27th Jun 2023
Passing data between electron and application(Front End)
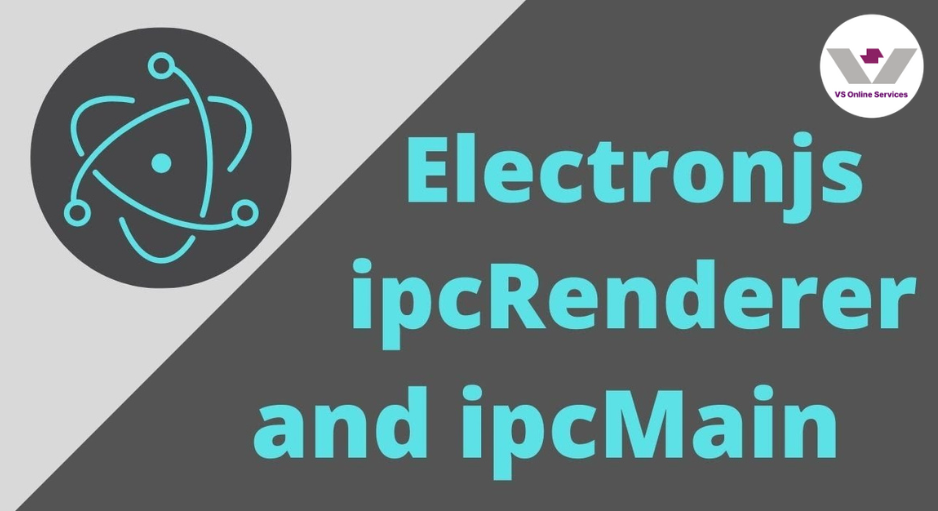
Electron is an open- source frame developed by GitHub that allows you to make cross-platform desktop operations using web technologies similar as HTML, CSS, and JavaScript. It combines the Chromium rendering engine and Node.js runtime, enabling you to create native-like applications for Windows, macOS, and Linux operating systems.
With Electron, you can leverage your existing web development skills to create desktop applications with access to operating system APIs and features. Electron operations are erected using a combination of main and renderer processes. The main process, running in the Node.js environment, manages the application lifecycle and provides native desktop integration capabilities. The renderer process, running in Chromium, handles the rendering of web-based user interfaces.
Why Electron
Cross-platform development: Electron allows developers to build applications that can run on multiple operating systems, including Windows, macOS, and Linux, using a single codebase. This cross-platform compatibility saves time and effort compared to developing separate applications for each platform.
Web technologies: Electron leverages web technologies such as HTML, CSS, and JavaScript, which are widely known and used by developers. This allows web developers to transition their skills and knowledge to desktop application development without having to learn new programming languages or frameworks.
Access to native features Electron provides access to native desktop features and APIs through its main process, allowing inventors to produce operations with native- suchlike capabilities. This includes features like system announcements, train system access, clipboard relations, and more. Lets produce a design to understand this conception.
To create an Electron project, you can follow these steps
First, create a new directory for your Electron project. You can choose any name you like.
Open your command- line interface( CLI) and navigate to the design directory. Run the following command to initialize npm and create a package.json file:
npm init -y
Run the following command to install Electron as a dev dependency in your project
npm install electron --save-dev
Next, create a new file called main.js in your project directory. This train will contain the main process law for your Electron operation.
const { app, BrowserWindow, ipcMain } = require("electron");
const path = require("path");
let win;
const createWindow = () => {
win = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
nodeIntegration: true,
preload: path.join(__dirname, "preload.js"),
},
autoHideMenuBar: true,
});
win.loadFile("index.html");
};
app.whenReady().then(() => {
createWindow();
});
Now produce train called index.html in your design directory. This train will be the main HTML train for your Electron operation which will be the picture train for UI.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="theme-color" content="#000000" />
<meta
name="description"
content="Web site created using create-react-app"/>
<title>Hello from Electron renderer!</title>
</head>
<body>
<h1>Hello from Electron renderer!</h1>
</body>
</html>
Open the package.json file in a text editor and add the following lines under the "scripts" section. Note that our electron file should always be pointed to main here.
