18th July 2023
Performance Optimization in Python for Multi Dimensional Array
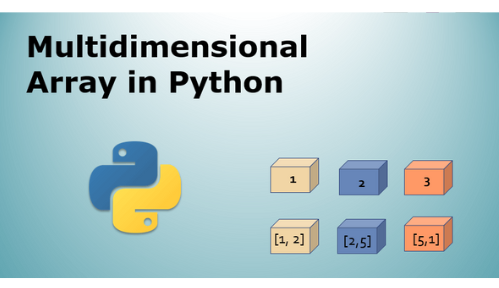
To achieve significant performance improvements when working with multi-dimensional arrays in Python, we embarked on a journey to optimize the processing time of a specific 3D array, aiming to reduce it from 10 seconds to just 1 second.
Initially, we attempted to enhance performance by utilizing Certain functions into Cython instead of the NumPy flatten method, which enabled us to circumvent the use of explicit for loops and element insertion in a multi-dimensional array. However, this approach yielded only a modest improvement, reducing the processing time from 10 seconds to 7 seconds, falling short of our target.
OUR SUCCESSFUL PERFORMANCE OPTIMIZATION STRATEGY
Recognizing the need for a more substantial improvement, we explored an alternative approach. Instead of dealing directly with the 3D array, we first converted the input data into a 1D array. Subsequently, we efficiently inserted elements into this 1D array. Finally, we leveraged NumPy's reshape functionality to transform the 1D array back into the desired 3D structure. This technique proved to be significantly more efficient than declaring a 3D array initially and inserting elements directly into it.
The decision between using "reshape" and "flatten" for multi-dimensional arrays hinges on the specific characteristics of your data and your desired performance outcome. In our case, the choice of "reshape" allowed us to achieve our goal with remarkable efficiency, resulting in a processing time that was not only much faster but also ten times quicker compared to the initial "flatten" approach.
- Reshape: This function is ideal when you need to adjust the shape (dimensions) of the array without altering the underlying data. It creates a new array with the specified shape while retaining the original data. Reshape is valuable when you wish to reorganize your data differently while keeping the data values intact. For instance, you might reshape a 1D array into a 2D array or vice versa without modifying the data values.
- Flatten: In contrast, "flatten" is more appropriate when your goal is to transform a multi-dimensional array into a 1D array by stacking all the elements along a single axis. This operation generates a new 1D array containing all the elements from the original array. Flatten proves useful when you want to work with the data in a linear, 1D format and don't require the original shape.
In summary, the choice between "reshape" and "flatten" hinges on your specific task. Opt for "reshape" when you need to maintain the original shape of the array but wish to change its dimensions while keeping the data intact. On the other hand, select "flatten" when your objective is to convert a multi-dimensional array into a flat, 1D array for further processing, disregarding the original shape. Your choice should align with the specific requirements of your data manipulation task.
STEPS IN CYTHON APPROACH FOR PERFORMANCE
However, if anyone want to try Cython related steps, you can follow the below steps.
Cython involves several steps. Cython is a powerful tool for optimizing Python code by converting it to C code. Here's a general approach for optimizing multi-dimensional arrays using Cython:
- Install Cython: Make sure you have Cython installed. You can install it using pip.
pip install cython
- Write Cython Code: Create a Cython module (usually with a `.pyx` extension) and define the functions that need optimization. In your case, you might want to optimize operations on multi-dimensional arrays. Here's a simple example of a Cython function that calculates the sum of elements in a 2D array.
cython
# mymodule.pyx
cimport numpy as np
import numpy as np
def sum_2d_array(np.ndarray[np.double_t, ndim=2] arr):
cdef int i, j
cdef double total = 0.0
for i in range(arr.shape[0]):
for j in range(arr.shape[1]):
total += arr[i, j]
return total
- Create a Setup File: Create a `setup.py` file to build the Cython extension. Here's an example:
python
from setuptools import setup
from Cython.Build import cythonize
setup(
ext_modules=cythonize("mymodule.pyx"),
)
- Build the Extension: Run the setup script to build the Cython extension.
python setup.py build_ext --inplace
- Use the Cythonized Module: Now, you can import and use the Cythonized module in your Python code.
python
import mymodule
import numpy as np
# Create a 2D NumPy array
arr = np.array([[1.0, 2.0], [3.0, 4.0]])
# Call the Cython function to sum the array
total = mymodule.sum_2d_array(arr)
print(total)
- Compile and Run: Compile and run your Python code. You should notice improved performance compared to the equivalent pure Python code.
Conclusion
Cython allows you to explicitly type variables and use C-like constructs, which can lead to significant performance gains when working with multi-dimensional arrays. Be sure to profile your code and iterate on optimizations as needed to achieve the best performance for your specific use case.