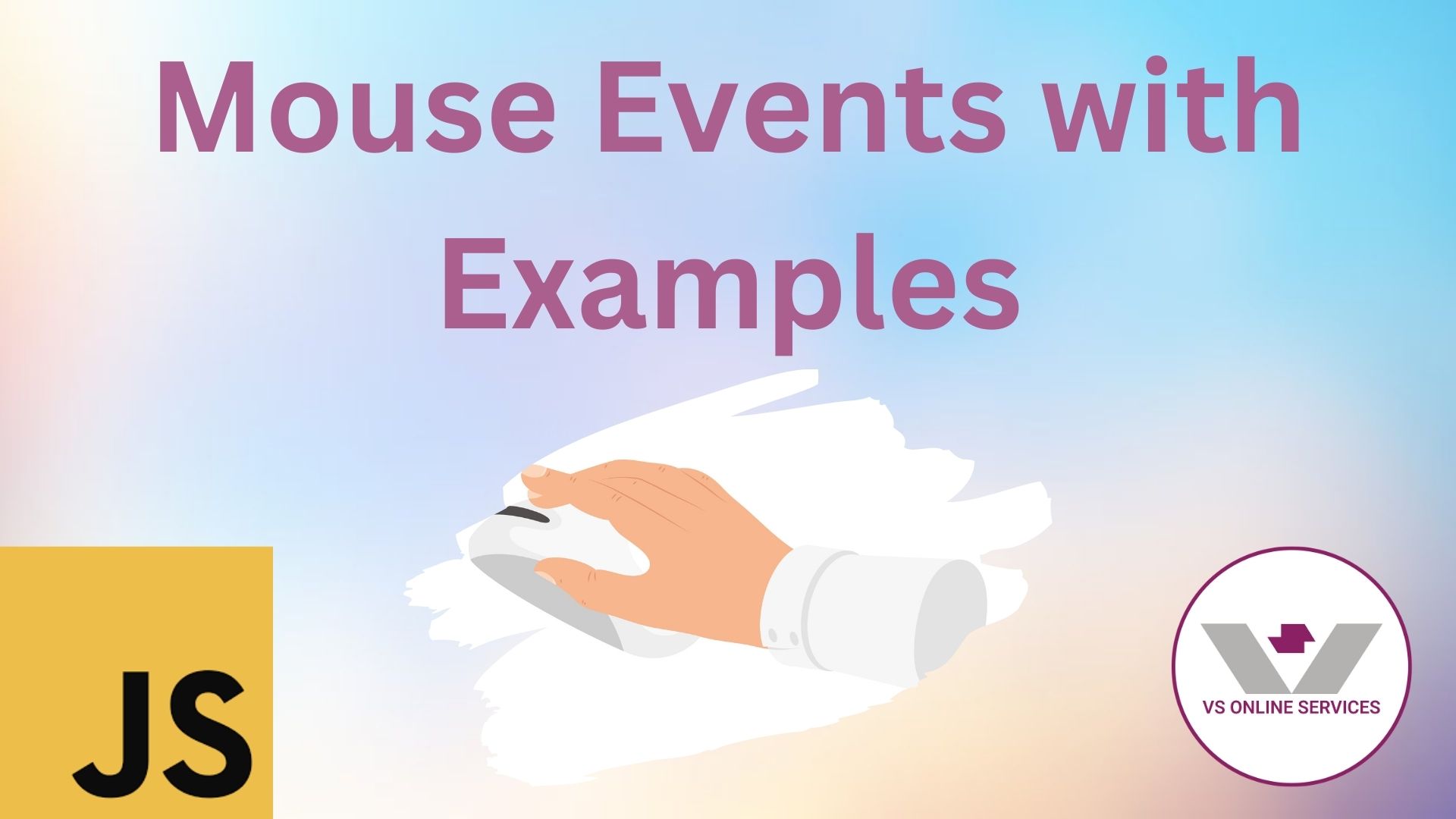
Mouse events are fundamental to creating interactive web applications. They enable developers to respond to user actions like clicking, hovering, or scrolling. In this blog, we'll explore some of the most used mouse events in software development. We'll provide live examples for each event to demonstrate how they work in practice.
Project Setup
We'll maintain a separate JavaScript file (mouseEvents.js) and implement each mouse event as a function. Below is the initial setup with the necessary HTML and JavaScript structure.