{
"name": "my-electron-app",
"version": "1.0.0",
"description": "",
"main": "main.js",
"scripts": {
"start": "electron .",
"test": "echo "Error: no test specified" && exit 1"
},
"author": "",
"license": "ISC",
"devDependencies": {
"electron": "^25.2.0"
}
}
In your command-line interface, navigate to the project directory and run the following command to start your Electron app.
npm start
This will launch your Electron application, and you should see a window displaying "Hello from Electron renderer!".
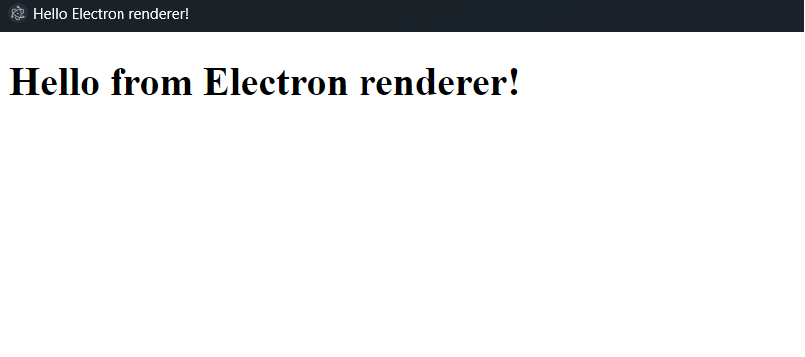
Passing data from Electron to your Window and vice versa
- IpcMain: In Electron, ipcMain is an object handed by the electron module that enables communication between the main process and the renderer processes in your Electron operation. It allows you to send and receive messages, also known as inter-process communication (IPC), between these processes. The main process is responsible for managing the operation's lifecycle, handling system events, and interacting with native APIs. On the other hand, renderer processes handle the rendering and user interface aspects of your application. ipcMain provides methods and events that facilitate the communication between these processes. We also have another important aspect of this communication which is called ipcRenderer which we will see in the next segment.
- IpcRenderer: The ipcRenderer module in Electron is a powerful tool for inter-process communication between the main process and the renderer processes in your Electron application. It allows you to shoot and admit coetaneous and asynchronous dispatches, enabling flawless communication and data exchange between different corridor of your operation.
ipcRenderer and ipcMain are two essential modules in Electron that facilitate inter-process communication (IPC) between the main process and the renderer processes of an Electron application. While they serve different roles, they work together to enable seamless communication between these processes.
Receiving messages
You can use the ipcMain.on( channel, listener) system to hear for dispatches transferred from the renderer processes. The channel parameter specifies the channel name or content of the communication, and the listener is a message function that gets executed when a communication is entered on that channel.
First, we need to register this function in our 'preload.js' file which is linked in our 'main.js' file, this acts as a bridge between the Electron and our front end.
const { contextBridge, ipcRenderer } = require("electron");
contextBridge.exposeInMainWorld("electronAPI", {
sentData: async (data) => ipcRenderer.send("sentData", data),
});
Also we must register our instructors in our'main.js' to admit the data transferred from frontal end.
ipcMain.on("sentData", async (event, data) => {
console.log(data);
});
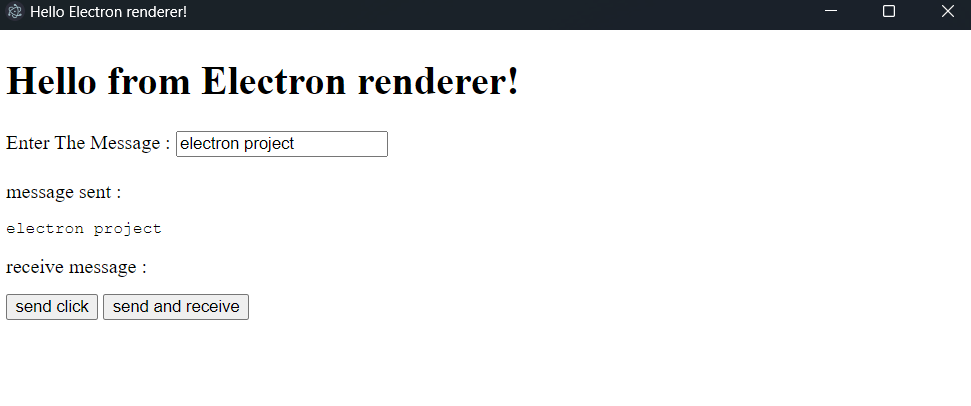
Here first we type the message 'From UI' in the front end and click on 'send message'. By doing this we're entering data from UI to Electron.
