16th May 2024
Unlocking React's Power: A Guide to Custom Hooks and Their Practical Use
Beyond the Basics: What Are Custom Hooks in React?
Have you ever built a React app and found yourself writing the same logic repeatedly in different components? It can feel repetitive and inefficient. Thankfully, React Hooks, introduced in version 16.8, offer a solution to this problem.
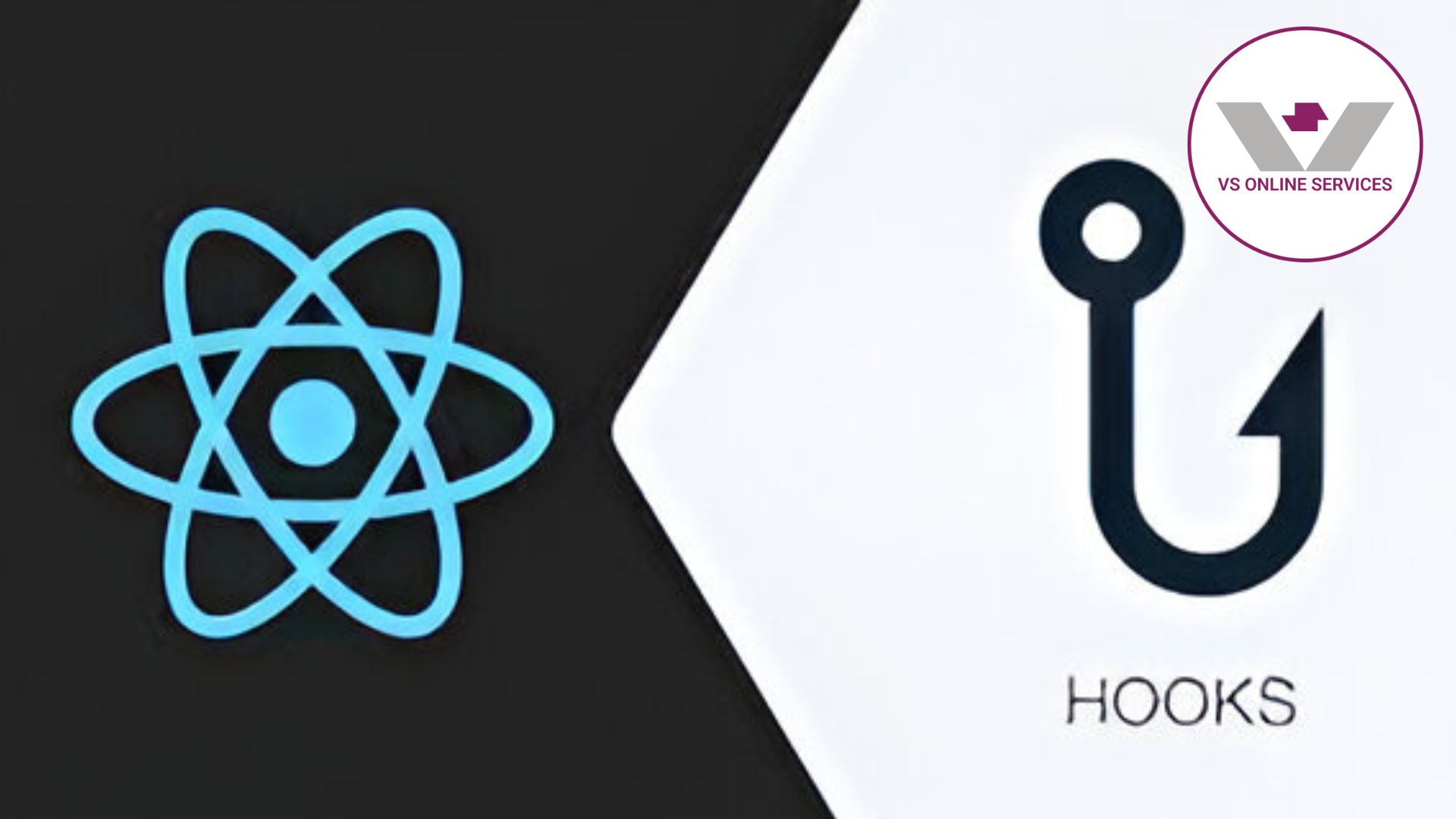
What are React Hooks?
