03rd June 2024
Understanding Multiple Inheritance and the Diamond Problem in C++
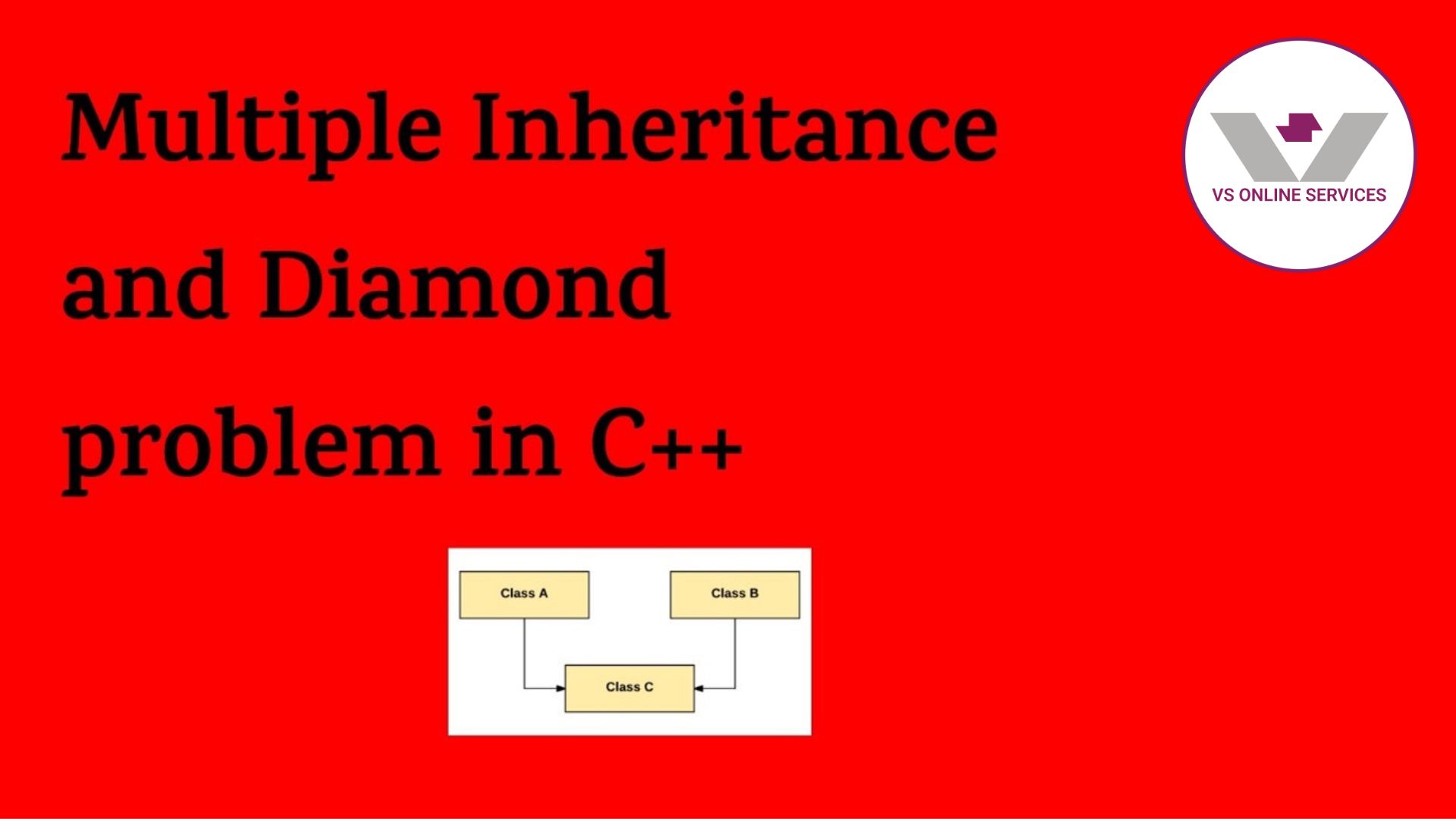
In object-oriented programming, inheritance is a powerful mechanism that allows one class to inherit properties and behaviors from another. This enables code reuse and the creation of more complex and adaptable systems. While single inheritance (where a class inherits from only one base class) is straightforward, multiple inheritance (where a class inherits from more than one base class) introduces additional complexities, including the infamous Diamond Problem.
In this blog, we'll first explore the concept of multiple inheritance and then delve into the Diamond Problem, demonstrating a real-world scenario to illustrate these concepts.
Multiple Inheritance in C++
Multiple inheritance allows a class to inherit features from more than one base class. This can be useful in scenarios where a class needs to combine functionalities from multiple sources.
Consider a scenario where we are developing software for a smart home system. We have separate classes for different functionalities, such as Lighting and Security, and we want to create a SmartHome class that inherits from both to combine their functionalities.