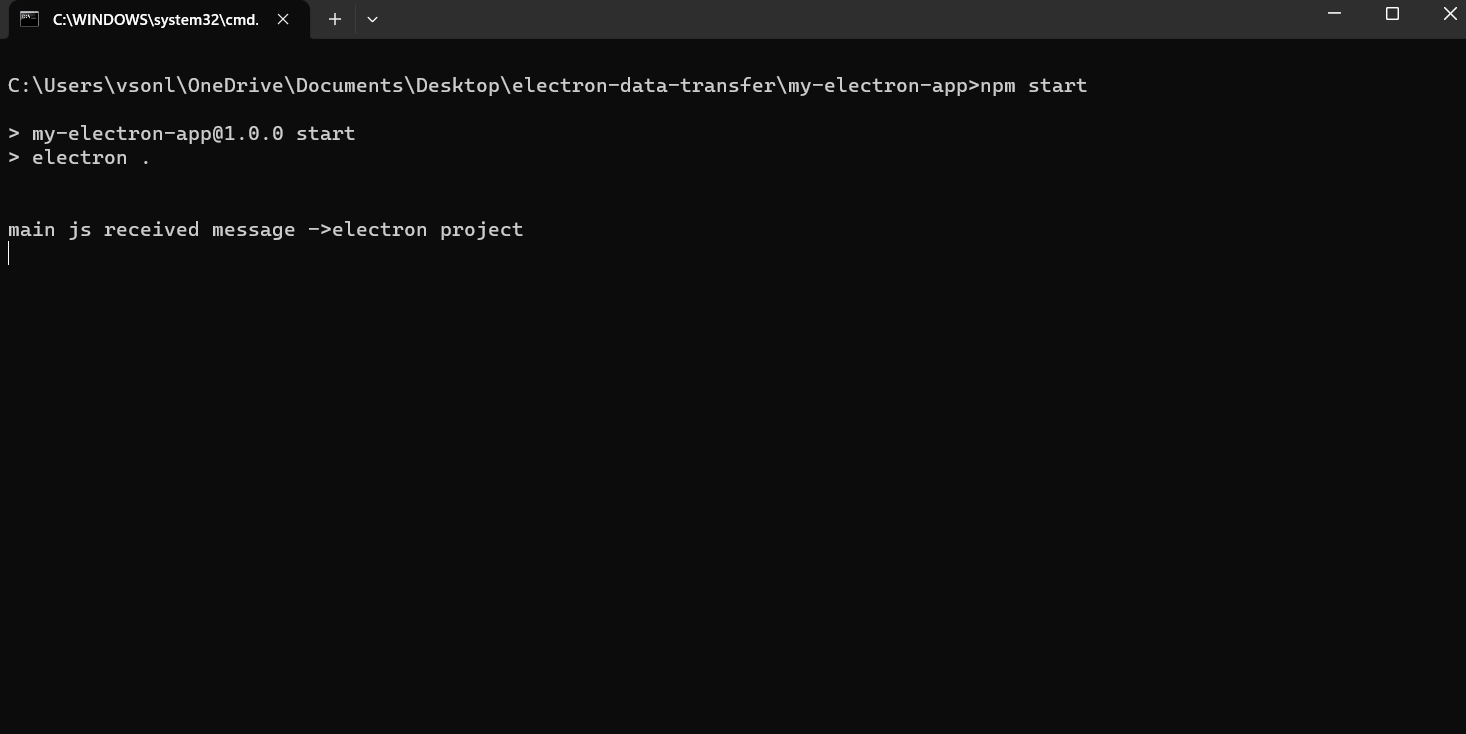
Sending messages
To shoot a communication from the main process to a specific renderer process, you can use the webContents.send( channel,. args) system. The channel parameter specifies the channel name or topic of the message, and ...args represents optional arguments that you want to send along with the message.
