1st May 2024
Angular vs. React: Picking the Perfect Framework for Your 2024 Project
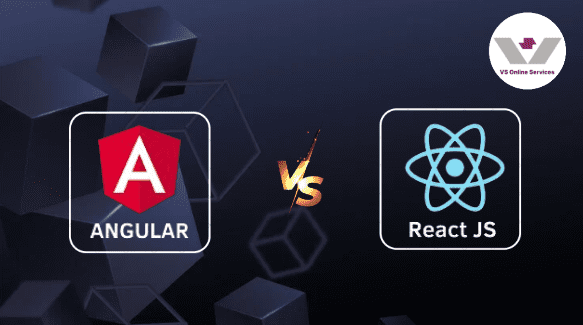
Introduction
The landscape of web development is constantly evolving, with new frameworks emerging and established players refining their offerings. When it comes to building performant and user-friendly single-page applications (SPAs), two frameworks reign supreme: Angular and React. But for developers in 2024, choosing between them can be a daunting task.
This blog post delves into a comprehensive comparison of Angular and React. We'll explore their strengths and weaknesses, examine suitable use cases for each. By the end, you'll be equipped to make an informed decision about which framework best aligns with your project requirements and development preferences.
Overview of Angular and React: Frameworks in the Spotlight
Angular and React are the undisputed heavyweights in the realm of front-end web development. Both empower developers to craft dynamic and engaging single-page applications (SPAs) that provide seamless user experiences. But beneath the surface, they differ significantly in their philosophies and approaches. Let's delve into a core overview of each framework.
Architecture and Data Binding: Unveiling the Core Philosophies
The architectural choices and data binding mechanisms employed by Angular and React significantly influence their development styles and application structures. Let's dissect these fundamental aspects to understand the unique flavours of each framework.
Angular: The Structured Commander (MVC & Two-Way Data Binding)
Architecture:
- Model: Holds the application's data and business logic.
- View: Handles UI presentation using HTML templates.
- Controller: Mediates communication between model and view, managing user interactions and data updates.
Data Binding:
Angular champions two-way data binding. Changes in the view (e.g., form input) automatically reflect in the underlying model, and vice versa. This simplifies development by reducing manual data flow management.
// component.ts
export class MyComponent {
name = 'John Doe';
updateName(newName: string) {
this.name = newName;
}
}
// template.html
<input type="text" [(ngModel)]="name" (keyup)="updateName($event.target.value)">
Benefits of Angular's Architecture and Data Binding:
- Reduced Boilerplate Code: Two-way data binding eliminates the need for manual DOM manipulation.
- Improved Developer Experience: Clear separation of concerns promotes code organization and maintainability.
- Suitable for Large-Scale Projects: Enforced structure provides a solid foundation for complex applications.
Drawbacks of Angular's Architecture and Data Binding:
- Steeper Learning Curve: Beginners might find the MVC structure and terminology less intuitive initially.
- Potential for Performance Overhead: Two-way data binding can introduce unnecessary re-renders in certain scenarios.
React: Flexible and Component-Based (One-Way Data Binding)
- Architecture: React adopts a component-based architecture. Applications are built by composing reusable UI components that encapsulate both presentation and logic. This promotes modularity and easier maintenance.
- Data Binding: React employs one-way data flow. Data is passed down from parent components to child components as props. This explicit data management requires more development effort but offers greater control.
function MyComponent(props) {
const [name, setName] = useState('John Doe');
const handleChange = (event) => {
setName(event.target.value);
}
return (
<div>
<input type="text" value={name} onChange={handleChange} />
</div>
);
}
Benefits of React's Architecture and Data Binding:
- Ease of Learning: The core concepts of components and props are relatively simpler to grasp.
- Flexibility: One-way data flow allows for fine-grained control over data updates.
- Performance Optimization: React's virtual DOM minimizes unnecessary DOM manipulations, potentially leading to smoother performance.
Drawbacks of React's Architecture and Data Binding:
- Increased Boilerplate Code: Manual data flow management can involve more code compared to Angular's two-way binding.
