04th June 2024
Understanding Prototypal Inheritance in JavaScript: A Comprehensive Guide
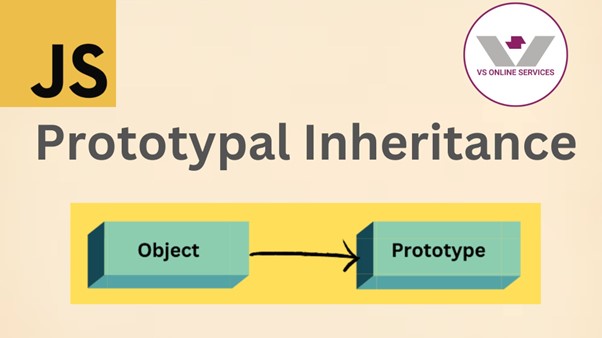
Introduction
JavaScript is a versatile and powerful programming language widely used for web development. One of its unique features is prototypal inheritance, which differs significantly from the classical inheritance model found in languages like Java or C++. Understanding prototypal inheritance is crucial for mastering JavaScript and leveraging its full potential. This article delves into the concept of prototypal inheritance, its workings, and its applications, supplemented with detailed examples and use cases.
What is Prototypal Inheritance?
Prototypal inheritance is a feature in JavaScript where objects inherit properties and methods from other objects. Unlike classical inheritance, which involves creating classes and instantiating objects from them, prototypal inheritance involves linking objects directly.
In JavaScript, every object has an internal link to another object called its prototype. This prototype object can have its own prototype, forming a chain known as the prototype chain. When a property or method is accessed on an object, JavaScript looks for it on the object itself first. If it's not found, JavaScript then searches up the prototype chain until it either finds the property or method or reaches the end of the chain (typically Object.prototype).
The Prototype Chain
The prototype chain is a fundamental concept in prototypal inheritance. It allows for property and method sharing across objects. Here's a simple illustration:
1. Object Creation:
const personPrototype = {
greet: function() {
console.log('Hello, my name is this.name');
}
};
const person = Object.create(personPrototype);
person.name = 'Alice';
person.age = 30;
person.greet(); // Output: Hello, my name is Alice
In this example:
- personPrototype is an object with a greet method.
- person is created using Object.create(personPrototype), which means it inherits from personPrototype.
- When person.greet() is called, JavaScript first looks for the greet method on person. Since it's not found, it looks up the prototype chain to personPrototype and finds it there.
Creating Objects with Prototypal Inheritance
Using Object.create()
The Object.create() method is a straightforward way to create objects with a specific prototype. Here's how it works:
const animalPrototype = {
speak: function() {
console.log('this.name makes a sound.');
}
};
const dog = Object.create(animalPrototype);
dog.name = 'Rover';
dog.breed = 'Golden Retriever';
dog.speak(); // Output: Rover makes a sound.
Using Constructor Functions
Constructor functions can also be used to create objects with prototypal inheritance. This approach is more similar to classical inheritance in other programming languages but still fundamentally based on prototypes.
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log('this.name makes a sound.');
};
const cat = new Animal('Whiskers');
cat.speak(); // Output: Whiskers makes a sound.
Using ES6 Classes
ES6 introduced classes as syntactic sugar over JavaScript's existing prototypal inheritance model. Despite the syntax resembling classical inheritance, it's still prototype-based under the hood.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log('this.name makes a sound.');
}
}
const parrot = new Animal('Polly');
parrot.speak(); // Output: Polly makes a sound.
Extending Prototypes
Prototypal inheritance allows for the easy extension of prototypes, enabling objects to inherit and share behavior.
Example: Extending the Prototype Chain
const personPrototype = {
greet: function() {
console.log('Hello, my name is this.name');
}
};
const employeePrototype = Object.create(personPrototype);
employeePrototype.work = function() {
console.log('this.name is working');
};
const employee = Object.create(employeePrototype);
employee.name = 'Bob';
employee.age = 25;
employee.position = 'Developer';
employee.greet(); // Output: Hello, my name is Bob
employee.work(); // Output: Bob is working
In this example:
- employeePrototype is created with Object.create(personPrototype), making it inherit from personPrototype.
- employee is created with Object.create(employeePrototype), making it inherit from employeePrototype.
- employee can access both greet from personPrototype and work from employeePrototype.
Use Cases of Prototypal Inheritance
1. Shared Methods and Properties
Prototypal inheritance is excellent for sharing methods and properties across multiple objects without duplicating code. For instance, in a game, you might have a Character prototype with shared methods like move, attack, and defend.
2. Dynamic Object Composition
Prototypal inheritance allows for dynamic object composition. You can create objects that inherit from different prototypes at runtime, enabling flexible and reusable code structures.
3. Performance Optimization
Since methods and properties are shared via the prototype chain, memory usage is optimized. Instead of each object having its own copy of methods, they share a single instance, reducing memory overhead.
Advantages of Prototypal Inheritance
- Efficiency: Shared methods and properties reduce memory usage.
- Flexibility: Objects can dynamically inherit from other objects, allowing for more flexible and reusable code.
- Simplicity: Prototypal inheritance is simpler and more straightforward than classical inheritance in many cases.
Conclusion
Prototypal inheritance is a powerful and flexible feature of JavaScript that enables efficient and reusable code. By understanding the prototype chain and how to create and extend objects with prototypes, you can leverage JavaScript's full potential and build robust applications. Whether you use Object.create(), constructor functions, or ES6 classes, mastering prototypal inheritance is essential for any JavaScript developer.