index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Mouse Events</title>
<link rel="stylesheet" href="styles.css">
<script defer src="mouseEvents.js"></script>
</head>
<body>
<h1>Mouse Events Examples</h1>
<button id="clickBtn">Click Me</button>
<div id="dblclickDiv" class="box">
Double Click Me
</div>
<p id="mousedownPara">Press down the mouse button on this text.</p>
<p id="mouseupPara">Release the mouse button over this text.</p>
<h1 id="mouseoverHeading">Mouse over this heading</h1>
<h1 id="mouseoutHeading">Mouse out of this heading</h1>
<div id="mousemoveDiv" class="box">
Move the mouse in this box
</div>
<p id="coordinates"></p>
<div id="contextMenuDiv" class="box">
Right Click in this box
</div>
<p id="contextMessage"></p>
<img id="zoomImage" src="image.jpg" alt="Zoomable Image" class="zoomable-image">
</body>
</html>
Make sure you have an image with image.jpg file name in the project folder.
styles.css:
body {
font-family: Arial, sans-serif;
padding: 20px;
}
.box {
width: 200px;
height: 100px;
margin: 10px 0;
padding: 10px;
background-color: lightgray;
border: 1px solid #ccc;
display: flex;
align-items: center;
justify-content: center;
}
.zoomable-image {
width: 200px;
transition: width 0.3s ease;
}
mouseEvents.js:
// mouseEvents.js
// Click Event
document.getElementById('clickBtn').addEventListener('click', handleClick);
function handleClick() {
alert('Button Clicked!');
}
// Double Click Event
document.getElementById('dblclickDiv').addEventListener('dblclick', handleDoubleClick);
function handleDoubleClick() {
this.style.backgroundColor = 'lightcoral';
}
// Mouse Down Event
document.getElementById('mousedownPara').addEventListener('mousedown', handleMouseDown);
function handleMouseDown() {
console.log('Mouse button pressed down.');
}
// Mouse Up Event
document.getElementById('mouseupPara').addEventListener('mouseup', handleMouseUp);
function handleMouseUp() {
console.log('Mouse button released.');
}
// Mouse Over Event
document.getElementById('mouseoverHeading').addEventListener('mouseover', handleMouseOver);
function handleMouseOver() {
this.style.color = 'blue';
}
// Mouse Out Event
document.getElementById('mouseoutHeading').addEventListener('mouseout', handleMouseOut);
function handleMouseOut() {
this.style.color = 'black';
}
// Mouse Move Event
document.getElementById('mousemoveDiv').addEventListener('mousemove', handleMouseMove);
function handleMouseMove(event) {
const coordinates = 'X: event.clientX, Y: event.clientY';
document.getElementById('coordinates').textContent = coordinates;
}
// Context Menu Event
document.getElementById('contextMenuDiv').addEventListener('contextmenu', handleContextMenu);
function handleContextMenu(event) {
event.preventDefault();
document.getElementById('contextMessage').textContent = 'Custom context menu action.';
}
// Wheel Event
document.getElementById('zoomImage').addEventListener('wheel', handleWheel);
function handleWheel(event) {
event.preventDefault();
const scale = event.deltaY < 0 ? 1.1 : 0.9;
this.style.width = 'this.clientWidth * scalepx';
}
When you open index.html in the browser you can see the elements being rendered on the page as shown in the image. We can perform the mouse actions on the elements.