- Potential for Code Complexity: In large projects, maintaining component structure and data flow can become challenging without proper organization.
Componentization and Reusability in Angular vs React
Componentization, the art of breaking down applications into reusable UI building blocks, is a cornerstone of modern front-end development. Both Angular and React champion this approach, but with some philosophical distinctions. Let's delve into how each framework fosters code reusability.
Angular: Structured Reusability with Directives
- Components: Angular applications are built from well-defined components encapsulating UI logic, presentation (HTML templates), and styles (CSS). This promotes code organization and maintainability.
- Reusability: Components are reusable across the application or even in other Angular projects. They can receive data as inputs and emit events to communicate with other components.
- Directives: Angular offers directives, which are reusable markers that manipulate the DOM or add behaviour to components. These can further enhance reusability by providing common functionalities across different components.
// user-card.component.ts
@Component({
selector: 'app-user-card',
templateUrl: './user-card.component.html',
styleUrls: ['./user-card.component.css']
})
export class UserCardComponent {
@Input() name: string; // Receive user name as input
@Output() userSelected = new EventEmitter<string>(); // Emit event on user selection
selectUser() {
this.userSelected.emit(this.name);
}
}
React: Flexible Reusability with Functional Components
- Components: Similar to Angular, React applications are composed of reusable components. These components are pure functions that take inputs (props) and return JSX (a syntax extension for describing UI) to render the UI.
- Reusability: React components are highly reusable across the application or even in other React projects. They are lightweight and promote a modular development style.
- Functional Components: React heavily utilizes functional components, which are simple functions that map props to UI. This encourages reusability by focusing on pure UI logic without complex lifecycle methods.
// UserCard.jsx
function UserCard(props) {
const { name, onSelect } = props; // Destructure props
const handleSelect = () => {
onSelect(name);
}
return (
<div className="user-card">
<p>{name}</p>
<button onClick={handleSelect}>Select</button>
</div>
);
}
Performance and Optimization
Both Angular and React can deliver performant applications with proper optimization strategies. React might have a slight edge for highly dynamic UIs, while Angular can shine in initial load times for complex applications. The choice depends on your specific project requirements and performance priorities.
Angular:
- Strengths: Angular's ahead-of-time compilation and lazy loading can lead to efficient initial page loads. Its built-in change detection mechanism optimizes re-renders.
- Limitations: Two-way data binding can introduce unnecessary re-renders in complex scenarios. Large Angular applications might require more optimization effort.
React:
- Strengths: React's virtual DOM minimizes unnecessary DOM manipulations, potentially leading to smoother performance for highly dynamic UIs.
- Limitations: Managing state and data flow in complex React applications can impact performance if not done efficiently.
Learning Curve, Community Support, and Industry Adoption
Angular has a steeper learning curve due to its comprehensive nature. It enforces structure through TypeScript and uses concepts like dependency injection. However, this structure comes with Angular documentation from Google and a large community to help beginners. Angular is widely adopted in the enterprise world for building complex web applications.
React has a gentler learning curve because it focuses on building reusable UI components. It uses plain JavaScript (or optional JSX syntax) and offers more flexibility in how you structure your application. React's documentation is good, but because it's a library, much of the learning comes from community resources and third-party libraries. While React is strong in the startup world, it's also being increasingly adopted by large enterprises.
Decision Factors and Considerations
Project complexity:
For large, enterprise-grade applications, Angular's structure and built-in features can be advantageous. For smaller projects or those with a focus on UI, React's flexibility might be a better fit.
Team experience:
If your team is familiar with TypeScript and enjoys a structured approach, Angular could be a good choice. If your team prefers JavaScript and more flexibility, React might be the way to go.
Project timeline:
If you need to get started quickly, React's easier learning curve might be beneficial. If you have time to invest in learning a comprehensive framework, Angular can provide a solid foundation for complex applications.
Conclusion
Both Angular and React are excellent choices for front-end development, each with its strengths and weaknesses. Consider the factors above to determine which framework best suits your project needs and team preferences. Remember, there's no single "best" choice; the most important thing is to pick a framework that will help you build a successful and maintainable application.