Similar to receiving messages, we should also register the method in 'preload.js' which is our bridge file between Electron and UI.
const { contextBridge, ipcRenderer } = require("electron");
contextBridge.exposeInMainWorld("electronAPI", {
sendDataToUI: async (data) => ipcRenderer.once("sendDataToUI", data),
});
function sendDataToUI () {
let data = {
message: "Sending data onload",
};
win.webContents.send("sendDataToUI ", data);
}
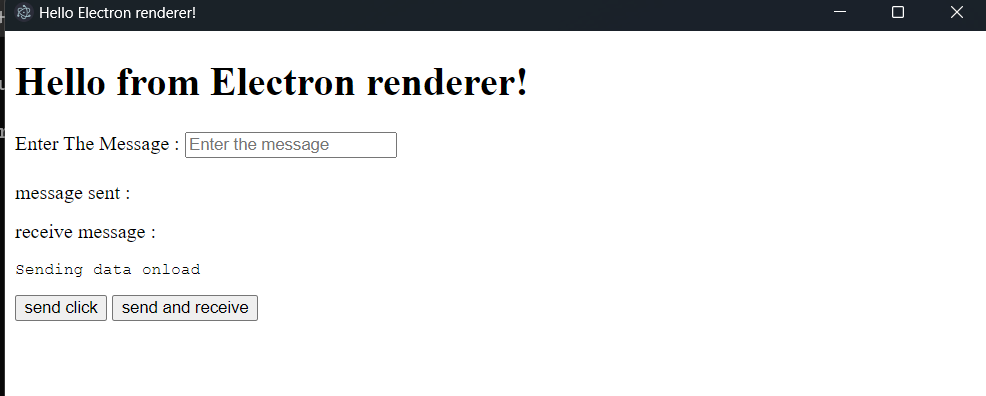
Send data from UI and get back data
Electron, ipcMain.handle is a system handed by the electron module that allows the main process to handle asynchronous dispatches from renderer processes and return a value or a pledge in response. The ipcMain.handle(channel, listener) method sets up a handler for messages sent from the renderer process. It listens for dispatches on the specified channel and executes the listener function when a communication is entered.
First let's register the function in 'preload.js'
const { contextBridge, ipcRenderer } = require("electron");