Here’s a simple example to illustrate multiple inheritance:
#include <iostream>
class Lighting {
public:
void turnOnLights() {
std::cout << "Lights turned on" << std::endl;
}
};
class Security {
public:
void activateAlarm() {
std::cout << "Security alarm activated" << std::endl;
}
};
class SmartHome : public Lighting, public Security {
public:
void activateSystems() {
turnOnLights();
activateAlarm();
std::cout << "Smart home systems activated" << std::endl;
}
};
int main() {
SmartHome myHome;
myHome.activateSystems();
return 0;
}
In this example:
- The Lighting class provides functionality to control the lighting.
- The Security class provides functionality to activate the security alarm.
- The SmartHome class inherits from both Lighting and Security, combining their functionalities to create a comprehensive smart home system.
Let’s add functionality to show device status for lights and alarm.
#include <iostream>
class Lighting {
public:
void turnOnLights() {
std::cout << "Lighting is on" << std::endl;
}
void status() {
std::cout << "Lighting status: On" << std::endl;
}
};
class Security {
public:
void activateAlarm() {
std::cout << "Alarm is turned on" << std::endl;
}
void status() {
std::cout << "Security status: Alarm activated" << std::endl;
}
};
class SmartHome : public Lighting, public Security {
public:
void printStatusOfAllDevices() {
status();
}
void activateSystems() {
turnOnLights();
activateAlarm();
// You want to print each device status
}
};
The program encounters an error called ambiguity.
What is Ambiguity?
In C++ multiple inheritance, ambiguity arises when a derived class inherits from more than one base class, and those base classes have members (functions or variables) with the same name. This leads to a situation where it is unclear which member the derived class should use, causing a conflict that the compiler cannot resolve without additional information.
Let's try to resolve it.
The compiler is unclear about which member the derived class should use - Can we specify the member somehow?
By using the scope resolution, we can specify it.
#include <iostream>
class Lighting {
public:
void turnOnLights() {
std::cout << "Lighting is on" << std::endl;
}
void status() {
std::cout << "Lighting status: On" << std::endl;
}
};
class Security {
public:
void activateAlarm() {
std::cout << "Alarm is turned on" << std::endl;
}
void status() {
std::cout << "Security status: Alarm activated" << std::endl;
}
};
class SmartHome : public Lighting, public Security {
public:
void printStatusOfAllDevices() {
Lighting::status();
Security::status();
}
void activateSystems() {
turnOnLights();
activateAlarm();
// You want to print each device status
}
};
Key Note: Ambiguity is not just a matter of the same names; it's about the compiler not being able to determine which member to use because of the multiple inheritance.
The scope resolution operator (::) resolves ambiguity by specifying which base class's member function to use.
while scope resolution helps resolve immediate conflicts, virtual inheritance offers a more structural solution for avoiding ambiguity in complex inheritance hierarchies.