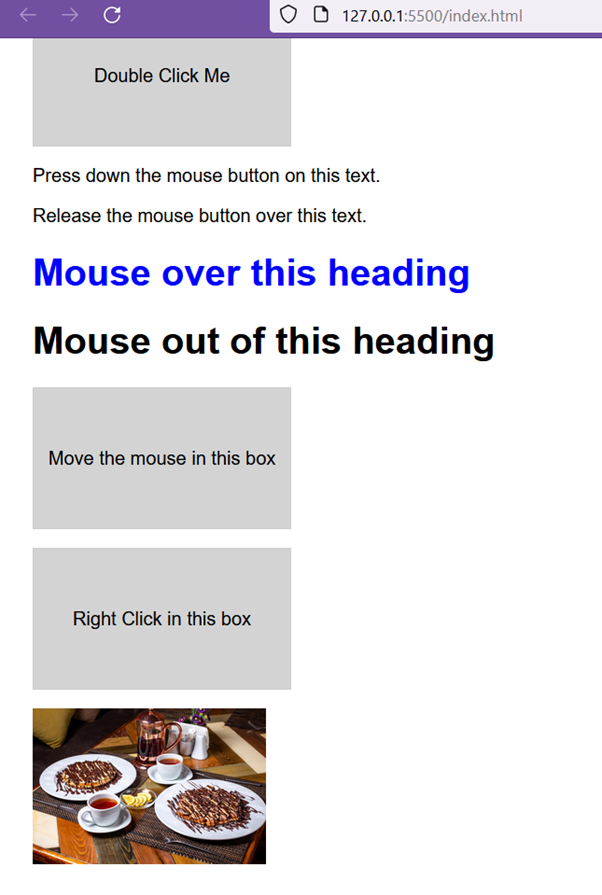
Detailed Explanation and Use Cases
1. Click Event
The click event is triggered when the user clicks on an element.
- Use Case: Display an alert message when a button is clicked.
document.getElementById('clickBtn').addEventListener('click', handleClick);
function handleClick() {
alert('Button Clicked!');
}
2. Double Click Event
The dblclick event is triggered when the user double-clicks on an element.
- Use Case: Change the background color of a div when it is double-clicked.
document.getElementById('dblclickDiv').addEventListener('dblclick', handleDoubleClick);
function handleDoubleClick() {
this.style.backgroundColor = 'lightcoral';
}
3. Mouse Down Event
The mousedown event is triggered when the mouse button is pressed down on an element.
- Use Case: Log a message when the mouse button is pressed down on a paragraph.
document.getElementById('mousedownPara').addEventListener('mousedown', handleMouseDown);
function handleMouseDown() {
console.log('Mouse button pressed down.');
}
4. Mouse Up Event
The mouseup event is triggered when the mouse button is released over an element.
- Use Case: Log a message when the mouse button is released over a paragraph.
document.getElementById('mouseupPara').addEventListener('mouseup', handleMouseUp);
function handleMouseUp() {
console.log('Mouse button released.');
}
5. Mouse Over Event
The mouseover event is triggered when the mouse pointer is moved onto an element.
- Use Case: Change the text color when the mouse pointer is over a heading.
document.getElementById('mouseoverHeading').addEventListener('mouseover', handleMouseOver);
function handleMouseOver() {
this.style.color = 'blue';
}
6. Mouse Out Event
The mouseout event is triggered when the mouse pointer is moved out of an element.
- Use Case: Revert the text color when the mouse pointer leaves a heading.
document.getElementById('mouseoutHeading').addEventListener('mouseout', handleMouseOut);
function handleMouseOut() {
this.style.color = 'black';
}
7. Mouse Move Event
The mousemove event is triggered when the mouse pointer is moved within an element.
- Use Case: Display the mouse coordinates within a div.
document.getElementById('mousemoveDiv').addEventListener('mousemove', handleMouseMove);
function handleMouseMove(event) {
const coordinates = 'X: event.clientX, Y: event.clientY';
document.getElementById('coordinates').textContent = coordinates;
}
8. Context Menu Event
The contextmenu event is triggered when the right mouse button is clicked (usually to open a context menu).
- Use Case: Prevent the default context menu from opening and display a custom message.
document.getElementById('contextMenuDiv').addEventListener('contextmenu', handleContextMenu);
function handleContextMenu(event) {
event.preventDefault();
document.getElementById('contextMessage').textContent = 'Custom context menu action.';
}
9. Wheel Event
The wheel event is triggered when the mouse wheel is scrolled.
- Use Case: Zoom in and out of an image when the mouse wheel is scrolled.
document.getElementById('zoomImage').addEventListener('wheel', handleWheel);
function handleWheel(event) {
event.preventDefault();
const scale = event.deltaY < 0 ? 1.1 : 0.9;
this.style.width = 'this.clientWidth * scalepx';
}
Conclusion
Mouse events are essential for creating interactive web applications. By understanding and utilizing these events, you can enhance the user experience on your website. The examples provided here are a great starting point to see how each event works and how it can be implemented in your projects.
Feel free to download the complete code from the repository and experiment with these events to get a hands-on understanding. Happy coding!