contextBridge.exposeInMainWorld("electronAPI", {
sendAndReceive: async (data) => ipcRenderer.invoke("sendAndReceive", data),
});
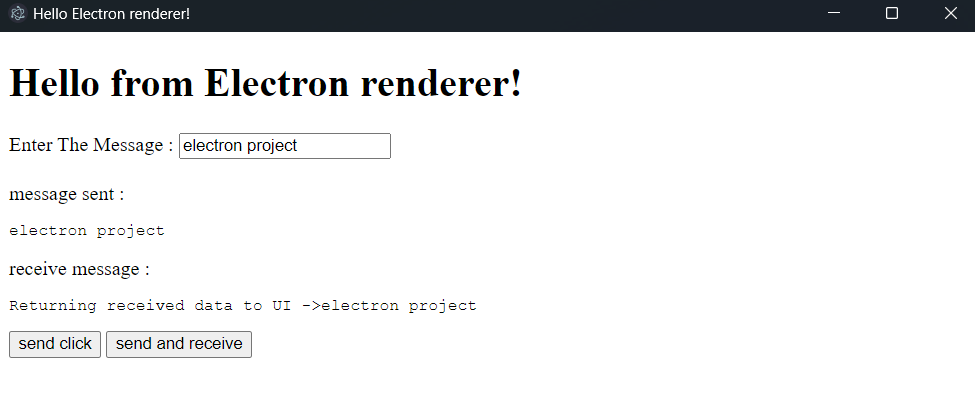
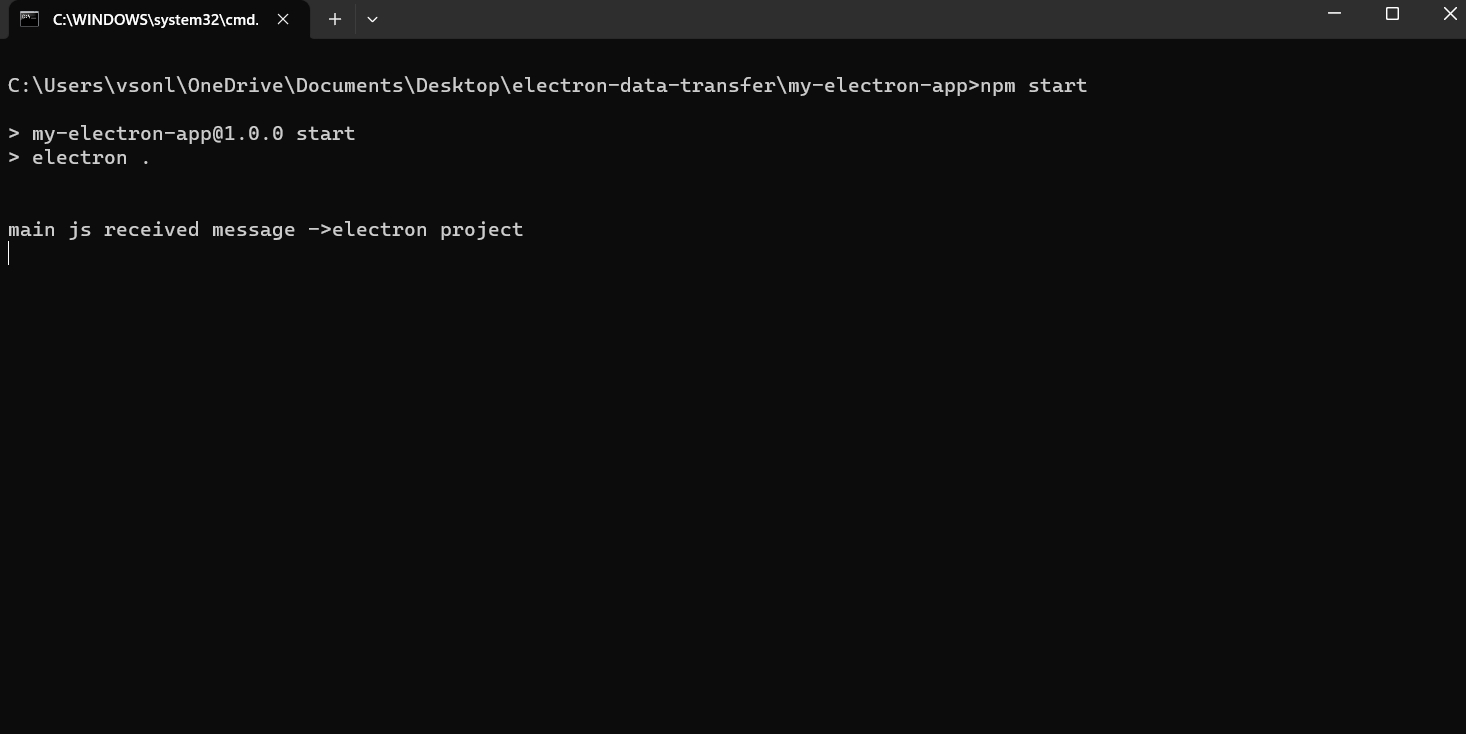
Conclusion
Electron provides a powerful framework for building cross-platform desktop applications using web technologies such as HTML, CSS, and JavaScript. With Electron, you can work your being web development chops to produce native- suchlike desktop operations that can run on Windows, macOS, and Linux operating systems. Electron allows you to build cross-platform desktop applications using web technologies, providing a consistent development experience across different operating systems. The framework combines the Chromium rendering engine and Node.js runtime, enabling you to create desktop applications with access to native features and APIs. Electron applications consist of a main process, responsible for managing the application's lifecycle and interacting with native APIs, and renderer processes, responsible for rendering the user interface.