Imagine your React components as delicious dishes. They display information and allow users to interact with your application. But sometimes, these dishes need a little extra something to make them truly shine. That's where React Hooks come in!
Think of React Hooks like spices in your kitchen. They are special functions that you can "add" to your components to give them additional functionality.
There are built-in React Hooks that handle common tasks like managing state (useState) and performing side effects (useEffect). These hooks are like your basic spices - salt, pepper, maybe some garlic. They bring essential flavour to your components.
Custom Hooks: Your Spice Rack Gets Bigger!
But what if you need a more specific flavour? Maybe a custom spice blend for your famous mac and cheese? That's where Custom Hooks come in!
Custom hooks are reusable functions that you create yourself. They allow you to encapsulate complex logic or functionality that you can then use across multiple components in your React app.
Think of it like creating your own unique spice blend. You can combine different built-in hooks, or even other custom hooks you've created, to achieve a specific functionality. This custom blend can then be "sprinkled" on any component that needs that particular flavour.
Why Use Custom Hooks? Your Toolbox Gets Bigger!
Imagine you're building a toolbox for your React development projects. You have the essential tools - screwdrivers, hammers, wrenches - that cover most basic tasks. But what if you encounter a project that requires a specialized tool, like a level for hanging shelves or a pipe wrench for plumbing repairs?
That's where custom hooks come in! They act as your specialized tools in the React development toolbox, extending your capabilities beyond the built-in hooks. Here's why custom hooks are essential for any React developer:
1.Supercharge Reusability:
Have you ever found yourself writing the same functionality for fetching data, handling authentication, or managing user preferences across multiple components? It's a tedious and error-prone process. Custom hooks eliminate this repetition.
By encapsulating the logic into a reusable function, you can use the same custom hook in any component that needs that specific functionality. It's like having a pre-built tool in your toolbox, ready to be used for any project requiring that task. This saves you development time and ensures consistency across your codebase.
2. Enhance Code Readability:
Complex logic can make your components hard to understand and maintain. Custom hooks help by compartmentalizing that complexity. By extracting the logic into a separate function with a clear name, you improve the readability of your components.
Imagine a component that handles complex form validation. Instead of having a long block of code within the component, you can create a custom hook named useFormValidation. This makes the component's purpose and functionality much clearer for anyone reading the code.
3. Simplify Maintenance:
As your React application grows, maintaining the code becomes crucial. Custom hooks make this process significantly easier. If you need to update a specific functionality, you only need to modify the logic within the custom hook. This change then propagates to all components using that hook, saving you time and effort.
Think of it like updating a single tool in your toolbox. Instead of having to adjust the functionality in each component where you use a specific logic, you simply modify the custom hook, which automatically updates all dependent components.
4. Unlock Advanced Functionality:
Built-in React hooks provide a solid foundation, but there might be situations where you need more specialized functionality. Custom hooks allow you to combine built-in hooks with other custom hooks or external libraries to create more complex functionalities tailored to your specific needs.
For example, you might create a custom hook that combines useState and a third-party library to handle user authentication with a backend server. This custom hook would provide a single point of entry for all authentication logic in your app.
By incorporating custom hooks into your React development toolbox, you gain the flexibility to tackle a wider range of challenges and build more robust and maintainable applications.
Building Your First Custom Hook: Step-by-Step
Now that you understand the benefits of custom hooks, we’ll create a simple custom hook that displays the current date and time on the screen.
Here's a step-by-step guide:
1. Setting Up the Custom Hook:
- Create a new React component file (e.g., useCurrentDateTime.js). Remember, custom hooks always start with the word "use" followed by a descriptive name.
- Import the necessary hooks from React: useState and useEffect.
import React, { useState, useEffect } from 'react';
2. Creating the State:
- Use the useState hook to manage the state variable that will hold the current date and time.
const useCurrentDateTime = () => {
const [dateTime, setDateTime] = useState(null);
return { dateTime };
};
3. Updating the State with “useEffect”:
- Use the useEffect hook to update the dateTime state with the current date and time whenever the component using the hook renders.
useEffect(() => {
const updateDateTime = () => {
const now = new Date().toLocaleString();
setDateTime(now);
};
updateDateTime();
// Dependency array: Empty array [] ensures the effect runs only once on mount
// We don't need to re-update dateTime on every render
}, []);
Explanation:
- Inside useEffect, we define a function updateDateTime that gets the current date and time using new Date().toLocaleString().