Introducing the Diamond Problem
Now, let's extend our scenario to introduce the Diamond Problem. Suppose we want to add a new feature: a Device class that provides some common functionality (e.g., a method to display the device status). Both Lighting and Security should inherit from Device to access this common functionality.
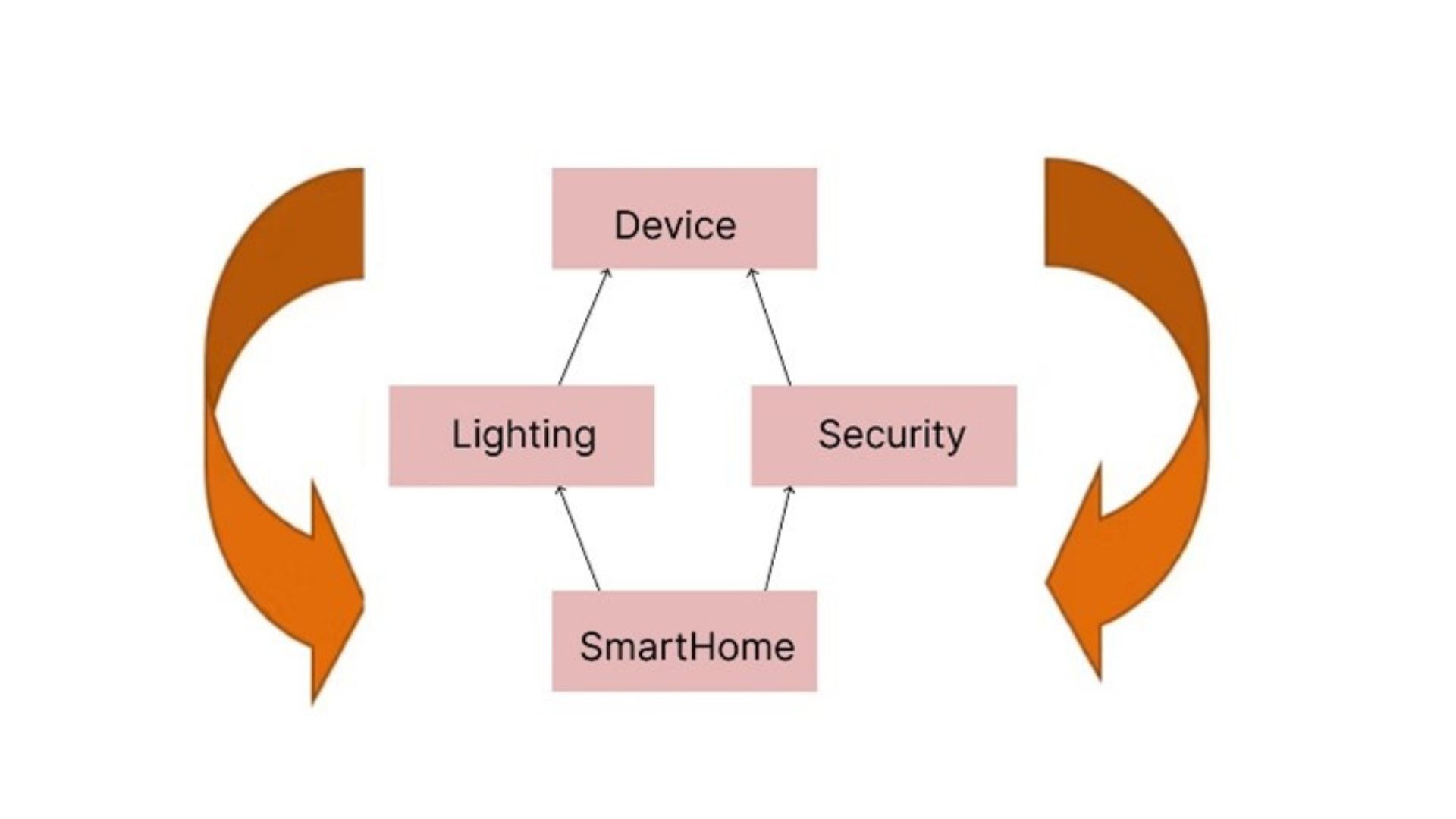
#include <iostream>
class Device {
public:
void status() {
std::cout << "Device Status Printed" << std::endl;
}
};
class Lighting : public Device {
public:
void turnOnLights() {
std::cout << "Lighting is on" << std::endl;
}
};
class Security : public Device {
public:
void activateAlarm() {
std::cout << "Alarm is turned on" << std::endl;
}
};
class SmartHome : public Lighting, public Security {
public:
void activateSystems() {
turnOnLights();
activateAlarm();
status();
}
};
In this code, you face an ambiguity issue because the Device is used by the base classes of SmartHome and SmartHome also uses the Device class.
How to Solve This?
Let’s study what is virtual inheritance.
What is Virtual Inheritance?
Virtual inheritance is a feature in C++ that allows a derived class to inherit from a base class such that only one instance of the base class is shared among all the derived classes. This feature is particularly useful in situations where multiple inheritance leads to the "diamond problem" or ambiguity issues.
#include <iostream>
class Device {
public:
void status() {
std::cout << "Device Status: " << std::endl;
}
};
class Lighting : virtual public Device {
public:
void turnOnLights() {
std::cout << "Lighting is on" << std::endl;
}
};
class Security : virtual public Device {
public:
void activateAlarm() {
std::cout << "Alarm is turned on" << std::endl;
}
};
class SmartHome : public Lighting, public Security {
public:
void activateSystems() {
turnOnLights();
activateAlarm();
status();
}
};
Note: Virtual Inheritance not just for resolving the Diamond Problem but also for scenarios where you want to ensure a single instance of a base class is shared among multiple derived classes.
it's important to note that virtual inheritance should be used judiciously due to its runtime overhead and potential complexity.
Conclusion
In C++, the Diamond Problem arises when a class inherits from two classes that both derive from a common base class, leading to ambiguity. Two common solutions to this problem are using scope resolution and virtual inheritance.
- Scope Resolution: By using the scope resolution operator (::), you can specify which member function or variable from a particular base class to use, effectively resolving ambiguity in multiple inheritance scenarios.
- Virtual Inheritance: Virtual inheritance ensures that only one instance of the base class is shared among all the derived classes. This resolves the Diamond Problem by eliminating ambiguity and allowing the multiple inheritance structure to function correctly.
By understanding and applying these techniques—scope resolution and virtual inheritance—you can effectively manage complex inheritance hierarchies in C++, ensuring clear and predictable behavior in your programs. Happy coding!