- We call updateDateTime initially to set the state with the current time.
- The dependency array for useEffect is an empty array []. This tells React to only run the effect once after the component mounts (when the hook is first used). Since we don't want to constantly re-update the time on every render, this is the desired behavior.
4. Using the Custom Hook:
- In another component (e.g., App.js), import the useCurrentDateTime hook.
import React from 'react';
import useCurrentDateTime from './useCurrentDateTime';
- Use the hook within your component's JSX to access the dateTime value and display it.
function App() {
const { dateTime } = useCurrentDateTime();
return (
<div>
<h1>The current date and time is: {dateTime}</h1>
</div>
);
}
export default App;
Explanation:
- We import the useCurrentDateTime hook from the file where we defined it.
- Inside the App component, we use the hook to get the dateTime value as part of a destructuring assignment.
- We then display the dateTime value within our JSX using curly braces for interpolation.
Now, whenever you run your React application, the App component will display the current date and time. This simple example demonstrates the power of custom hooks: they encapsulate logic, improve code readability, and promote reusability.
Experimenting Further:
This is just a basic example. You can try modifying this custom hook to:
- Update the time automatically using setInterval inside useEffect.
- Allow customization of the date and time format by accepting props in the custom hook.
By experimenting with custom hooks, you'll unlock their full potential and become a more efficient React developer.
Level Up Your Development: Common Use Cases for Custom Hooks
Custom hooks are like Swiss Army knives in the React developer's toolbox. They offer a versatile and powerful way to tackle various challenges you might encounter while building complex UIs. Here are some common use cases for custom hooks that will elevate your development skills:
1. Data Fetching:
Imagine building an e-commerce app. You might need to fetch product data from an API in multiple components (product listings, product details, cart).
- Benefits: Create a custom hook (useFetchData) that encapsulates the logic for making API calls, handling errors, and managing loading states. This avoids code duplication and keeps your components clean.
2. User Authentication:
Authentication logic can get intricate, especially when dealing with tokens, user sessions, and access control.
- Benefits: Build a custom hook (useAuth) that handles login, logout functionality, and retrieves user data. This centralizes authentication logic and simplifies its use across components requiring user information or authorization checks.
3. Form Handling:
Complex forms with validation and error handling can become messy.
- Benefits: Create a custom hook (useForm) that manages form state, validation logic, and error messages. This ensures consistent form behaviour across your application and makes handling user input more manageable.
Beyond these examples, the possibilities are endless. You can use custom hooks to manage user preferences, perform local storage operations, handle accessibility features, and much more. The key is to identify repetitive logic or complex functionality that can be encapsulated and reused across your components.
Future-Proof Your App: Tips for Highly Reusable Custom Hooks
Custom hooks are a powerful tool, but like any tool, they need to be used effectively to maximize their benefits. Here are some key tips to ensure your custom hooks are highly reusable and future-proof your React applications:
1.Single Responsibility Principle:
Each custom hook should ideally have one clear purpose. This makes it easier to understand, use, and integrate into different components. Avoid creating "do-it-all" hooks that handle multiple unrelated functionalities. Break down complex logic into smaller, single-purpose hooks if necessary.
2. Clear and Concise Naming:
Choose descriptive and meaningful names for your custom hooks. This helps developers understand what the hook does at a glance. Use the "use" prefix followed by a verb that describes the functionality (e.g., useFetchData, useFormValidation).
3. Provide Clear Documentation:
Write clear and concise documentation for your custom hooks. This documentation should explain:
- The purpose of the hook.
- The parameters it takes and their expected types (if using type checking).
- The return value and its type (if using type checking).
- How to use the hook in a component.
You can use comments within the code or dedicate a separate documentation file for detailed explanations.
4. Test Thoroughly:
Write unit tests for your custom hooks to ensure they behave as expected under different conditions. This helps catch bugs early and prevents regressions in future updates.
5. Stay Updated:
Keep yourself updated with the latest advancements in React and custom hooks best practices. As React evolves, new patterns and approaches may emerge. Learning and adapting will ensure your custom hooks remain efficient and future-proof.